Wi-Fi Information Retrieval for Flutter Apps
Published on by Flutter News Hub
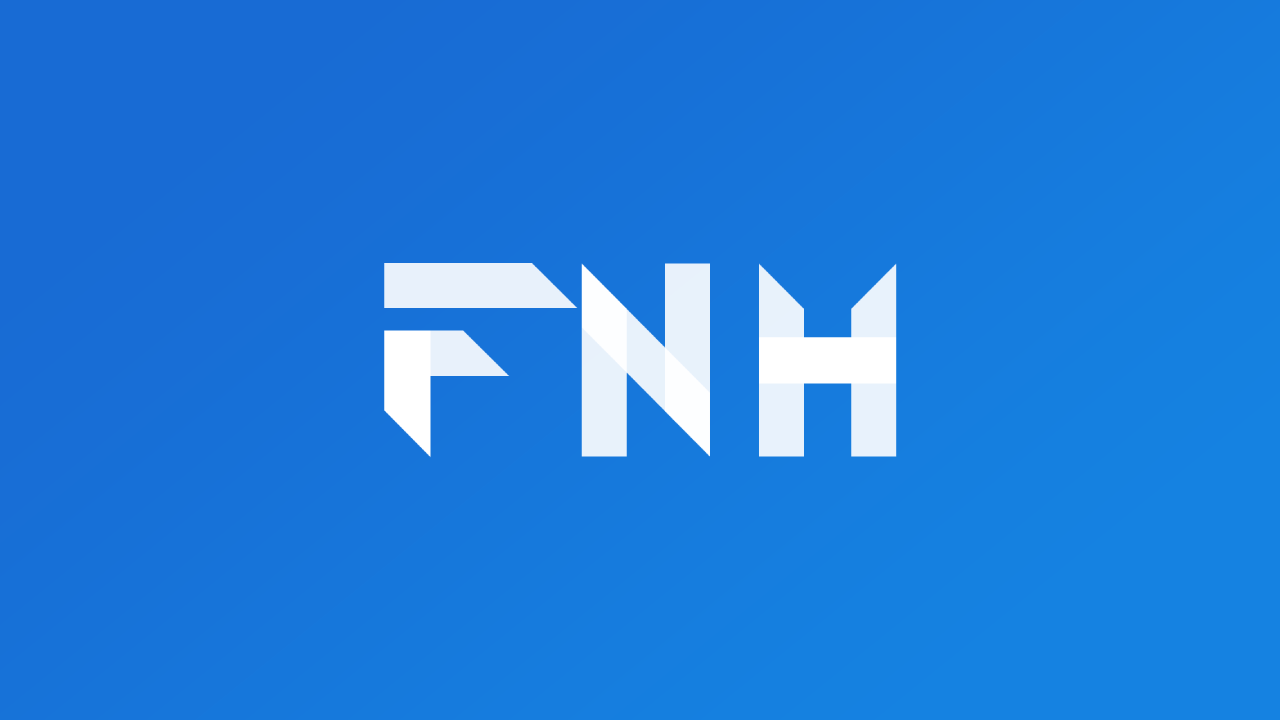
The wifi_info_flutter plugin empowers Flutter developers to retrieve detailed information about a device's Wi-Fi connection. This includes essential details such as the Wi-Fi's BSSID (Basic Service Set Identifier), IP address, and network name (SSID).
Android Integration
For Android devices, the plugin leverages the Android API to access Wi-Fi data. To ensure successful retrieval of Wi-Fi BSSID and IP address, it's crucial to meet the following conditions:
- Ensure your app has the ACCESS_FINE_LOCATION permission. For Android 10 (API level 29) and above, this permission is mandatory.
- Enable location services on the device (Settings > Location).
To retrieve Wi-Fi information on Android, utilize the following code snippets:
import 'package:wifi_info_flutter/wifi_info_flutter.dart';
var wifiBSSID = await WifiInfo().getWifiBSSID();
var wifiIP = await WifiInfo().getWifiIP();
var wifiName = await WifiInfo().getWifiName();
iOS Integration
On iOS devices, the plugin relies on the CNCopyCurrentNetworkInfo function for Wi-Fi information retrieval. However, with iOS 13 and later, Apple has restricted access to this function. Consequently, the plugin will return null values for getWifiBSSID()
and getWifiName()
.
To work around this limitation, apps can request user authorization to use location services. Add the following keys to your Info.plist file, located at <project root>/ios/Runner/Info.plist
:
NSLocationAlwaysAndWhenInUseUsageDescription
Describe why the app needs access to the user’s location information all the time (foreground and background).
NSLocationWhenInUseUsageDescription
Describe why the app needs access to the user’s location information when the app is running in the foreground.
Then, use the requestLocationServiceAuthorization
method to prompt the user for authorization.
Sample Usage
Here's a complete example of using the plugin:
import 'package:flutter/material.dart';
import 'package:wifi_info_flutter/wifi_info_flutter.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State {
String _wifiName = 'Unknown';
String _wifiBSSID = 'Unknown';
String _wifiIP = 'Unknown';
@override
void initState() {
super.initState();
_getWifiInfo();
}
Future _getWifiInfo() async {
var wifiInfo = WifiInfo();
_wifiName = await wifiInfo.getWifiName() ?? 'Unknown';
_wifiBSSID = await wifiInfo.getWifiBSSID() ?? 'Unknown';
_wifiIP = await wifiInfo.getWifiIP() ?? 'Unknown';
setState(() {});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Wi-Fi Information'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('Wi-Fi Name: $_wifiName'),
Text('Wi-Fi BSSID: $_wifiBSSID'),
Text('Wi-Fi IP: $_wifiIP'),
],
),
),
),
);
}
}
Conclusion
The wifi_info_flutter plugin provides a convenient and reliable way to retrieve detailed Wi-Fi information on both Android and iOS devices. By following the outlined steps and code examples, developers can effortlessly integrate Wi-Fi information retrieval into their Flutter applications.