The Ultimate Guide to Video Editing with Flutter
Published on by Flutter News Hub
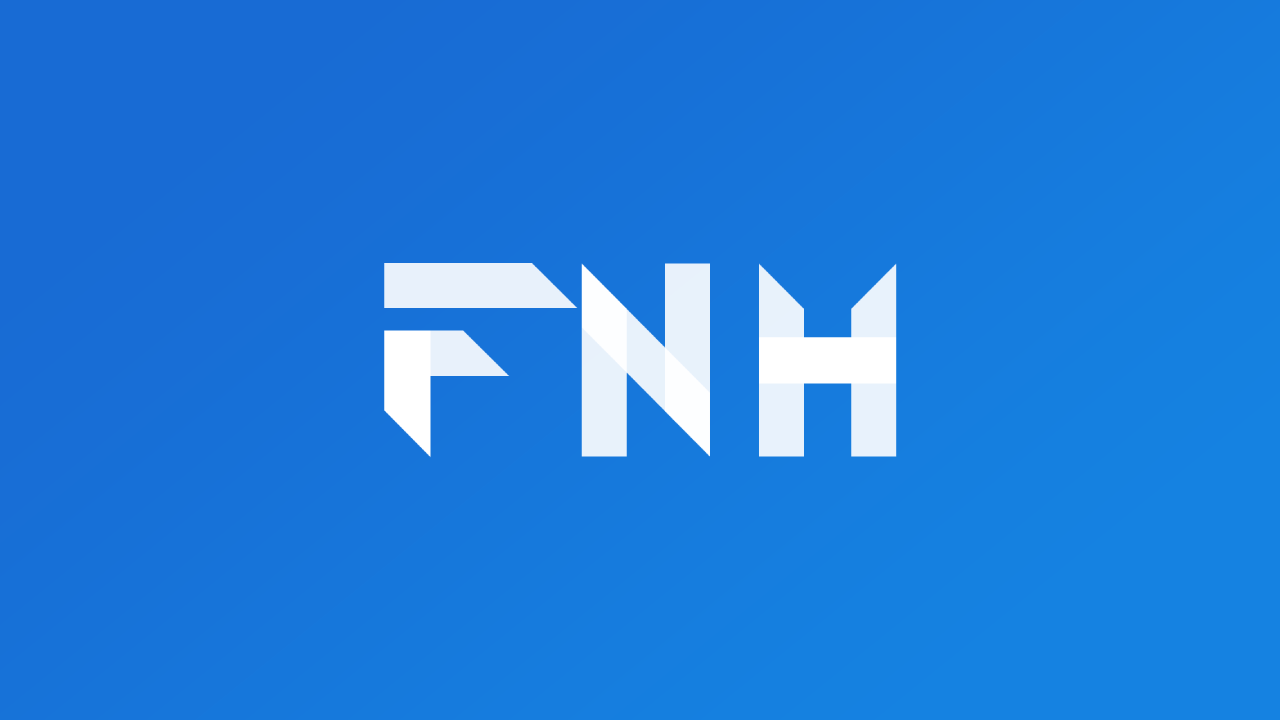
Video editing is a crucial aspect of modern app development, empowering users to create engaging and impactful video content. Flutter, a popular cross-platform UI framework, offers a robust solution for video editing through the video_editor package.
Installation and Usage
To integrate the video_editor package into your Flutter project, follow these steps:
flutter pub add video_editor
Import the package:
import 'package:video_editor/video_editor.dart';
API Overview
The video_editor package provides a comprehensive API for video editing operations:
Method | Description |
---|---|
initialize() | Initializes the video controller with video, trim, and cover parameters |
rotate90Degrees() | Rotates the video 90 degrees in a specified direction |
cropAspectRatio() | Updates the aspect ratio and centers the crop area |
updateCrop() | Updates the crop area's minimum and maximum values |
updateTrim() | Updates the trim area's minimum and maximum values |
exportVideo() | Exports the video using FFmpeg |
exportGif() | Exports the video as a GIF image |
Customizing the Video Editor
Crop Style:
CropStyle(
croppingBackground: Colors.black.withOpacity(0.48),
background: Colors.black,
gridSize: 3,
selectedBoundariesColor: Colors.red,
);
Trim Style:
TrimStyle(
background: Colors.black.withOpacity(0.6),
positionLineColor: Colors.red,
lineColor: Colors.white,
onTrimmingColor: Colors.blue,
);
Cover Style:
CoverStyle(
selectedBorderColor: Colors.white,
borderWidth: 2,
borderRadius: 5.0,
);
Exporting the Video
To export the edited video, you can use the following FFmpegVideoEditorConfig:
FFmpegVideoEditorConfig(
format: VideoExportFormat.mp4,
scale: 0.5, // Resizes the generated file to half the original size
isFiltersEnabled: true, // Applies the editor parameters
);
To export the video as a GIF, use the following CoverFFmpegVideoEditorConfig:
CoverFFmpegVideoEditorConfig(
format: CoverExportFormat.gif,
quality: 100, // Sets the quality of the generated GIF
);
Example
The following code demonstrates basic usage of the video_editor package:
VideoEditorController _controller = VideoEditorController.file(File('/path/to/video.mp4'));
_controller.initialize().then((_) => setState(() {}));
Future exportVideo() async {
final config = VideoFFmpegVideoEditorConfig(_controller);
final FFmpegVideoEditorExecute execute = await config.getExecuteConfig();
// Handle video exportation using ffmpeg_kit_flutter or your own video server
}
Conclusion
The video_editor package is a powerful and customizable solution for video editing in Flutter. It empowers developers to create user-friendly video editing experiences and export high-quality videos effortlessly. With its extensive API and customizable styles, the package provides the flexibility to cater to diverse editing requirements.