Supercharge Your Flutter Desktop App with Window Management
Published on by Flutter News Hub
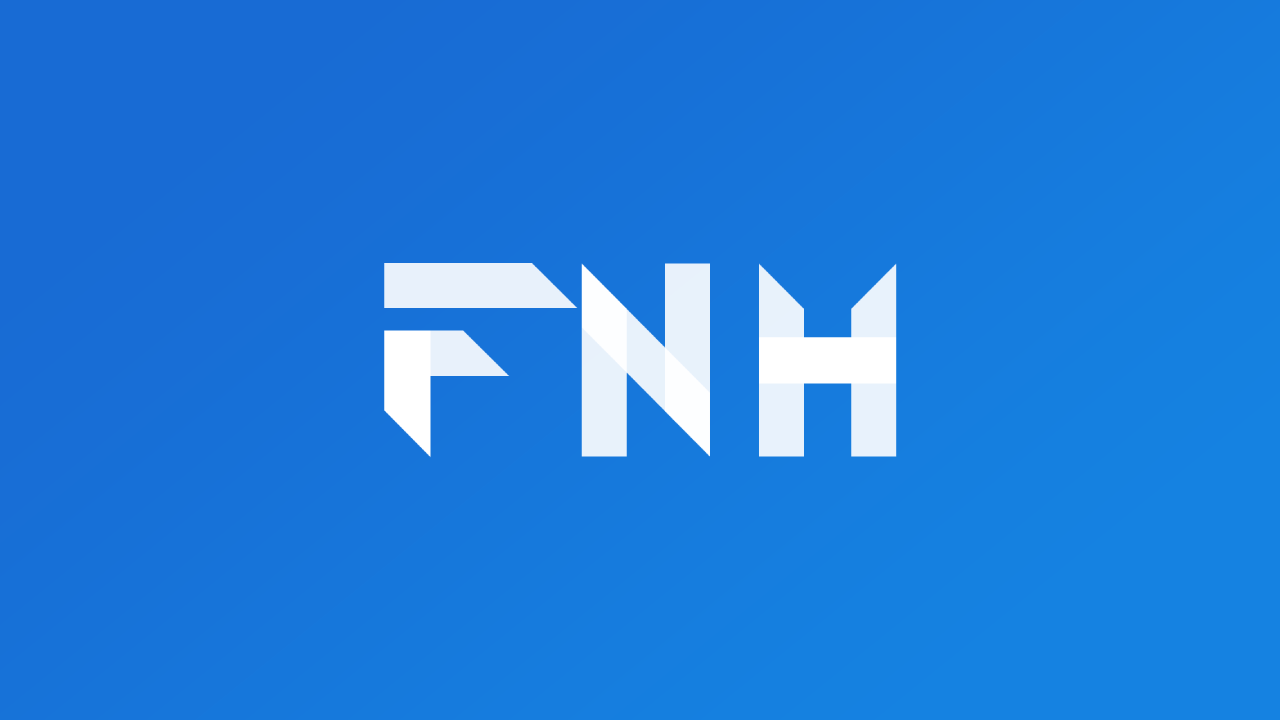
Elevate your Flutter desktop applications to a whole new level with the power of window management. This plugin empowers you to effortlessly control various aspects of your window, including resizing, repositioning, hiding, and more.
Getting Started
- Installation: Add the following line to your pubspec.yaml file:
dependencies: window_manager: ^0.3.8
- Initialization: Initialize the windowManager after ensuring WidgetsFlutterBinding.ensureInitialized().
import 'package:flutter/material.dart'; import 'package:window_manager/window_manager.dart'; void main() async { WidgetsFlutterBinding.ensureInitialized(); await windowManager.ensureInitialized(); // ... Your app code }
Window Control
Resizing and Moving
Resizing and moving the window is a breeze with the setBounds method:
// Set the window's position and size await windowManager.setBounds(Rect.fromLTWH(100, 100, 800, 600));
Maximizing, Minimizing, and Restoring
Maximize, minimize, or restore the window with a single line of code:
// Maximize the window await windowManager.maximize(); // Minimize the window await windowManager.minimize(); // Restore the window to its previous state await windowManager.restore();
Full Screen Management
Toggle full-screen mode with ease:
// Enter full-screen mode await windowManager.setFullScreen(true); // Exit full-screen mode await windowManager.setFullScreen(false);
Window Visibility
Hide or show the window as needed:
// Hide the window await windowManager.hide(); // Show the window await windowManager.show();
Customizing the Window
Modify the window's appearance and behavior:
// Set the window title await windowManager.setTitle('My Awesome App'); // Set the background color await windowManager.setBackgroundColor(Colors.blue); // Disable the title bar await windowManager.setTitleBarStyle(TitleBarStyle.hidden);
Event Handling
Stay informed about window events such as closing, focus changes, and more:
import 'package:flutter/cupertino.dart'; import 'package:window_manager/window_manager.dart'; class MyApp extends StatefulWidget { @override _MyAppState createState() => _MyAppState(); } class _MyAppState extends State with WindowListener { @override void initState() { super.initState(); windowManager.addListener(this); } @override void onWindowClose() { print('Window is closing!'); } }
Conclusion
The window_manager plugin opens up a world of possibilities for Flutter desktop development. Now you can build sophisticated applications that seamlessly integrate with the native windowing system. With its comprehensive API and well-documented usage, this plugin empowers you to create truly exceptional user experiences.