Vexana: A Comprehensive Network Management Solution for Flutter
Published on by Flutter News Hub
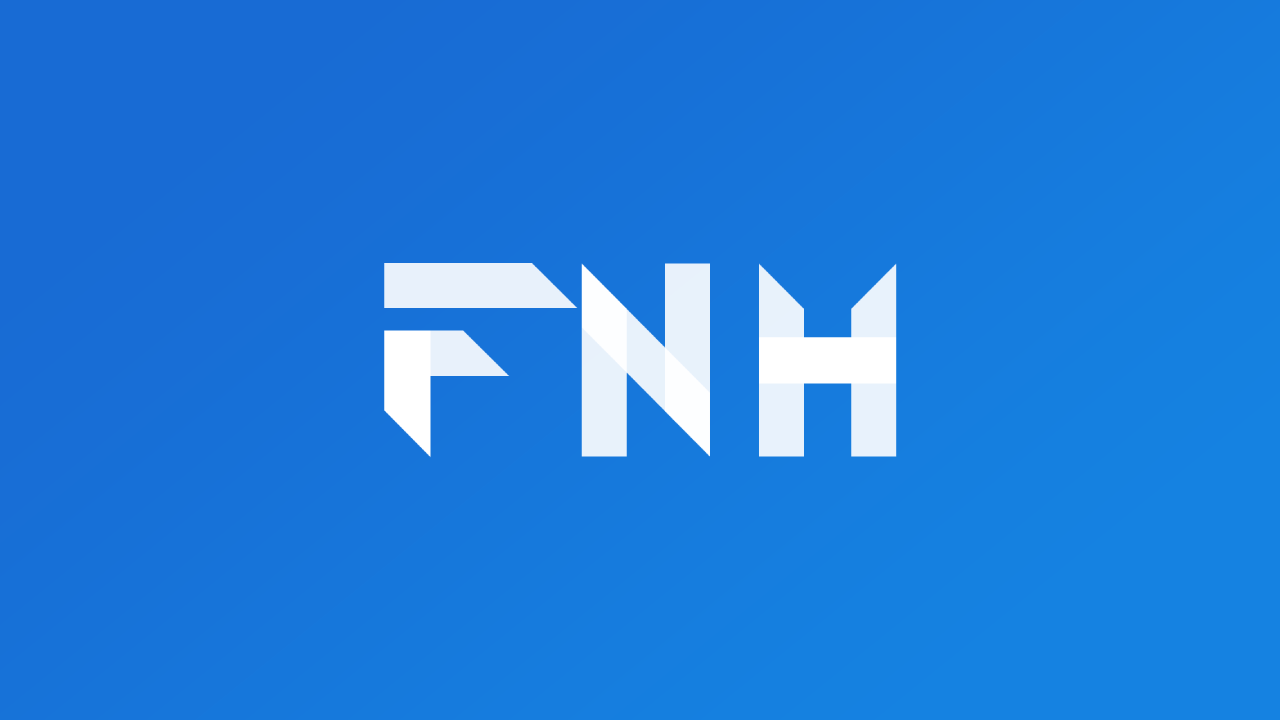
Vexana is a powerful and user-friendly network management library for Flutter applications. It simplifies the process of sending network requests, parsing JSON responses, and handling errors, providing a robust and efficient solution for handling network operations.
Key Features of Vexana:
- Dynamic Model Parsing: Parses JSON responses into custom models with ease.
- Base Error Model: Manages error models effectively, providing a consistent way to handle errors throughout your application.
- Timeout Handling: Sets customizable timeouts to prevent endless network requests.
- Download Files: Seamlessly downloads files in various formats, including PDF, PNG, and more.
- Cancel Requests: Allows you to cancel network requests on demand.
- Primitive Request Handling: Supports parsing of primitive types, such as lists, for simple data retrieval.
- Network Model Interface: Enforces a common interface for network models, ensuring consistency and reducing boilerplate code.
- Refresh Token Support: Provides an elegant solution for refreshing authentication tokens, ensuring uninterrupted network operations.
- Caching: Stores network responses locally for faster access and reduced data usage.
- No Network Handling: Elegantly addresses network connectivity issues, providing a seamless user experience.
Getting Started with Vexana:
To use Vexana, you can follow these steps:
- Add Vexana to your project's dependencies:
dependencies:
vexana: ^1.0.0
- Create a Network Manager:
// Initialize the network manager with custom configurations, if needed.
INetworkManager networkManager = NetworkManager(
isEnableLogger: true,
errorModel: UserErrorModel(), // Custom error model for error handling
options: BaseOptions(
baseUrl: "https://jsonplaceholder.typicode.com",
),
);
- Send a Network Request:
// Send a POST request to the `/todosPost` endpoint, using a custom request body model
final todoPostRequestBody = TodoPostRequestData();
final response = await networkManager.send(
"/todosPost",
parseModel: Todo(),
method: RequestType.POST,
data: todoPostRequestBody,
);
Using Vexana's Utility Features:
Vexana also provides several utility functions for common network operations:
- Download a File:
// Download a file using the file path and a progress callback
final response = await networkManager.downloadFileSimple(
'http://www.africau.edu/images/default/sample.pdf',
(count, total) {
print('$');
},
);
- Cancel a Network Request:
// Initialize a cancel token
final cancelToken = CancelToken();
networkManager
.send('/users?delay=5',
parseModel: ReqResModel(),
method: RequestType.GET,
canceltoken: cancelToken)
.catchError((err) {
// Handle errors specifically related to cancel tokens
if (CancelToken.isCancel(err)) {
print('Request canceled! ' + err.message);
}
});
// Cancel the request after 8 seconds
cancelToken.cancel('canceled');
- Handle Caching:
// Send a GET request with a cache expiration of 3 seconds
final response = await networkManager.send(
"/todos",
parseModel: Todo(),
expiration: Duration(seconds: 3),
method: RequestType.GET,
);
- Handle Refresh Tokens:
// Initialize the network manager with a refresh token callback
INetworkManager networkManager = NetworkManager(
isEnableLogger: true,
options: BaseOptions(
baseUrl: 'https://jsonplaceholder.typicode.com',
),
onRefreshFail: () {
// Navigate to login or take appropriate action when refresh fails
},
onRefreshToken: (error, newService) async {
// Write your refresh token business logic here
// Update the error.req.headers with the new token
return error;
},
);
Conclusion:
Vexana is a comprehensive and versatile network management library for Flutter applications. It empowers developers with a range of features and utilities to streamline and enhance network operations. By leveraging Vexana, you can improve the performance, reliability, and user experience of your Flutter apps.