Title: Simplify Data Management with Freezed: A Comprehensive Guide
Published on by Flutter News Hub
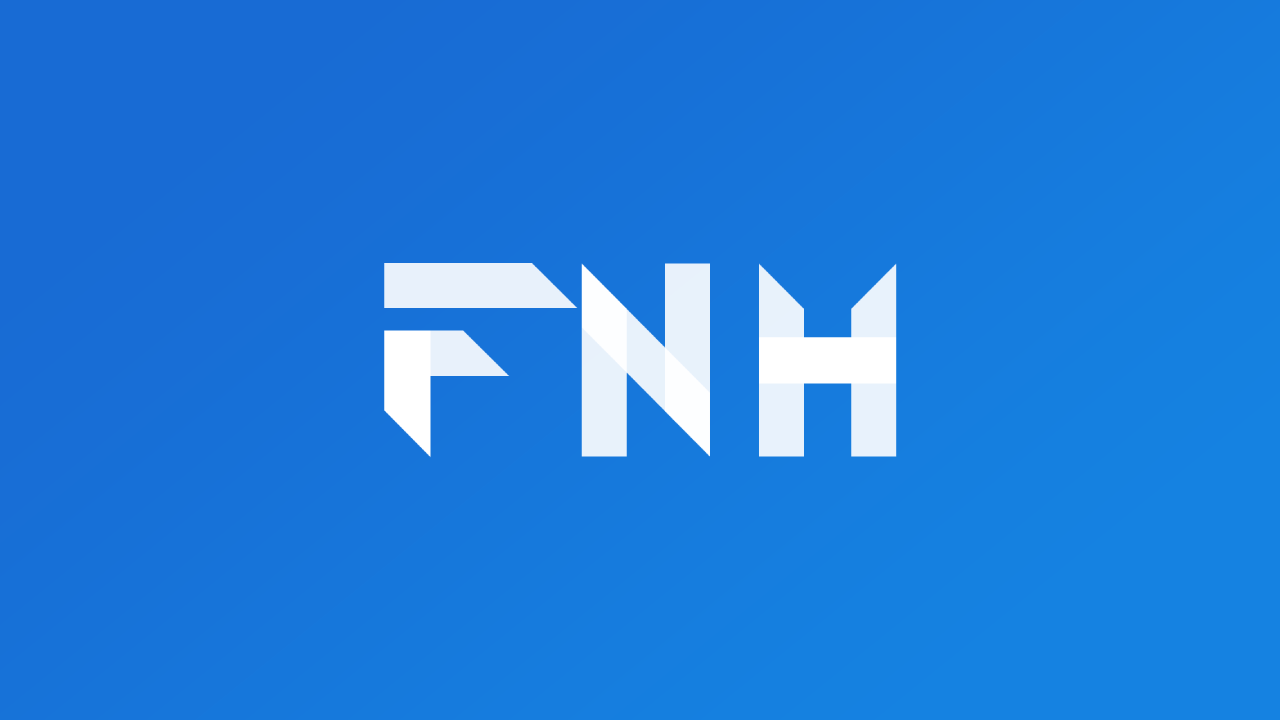
Freezed is a powerful code generator that streamlines the creation of immutable classes, unions, pattern matching, and deep copying in Dart. This comprehensive guide will delve into the functionalities of Freezed, providing practical examples to help you enhance your code.
Defining Immutable Data Structures:
Freezed simplifies the creation of immutable classes by annotating them with @freezed. These classes prevent unintended data modifications, ensuring data integrity. For instance:
@freezed class Person { const factory Person({required String name, required int age}) = _Person; }
Creating Union Types:
Union types allow you to define classes with different variants, each with its own set of properties. In Freezed, union types are annotated with @freezed sealed. For example:
@freezed sealed class Shape { const factory Shape.circle(int radius) = Circle; const factory Shape.rectangle(int width, int height) = Rectangle; }
Pattern Matching with when and map:
To extract data from union types, Freezed provides the when and map methods. when destructures data, while map applies a transformation to each variant.
final Shape shape = Shape.circle(42); shape.when( circle: (radius) => print('Radius: $radius'), rectangle: (width, height) => print('Width: $width, Height: $height'), );
Deep Copying with copyWith:
Freezed automatically generates a copyWith method for immutable classes. This method allows you to create a new instance with modified properties. Consider the following:
var person = Person('John', 24); person = person.copyWith(age: 30); print(person.age); // Outputs 30
Integration with json_serializable:
Freezed can seamlessly integrate with json_serializable for serialization and deserialization. By adding factory Person.fromJson(Map<String, dynamic> json) => _$PersonFromJson(json); to your Freezed class, you can easily deserialize JSON data.
Decorators and Comments Support:
Freezed provides support for decorators and comments, allowing you to enhance your classes with documentation and metadata. For instance, you can use @Assert to add assertions to your constructors, or @JsonKey to specify JSON serialization options.
@freezed class Person { @Assert('name.isNotEmpty', 'Name must not be empty') const factory Person({required String name, required int age}) = _Person; }
Optimizing Generated Code:
For improved performance, Freezed provides a build.yaml file where you can specify various options. For instance, you can disable the generation of when and map methods to reduce code size.
targets: $default: builders: freezed: options: when: false map: false
Conclusion:
Freezed simplifies and enhances data management in Dart, enabling developers to create robust, immutable, and easily maintainable models. By leveraging its powerful features such as union types, pattern matching, and deep copying, you can streamline your codebase and improve its reliability.