Transparent Image: A Simple Yet Effective Library
Published on by Flutter News Hub
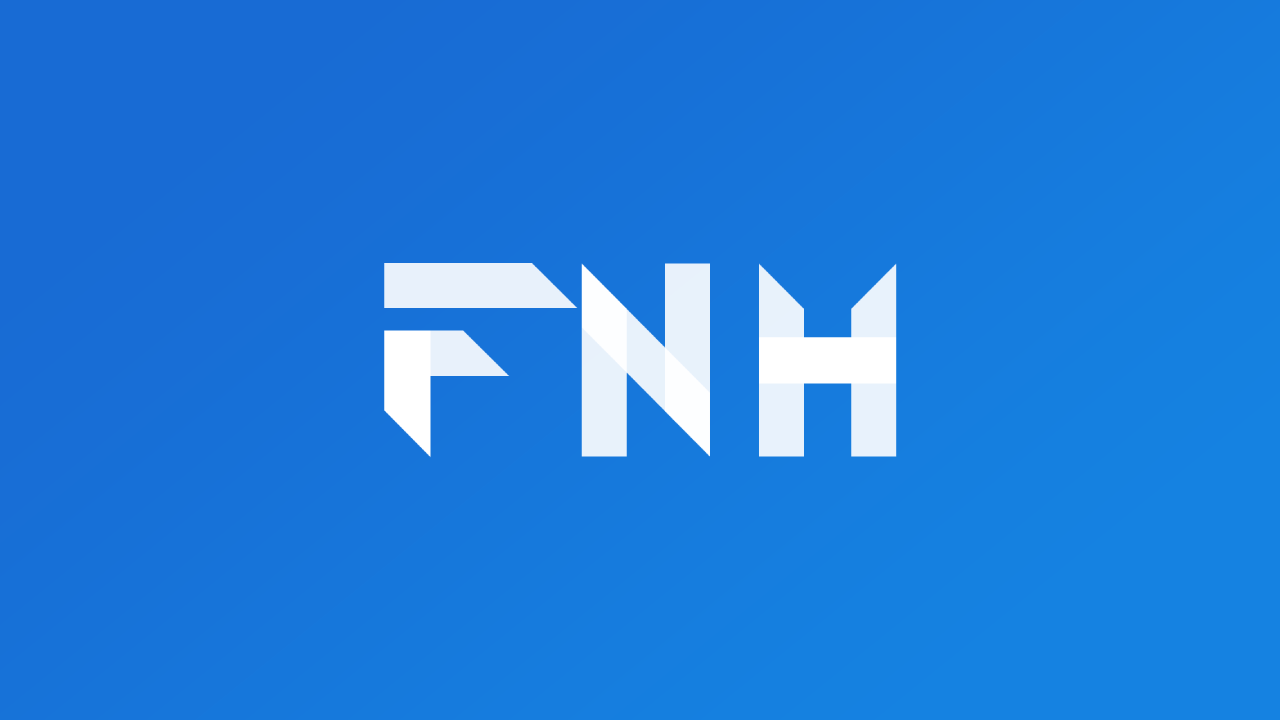
The transparent_image
package provides a simple and convenient way to represent transparent images in Flutter applications. It exports a single constant, kTransparentImage
, which can be used for various purposes, including displaying transparent images, providing a placeholder for loading images, and creating animations.
Usage
Image Widget
To display a transparent image, you can use the Image
widget with the kTransparentImage
constant as the source:
Image.memory(kTransparentImage);
ImageProvider
You can also use the kTransparentImage
constant as an ImageProvider
to provide a transparent image to other widgets:
MemoryImage(kTransparentImage);
FadeInImage Widget
The FadeInImage
widget is a Flutter widget that allows you to fade in an image while it is loading. You can use the kTransparentImage
constant as the placeholder image while the actual image is loading:
FadeInImage.memoryNetwork(
placeholder: kTransparentImage,
image: 'https://picsum.photos/250?image=9',
);
Benefits
Using the transparent_image
package offers several benefits:
- Simplicity: The package is extremely simple to use, with just a single constant to export.
-
Convenience: The
kTransparentImage
constant can be used in various contexts, making it a versatile tool for working with transparent images. - Efficiency: Using a transparent image as a placeholder can improve performance by avoiding unnecessary rendering and loading.
Contributors
The following individuals have contributed to the development of the transparent_image
package:
- Flutter team
- Brian Egan
Code Examples
Displaying a Transparent Image
import 'package:transparent_image/transparent_image.dart';
void main() {
// ...
Widget build(BuildContext context) {
return Image.memory(kTransparentImage);
}
// ...
}
Using Transparent Image as Placeholder
import 'package:transparent_image/transparent_image.dart';
void main() {
// ...
Widget build(BuildContext context) {
return FadeInImage.memoryNetwork(
placeholder: kTransparentImage,
image: 'https://picsum.photos/250?image=9',
);
}
// ...
}
Using Transparent Image as ImageProvider
import 'package:transparent_image/transparent_image.dart';
void main() {
// ...
Widget build(BuildContext context) {
return Image(
image: MemoryImage(kTransparentImage),
);
}
// ...
}