Uni Links: Simplifying App and Web Linking in Flutter
Published on by Flutter News Hub
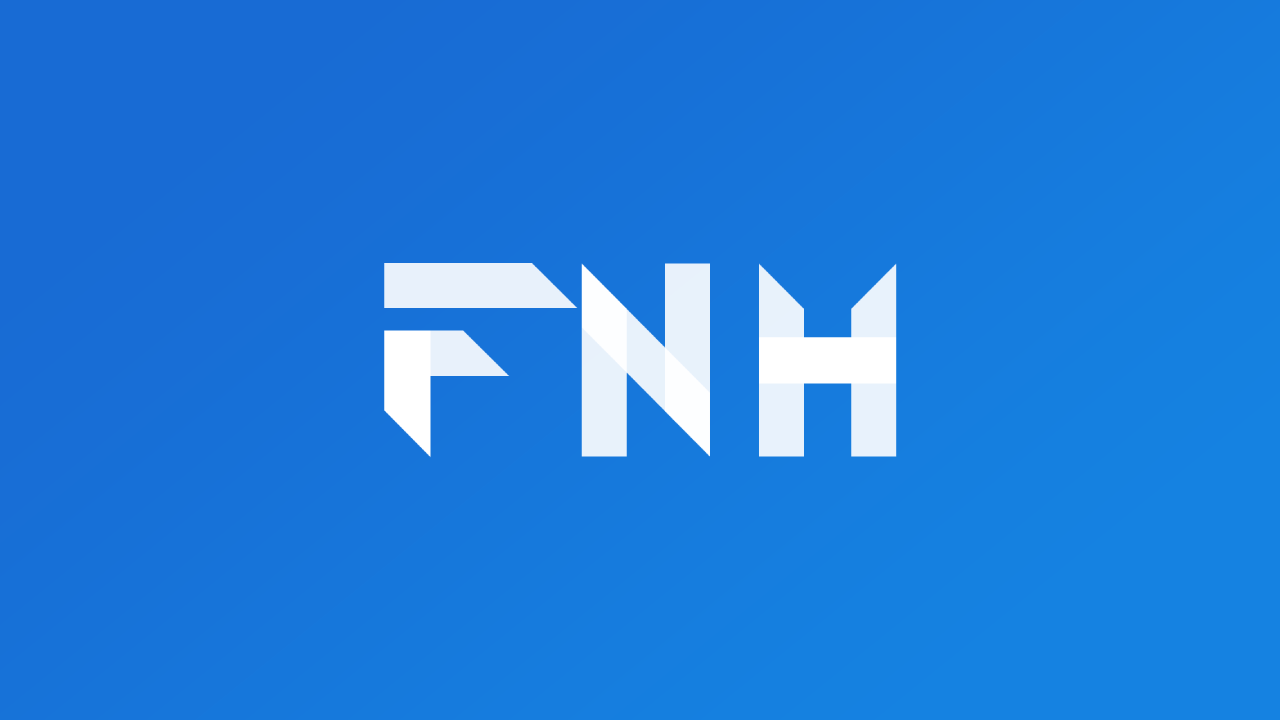
Uni Links is a Flutter plugin that enables seamless app linking and deep linking for both Android and iOS platforms. This powerful plugin empowers developers to handle app startup and URL changes, making it an essential tool for enhancing user engagement and creating a smooth user experience.
Installation and Setup
To integrate Uni Links into your Flutter project, update your pubspec.yaml file by adding:
dependencies: uni_links: ^0.6.0
Next, add the following permission to your Android manifest (if you haven't already):
<manifest ...> <!-- ... other tags --> <application ...> <activity ...> <!-- ... other tags --> <!-- Deep Links --> <intent-filter> <action android:name="android.intent.action.VIEW" /> <category android:name="android.intent.category.DEFAULT" /> <category android:name="android.intent.category.BROWSABLE" /> <!-- Accepts URIs that begin with YOUR_SCHEME://YOUR_HOST --> <data android:scheme="[YOUR_SCHEME]" android:host="[YOUR_HOST]" /> </intent-filter> <!-- App Links --> <intent-filter android:autoVerify="true"> <action android:name="android.intent.action.VIEW" /> <category android:name="android.intent.category.DEFAULT" /> <category android:name="android.intent.category.BROWSABLE" /> <!-- Accepts URIs that begin with https://YOUR_HOST --> <data android:scheme="https" android:host="[YOUR_HOST]" /> </intent-filter> </activity> </application> </manifest>
For iOS, create an associated domain entitlement by adding or editing the com.apple.developer.associated-domains entitlement in your ios/Runner/Info.plist file or through Xcode.
<?xml ...> <!-- ... other tags --> <plist> <dict> <!-- ... other tags --> <key>CFBundleURLTypes</key> <array> <dict> <key>CFBundleTypeRole</key> <string>Editor</string> <key>CFBundleURLName</key> <string>[ANY_URL_NAME]</string> <key>CFBundleURLSchemes</key> <array> <string>[YOUR_SCHEME]</string> </array> </dict> </array> <!-- ... other tags --> </dict> </plist>
Usage: Initial Link and URI
To access the link that started your app, use getInitialLink():
import 'package:uni_links/uni_links.dart'; Future initUniLinks() async { final initialLink = await getInitialLink(); // handle the link... }
You can also convert the initial link to a Uri object using getInitialUri().
To listen for changes in the link, use the linkStream or uriLinkStream:
import 'package:uni_links/uni_links.dart'; StreamSubscription _sub; Future initUniLinks() async { _sub = linkStream.listen((String? link) { // handle the link... }); } @override void dispose() { _sub.cancel(); super.dispose(); }
Handling Cold Starts vs Background Starts
Uni Links distinguishes between cold starts (when the app is launched from a closed state) and background starts (when the app is brought to the foreground from the background). Use getInitialLink() and linkStream to handle cold starts, while getInitialUri() and uriLinkStream are suitable for background starts.
Tools for Invoking Links
For testing purposes, you can use command-line tools to invoke links:
Android:
adb shell 'am start -W -a android.intent.action.VIEW -c android.intent.category.BROWSABLE -d "unilinks://example.com/path/portion/?uid=123&token=abc"'
iOS:
/usr/bin/xcrun simctl openurl booted "unilinks://example.com/path/portion/?uid=123&token=abc"