WS: Cross-Platform WebSocket Client
Published on by Flutter News Hub
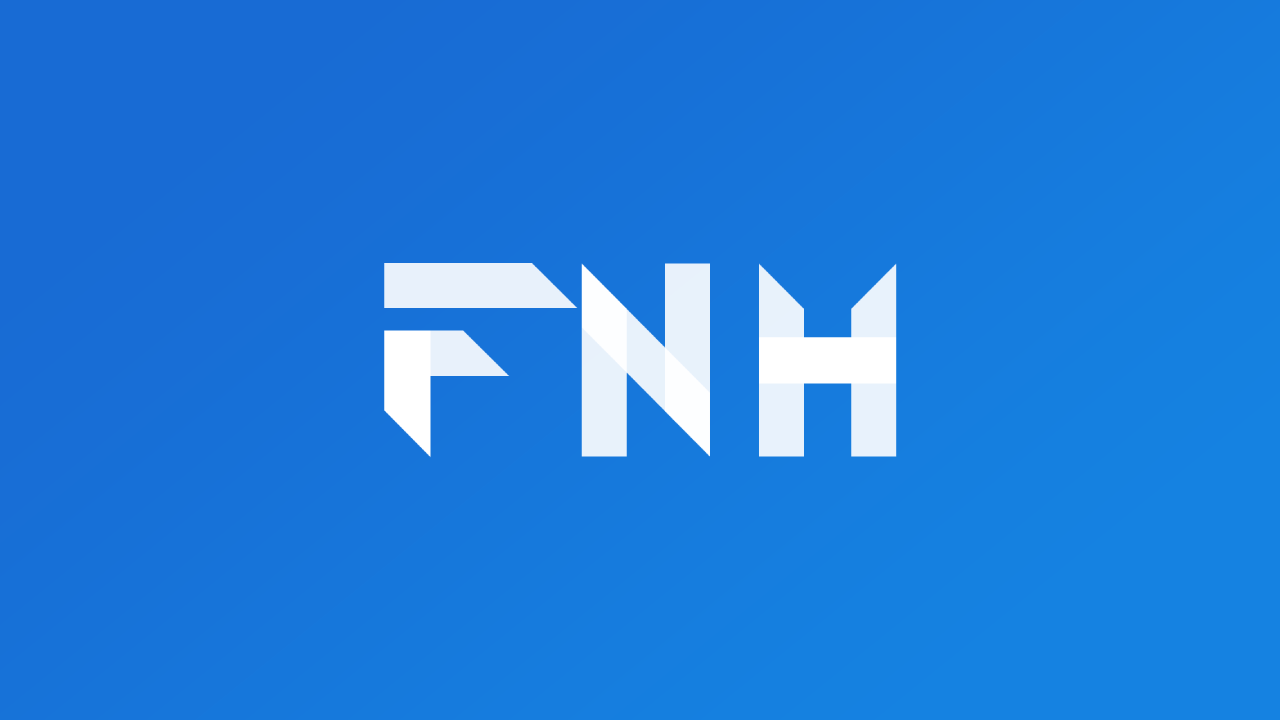
The ws
package provides a comprehensive WebSocket client library for Dart and Flutter applications, enabling seamless communication with WebSocket servers across multiple platforms.
Features
- Cross-platform WebSocket client
- Automatic reconnection with customizable backoff strategy
- JSON message decoding
- WebSocket metrics tracking
- Extensible with interceptors (middlewares)
- Secure WebSocket connections (wss://)
- Customizable connection options for both VM and JS platforms
Installation
Add the following dependency to your pubspec.yaml
file:
dependencies:
ws:
Usage
import 'package:ws/ws.dart';
void main() async {
final ws = WebSocket('ws://echo.websocket.org');
ws.connect().then((_) {
ws.stream.listen((message) {
print('Received message: $message');
});
ws.add('Hello WebSocket!');
});
}
Reconnect with Delay
Implement a simple Exponential Backoff (with Jitter) strategy with full jitter or Fixed Interval
strategy with optional jitter.
import 'package:ws/ws.dart';
void main() async {
// Full Jitter strategy
final ws = WebSocket('ws://echo.websocket.org',
connectionRetryInterval: const WebSocketRetryInterval(
minDelay: Duration(seconds: 1),
maxDelay: Duration(seconds: 10),
),
);
ws.connect().then((_) {
ws.stream.listen((message) {
print('Received message: $message');
});
ws.add('Hello WebSocket!');
});
}
Platform-Specific Options
- VM: Customize the client with
WebSocketOptions.vm()
- JS: Customize the client with
WebSocketOptions.js()
Interceptors (Middlewares)
Add custom pre-processing or post-processing logic to the WebSocket stream.
void main() async {
final ws = WebSocket('ws://echo.websocket.org',
interceptors: [
WsLogInterceptor(logger: print),
WsJsonEncodeInterceptor(),
],
);
ws.connect().then((_) {
ws.stream.listen((message) {
print('Received message: $message');
});
ws.add('Hello WebSocket!');
});
}
Conclusion
The ws
package offers a robust and versatile WebSocket client solution for Dart and Flutter applications. Its comprehensive features, such as reconnection, JSON decoding, metrics, and interceptors, make it an indispensable tool for building real-time communication applications.