Universal BLE: A Comprehensive Cross-Platform Solution for Bluetooth Low Energy Apps
Published on by Flutter News Hub
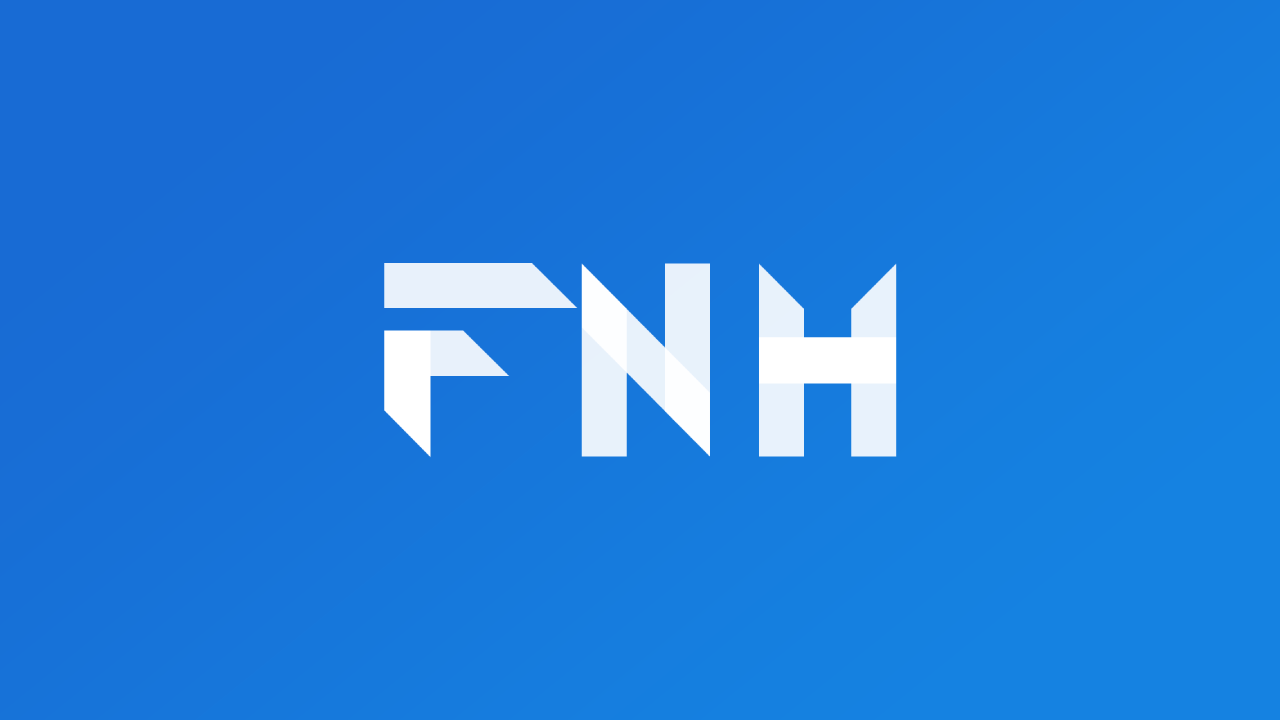
Universal BLE is a powerful plugin that allows Flutter developers to seamlessly interact with Bluetooth Low Energy (BLE) devices across multiple platforms, including Android, iOS, macOS, Windows, Linux, and even the web.
Getting Started
To integrate Universal BLE into your Flutter app:
- Add the following dependency to your pubspec.yaml file:
dependencies:
universal_ble: ^latest
- Import the Universal BLE package in your Dart code:
import 'package:universal_ble/universal_ble.dart';
Scanning for Devices
To scan for BLE devices, you can use the startScan
method:
UniversalBle.startScan();
You can optionally provide a ScanFilter
object to filter the scan results based on services or manufacturer data:
UniversalBle.startScan(
scanFilter: ScanFilter(
withServices: ["SERVICE_UUID"],
withManufacturerData: ["MANUFACTURER_DATA"]
)
);
Once a scan result is discovered, the UniversalBle.onScanResult
event will be triggered, providing you with the device's deviceId
.
Connecting to Devices
To establish a connection with a discovered device, use the connect
method:
UniversalBle.connect(deviceId);
You can receive updates on the connection status through the UniversalBle.onConnectionChanged
event:
UniversalBle.onConnectionChanged = (deviceId, state) {
// Handle connection state changes (e.g. connected, disconnected)
};
Discovering Services and Characteristics
After connecting to a device, you can discover its services and characteristics using the discoverServices
method:
UniversalBle.discoverServices(deviceId);
Reading and Writing Data
Once services and characteristics have been discovered, you can read or write data to them:
// Read data from a characteristic
UniversalBle.readValue(deviceId, serviceId, characteristicId);
// Write data to a characteristic
UniversalBle.writeValue(deviceId, serviceId, characteristicId, value);
// Subscribe to a characteristic for notifications
UniversalBle.setNotifiable(deviceId, serviceId, characteristicId, BleInputProperty.notification);
// Handle characteristic updates
UniversalBle.onValueChanged = (deviceId, characteristicId, value) {
// Process incoming data
};
Pairing and Unpairing Devices
On supporting platforms, Universal BLE allows you to pair and unpair devices programmatically:
// Pair a device
UniversalBle.pair(deviceId);
// Unpair a device
UniversalBle.unPair(deviceId);
You can monitor pairing state changes through the UniversalBle.onPairingStateChange
event.
Bluetooth Availability and Management
Universal BLE provides methods to check and manage Bluetooth availability:
// Get Bluetooth availability state
AvailabilityState state = await UniversalBle.getBluetoothAvailabilityState();
// Enable Bluetooth
UniversalBle.enableBluetooth();
You can also subscribe to UniversalBle.onAvailabilityChange
to receive updates on Bluetooth availability.
Platform-Specific Considerations
- Android: Permissions for Bluetooth scanning and connection must be declared in the Android manifest.
-
iOS/macOS:
NSBluetoothPeripheralUsageDescription
andNSBluetoothAlwaysUsageDescription
must be added to the app's Info.plist. - Windows/Linux: Bluetooth 4.0 is required for support.
-
Web:
withServices
parameter in the ScanFilter must be set to ensure access to specified services on connected devices.
Command Queue and Timeout
Universal BLE uses a command queue to prevent multiple commands from being sent simultaneously. You can disable the queue or set a timeout value using UniversalBle.queuesCommands
and UniversalBle.timeout
respectively.
Customizing Platform Implementation
If necessary, you can create your own platform-specific implementation of Universal BLE by extending the UniversalBlePlatform
base class and setting it as the active instance:
class UniversalBleMock extends UniversalBlePlatform {
// Implement custom commands
}
UniversalBle.setInstance(UniversalBleMock());
Universal BLE simplifies Bluetooth LE development across platforms, empowering you to create powerful and connected apps.