Streamlining Theme Switching in Flutter with Cubit
Published on by Flutter News Hub
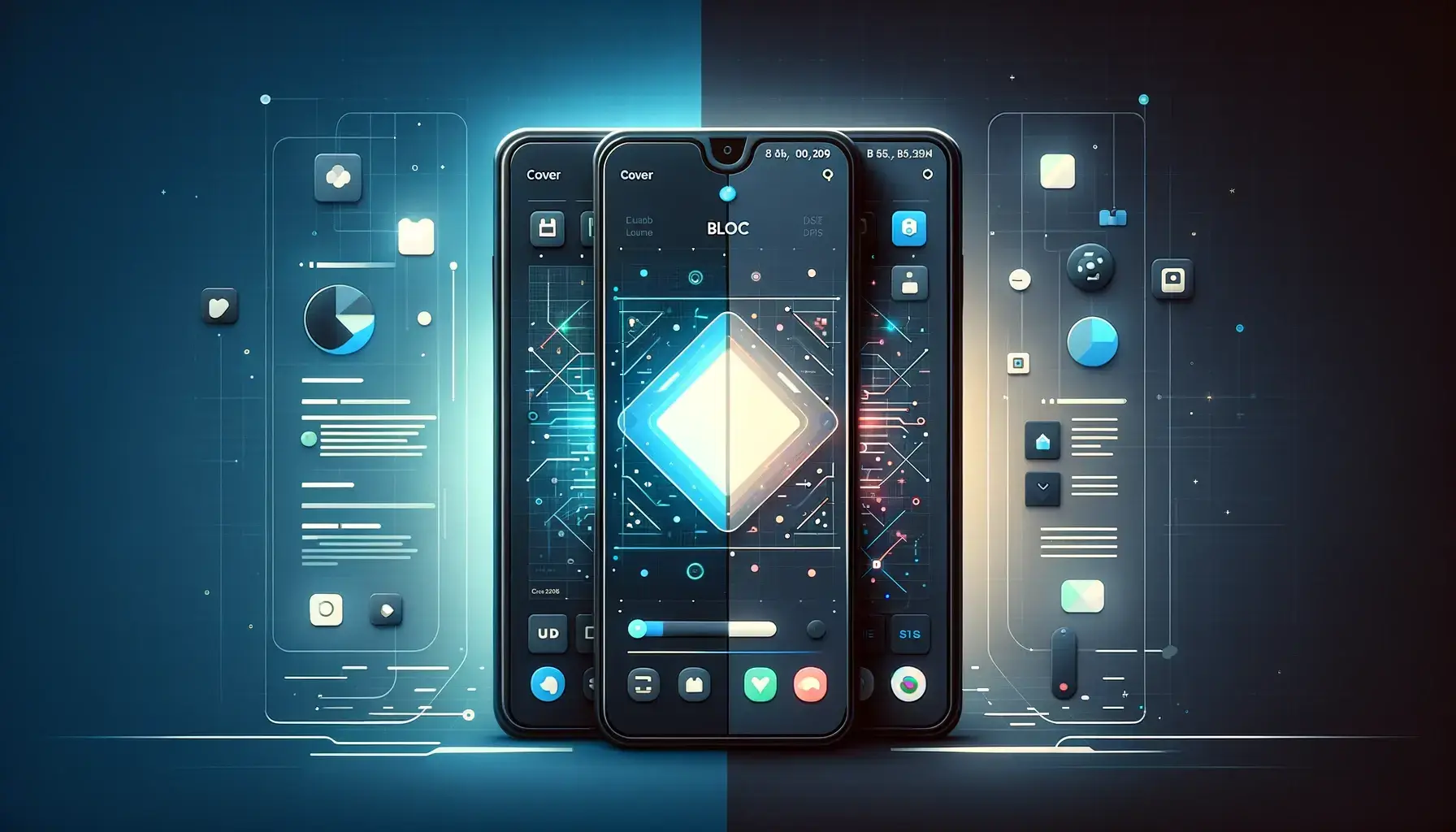
Flutter is renowned for its powerful UI capabilities, and a theme switcher can significantly enhance the user experience. This blog post will guide you through implementing a dynamic theme switcher in Flutter using Cubit, part of the latest version of the Bloc library.
Introduction to Cubit in Flutter
Cubit is a lightweight state management solution that is part of the Bloc package. It offers a simpler API than traditional Bloc, making it ideal for straightforward tasks like managing a theme switcher.
Why Choose Cubit for a Theme Switcher?
- Simplicity: Cubit provides a straightforward way to manage state, perfect for singular-purpose features like theme switching.
- Less Boilerplate: Compared to traditional Bloc, Cubit requires less code, making your implementation cleaner and more maintainable.
- Reactivity and Efficiency: Cubit handles state changes reactively and efficiently, ensuring smooth updates to your app's UI.
Step-by-Step Implementation with Cubit
1. Setting Up Dependencies
Ensure you have the latest Bloc package in your pubspec.yaml:
dependencies: flutter_bloc: ^8.1.0 flutter: sdk: flutter
2. Create Theme Cubit
Implement a Cubit to manage your theme state:
class ThemeCubit extends Cubit<ThemeData> { ThemeCubit() : super(ThemeData.light()); void toggleTheme() { emit(state.brightness == Brightness.dark ? ThemeData.light() : ThemeData.dark()); } }
3. Integrating Cubit into the App
Use BlocProvider to provide the ThemeCubit to your app:
void main() { runApp(BlocProvider( create: (context) => ThemeCubit(), child: MyApp(), )); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return BlocBuilder<ThemeCubit, ThemeData>( builder: (context, themeData) { return MaterialApp( theme: themeData, home: HomeScreen(), ); }, ); } }
4. Adding a Theme Toggle Widget
Create a widget to switch themes:
class ThemeToggle extends StatelessWidget { @override Widget build(BuildContext context) { return Switch( value: context.read<ThemeCubit>().state.brightness == Brightness.dark, onChanged: (_) => context.read<ThemeCubit>().toggleTheme(), ); } }
Conclusion
Using Cubit for a theme switcher in Flutter makes your application adaptable and user-friendly. The simplicity and reduced boilerplate of Cubit make it an excellent choice for managing specific features like theming. This approach ensures your application remains both scalable and maintainable.