Unlocking File System Access with path_provider: A Comprehensive Guide for Flutter Developers
Published on by Flutter News Hub
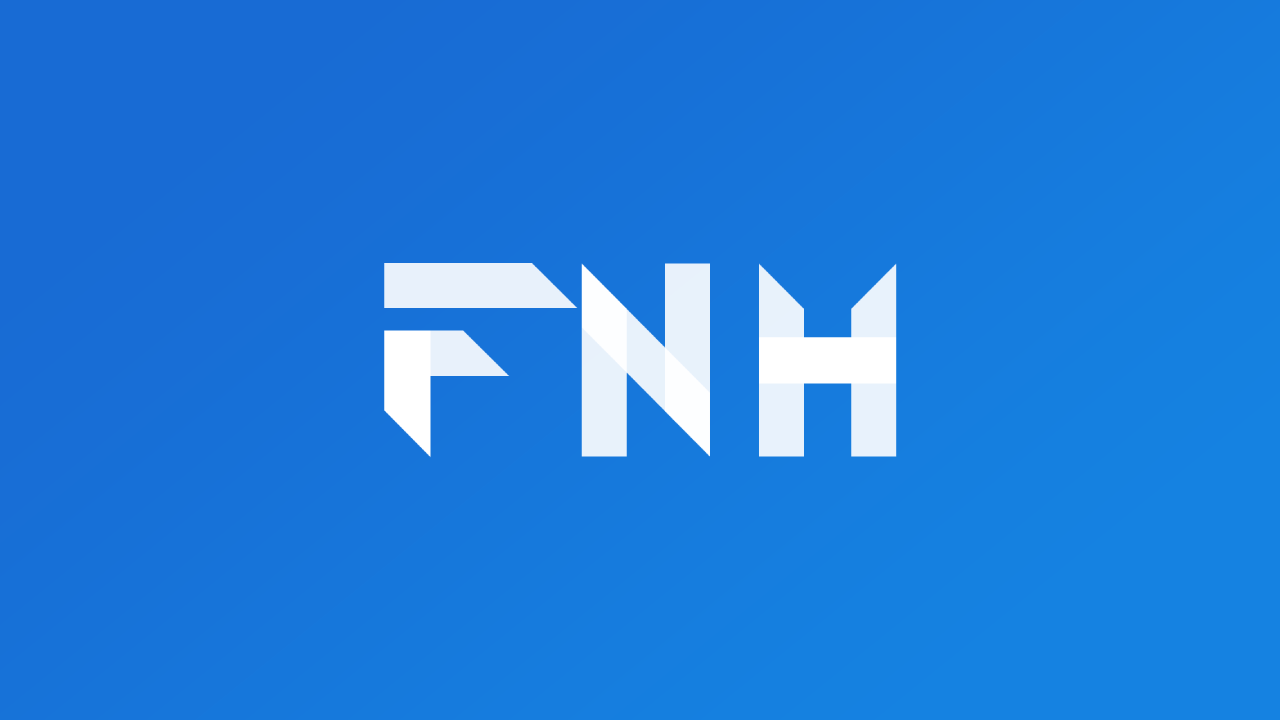
Flutter's path_provider plugin empowers developers with the ability to locate commonly used directories on a device's file system. Whether you need access to temporary storage, application documents, or the downloads folder, this plugin has you covered. It supports a wide range of platforms, including Android, iOS, Linux, macOS, and Windows.
Supported Directories and Platforms
The following table lists the directories supported by path_provider, along with the platforms they're available on:
Directory Android iOS Linux macOS Windows
| Temporary | ✔️ | ✔️ | ✔️ | ✔️ | ✔️
| Application Support | ✔️ | ✔️ | ✔️ | ✔️ | ✔️
| Application Library | ❌️ | ✔️ | ❌️ | ✔️ | ❌️
| Application Documents | ✔️ | ✔️ | ✔️ | ✔️ | ✔️
| Application Cache | ✔️ | ✔️ | ✔️ | ✔️ | ✔️
| External Storage | ✔️ | ❌ | ❌ | ❌️ | ❌️
| External Cache Directories | ✔️ | ❌ | ❌ | ❌️ | ❌️
| External Storage Directories | ✔️ | ❌ | ❌ | ❌️ | ❌️
| Downloads | ❌ | ✔️ | ✔️ | ✔️ | ✔️
Usage
To get started with path_provider, add it as a dependency to your pubspec.yaml file:
dependencies: path_provider: ^2.0.0
Then, you can use the following methods to access specific directories:
import 'package:path_provider/path_provider.dart'; Future getTemporaryDirectory() async { return await getTemporaryDirectory(); } Future getApplicationDocumentsDirectory() async { return await getApplicationDocumentsDirectory(); } Future getDownloadsDirectory() async { return await getDownloadsDirectory(); }
Example
Here's an example of how to use path_provider to save a file to the application documents directory:
import 'dart:io'; import 'package:path_provider/path_provider.dart'; Future saveFile() async { // Get the application documents directory Directory appDocumentsDir = await getApplicationDocumentsDirectory(); // Create a new file in the documents directory File file = File('${appDocumentsDir.path}/my_file.txt'); // Write some data to the file file.writeAsString('Hello, world!'); }
Testing
path_provider uses a PlatformInterface, which means that not all platforms share the same implementation. To test path_provider, mock the PathProviderPlatform rather than the PlatformChannel.
Refer to the path_provider test for an example: https://github.com/flutter/packages/blob/main/packages/path_provider/path_provider/test/path_provider_test.dart
Conclusion
path_provider is an essential plugin for Flutter developers who need to access and manipulate files on a device's file system. With its comprehensive support for various directories and platforms, it empowers you to build robust and user-friendly applications.