Unlock Powerful Autocomplete with Flutter TypeAhead
Published on by Flutter News Hub
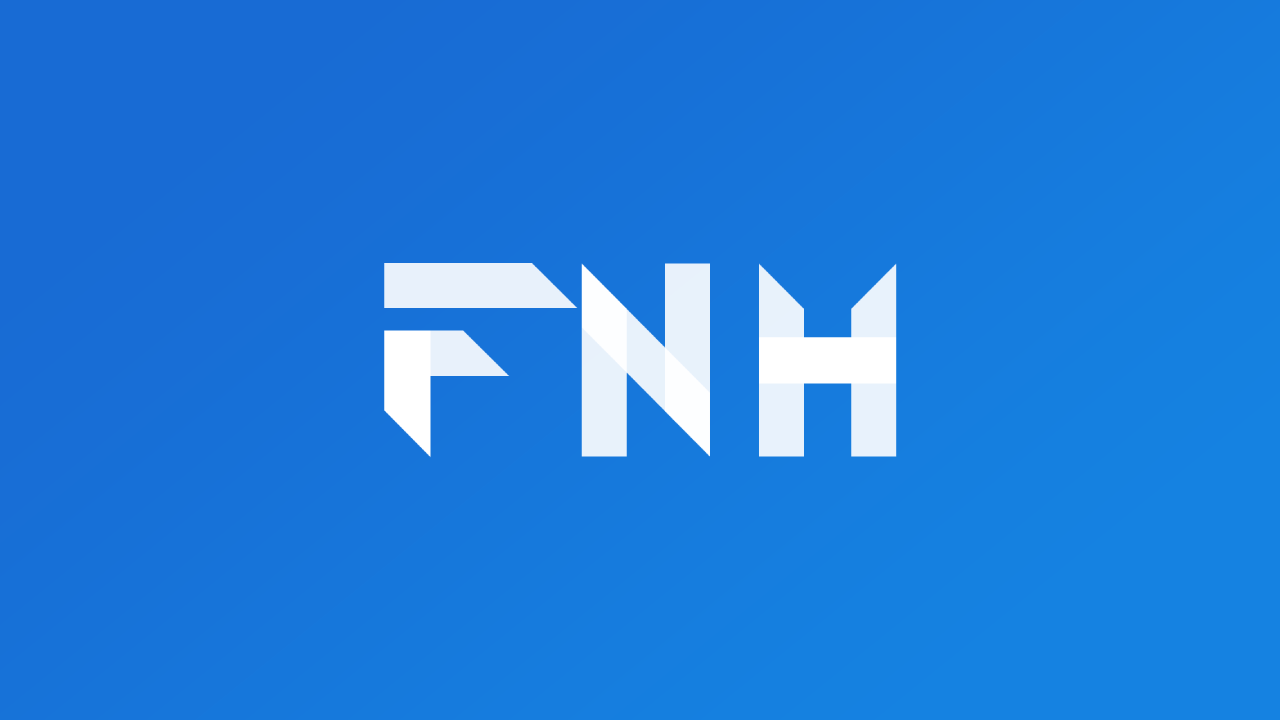
Flutter TypeAhead is a robust widget for creating autocomplete suggestions as users type. It simplifies the process of showing suggestions to users, making it an essential tool for any developer looking to enhance their app's user experience.
Key Features:
- Floating suggestion box that aligns with the width of your TextField
- Customizable layout, animations, decorations, and more
- Available in both Material and Cupertino flavors
Getting Started:
import 'package:flutter_typeahead/flutter_typeahead.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Flutter TypeAhead'), ), body: Center( child: TypeAheadField( suggestionsCallback: (search) => CityService.of(context).find(search), builder: (context, controller, focusNode) => TextField( controller: controller, focusNode: focusNode, decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'City', ), ), itemBuilder: (context, city) => ListTile( title: Text(city.name), subtitle: Text(city.country), ), onSelected: (city) { // Perform action on city selection }, ), ), ), ); } }
Customizing the Layout:
TypeAheadField( listBuilder: (context, children) => GridView.count( crossAxisCount: 2, crossAxisSpacing: 8, mainAxisSpacing: 8, shrinkWrap: true, children: children, ), );
Customizing the TextField:
TypeAheadField( builder: (context, controller, focusNode) => TextFormField( controller: controller, focusNode: focusNode, obscureText: true, decoration: InputDecoration( labelText: 'Password', ), ), );
Customizing the Suggestions Box:
TypeAheadField( decorationBuilder: (context, child) => Material( elevation: 4, borderRadius: BorderRadius.circular(8), child: child, ), offset: Offset(0, 12), constraints: BoxConstraints( maxHeight: 500, ), );
Controlling the Suggestions Box:
- hideOnLoading: Hide the suggestions box during loading.
- hideOnEmpty: Hide the suggestions box when no suggestions are available.
- hideOnError: Hide the suggestions box when an error occurs.
- hideOnSelect: Hide the suggestions box after a suggestion is selected.
- hideOnUnfocus: Hide the suggestions box when the TextField loses focus.
- hideWithKeyboard: Hide the suggestions box when the keyboard is closed.
Controlling the Focus:
- showOnFocus: Show the suggestions box when the TextField gains focus.
- hideOnUnfocus: Hide the suggestions box when the TextField loses focus.
Conclusion:
Flutter TypeAhead is a versatile and powerful autocomplete widget that enhances the user experience in any Flutter app. With its customizable features and easy configuration, it's the perfect tool for any developer seeking to add seamless autocomplete functionality to their applications.