Sliver Tools: A Collection of Essential Sliver Widgets
Published on by Flutter News Hub
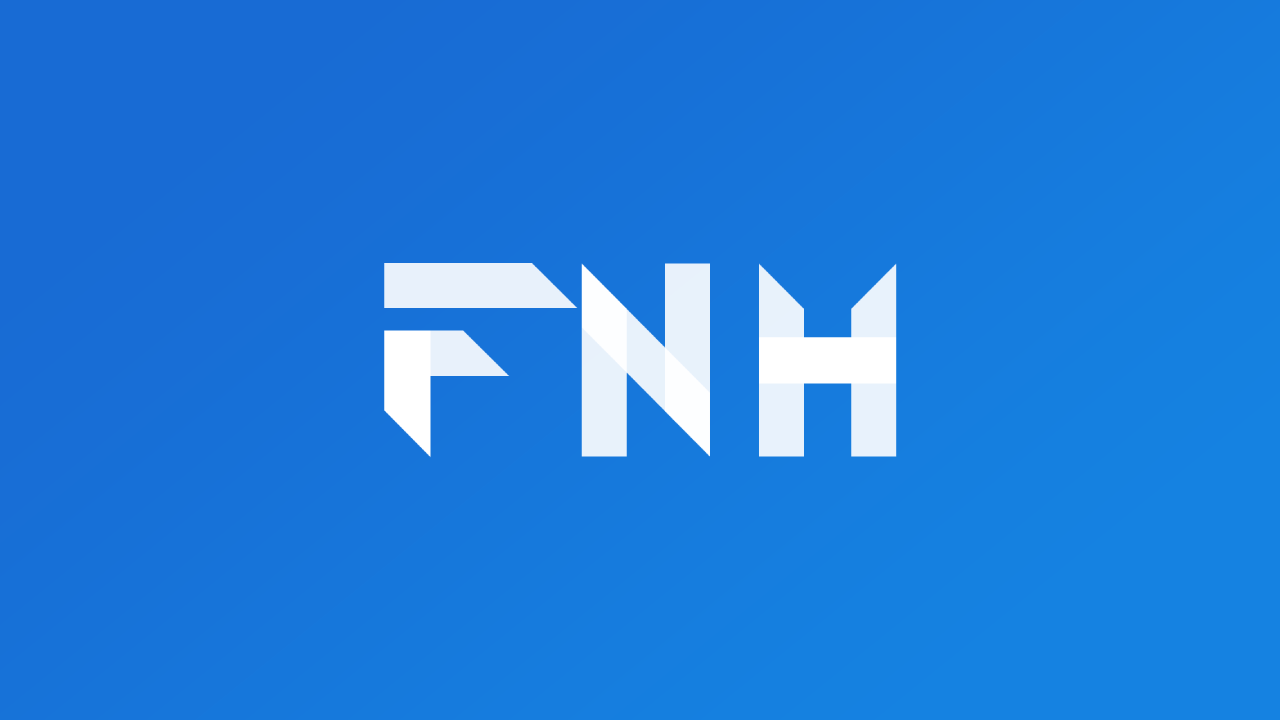
In Flutter, slivers are specialized widgets for building scrolling content within a CustomScrollView. The Sliver package provides a range of basic sliver widgets, but there are often cases where additional functionality is required. That's where the sliver_tools package comes in.
MultiSliver: Grouping Multiple Slivers
The MultiSliver widget allows you to group multiple slivers together and treat them as a single unit. This can be useful for adding padding, decorations, or other effects to a group of slivers.
class WidgetThatReturnsASliver extends StatelessWidget { @override Widget build(BuildContext context) { return MultiSliver( pushPinnedChildren: false, // defaults to false children: [ SliverPersistentHeader(...), SliverList(...), ], ); } }
SliverStack: Stacking Slivers and Box Widgets
The SliverStack widget allows you to stack both slivers and box widgets. This can be used to add decorative elements or other widgets to a sliver.
class WidgetThatReturnsASliver extends StatelessWidget { @override Widget build(BuildContext context) { return SliverStack( insetOnOverlap: false, // defaults to false children: [ SliverPositioned.fill( child: Container( decoration: BoxDecoration( color: Colors.white, boxShadow: const [ BoxShadow( offset: Offset(0, 4), blurRadius: 8, color: Colors.black26, ), ], borderRadius: BorderRadius.circular(8), ), ), ), SliverList(...), ], ); } }
SliverClip: Clipping Sliver Content
The SliverClip widget allows you to clip a sliver's content, preventing it from drawing outside of a specified area. This can be useful for creating effects such as rounded corners or overlapping content.
class WidgetThatReturnsASliver extends StatelessWidget { @override Widget build(BuildContext context) { return SliverClip( clipOverlap: true, // defaults to true child: SliverList(...), ); } }
SliverAnimatedPaintExtent: Animating Sliver Paint Extent
The SliverAnimatedPaintExtent widget allows you to animate the paint extent of a sliver. This can be useful for creating smooth transitions when a sliver's content changes size.
class WidgetThatReturnsASliver extends StatelessWidget { @override Widget build(BuildContext context) { return SliverAnimatedPaintExtent( duration: const Duration(milliseconds: 150), child: SliverList(...), ); } }
SliverAnimatedSwitcher: Animated Transitions for Slivers
The SliverAnimatedSwitcher widget is a pre-configured version of the AnimatedSwitcher widget, tailored specifically for use with slivers. It provides smooth transitions when switching between different sliver children.
SliverCrossAxisConstrained: Constraining Sliver Cross-Axis
The SliverCrossAxisConstrained widget allows you to constrain the cross-axis extent of a sliver, limiting its width or height. This can be useful for creating content that is constrained to a specific size.
class WidgetThatReturnsASliver extends StatelessWidget { @override Widget build(BuildContext context) { return SliverCrossAxisConstrained( maxCrossAxisExtent: 700, alignment: 0, // between -1.0 (left) and 1.0 (right) child: SliverList(...), ); } }
SliverCrossAxisPadded: Padding Sliver Cross-Axis
The SliverCrossAxisPadded widget allows you to add padding to the cross-axis of a sliver, creating additional space around its content. This can be useful for creating layouts with consistent spacing.
class WidgetThatReturnsASliver extends StatelessWidget { @override Widget build(BuildContext context) { return SliverCrossAxisPadded( paddingStart: 24, paddingEnd: 48, textDirection: TextDirection.ltr, // optional, defaults to the Directionality specified by the context child: SliverList(...), ); } }
SliverPinnedHeader: Easily Create Pinned Headers
The SliverPinnedHeader widget simplifies the creation of pinned headers in a scroll view. It ensures that the header remains visible at the top of the viewport even when the user scrolls down.
Conclusion
The sliver_tools package provides a range of highly useful widgets that extend the capabilities of Flutter's built-in sliver widgets. By leveraging these tools, you can create more complex and visually appealing scrolling content with ease.