Sizer: Responsive UI for Flutter Made Easy
Published on by Flutter News Hub
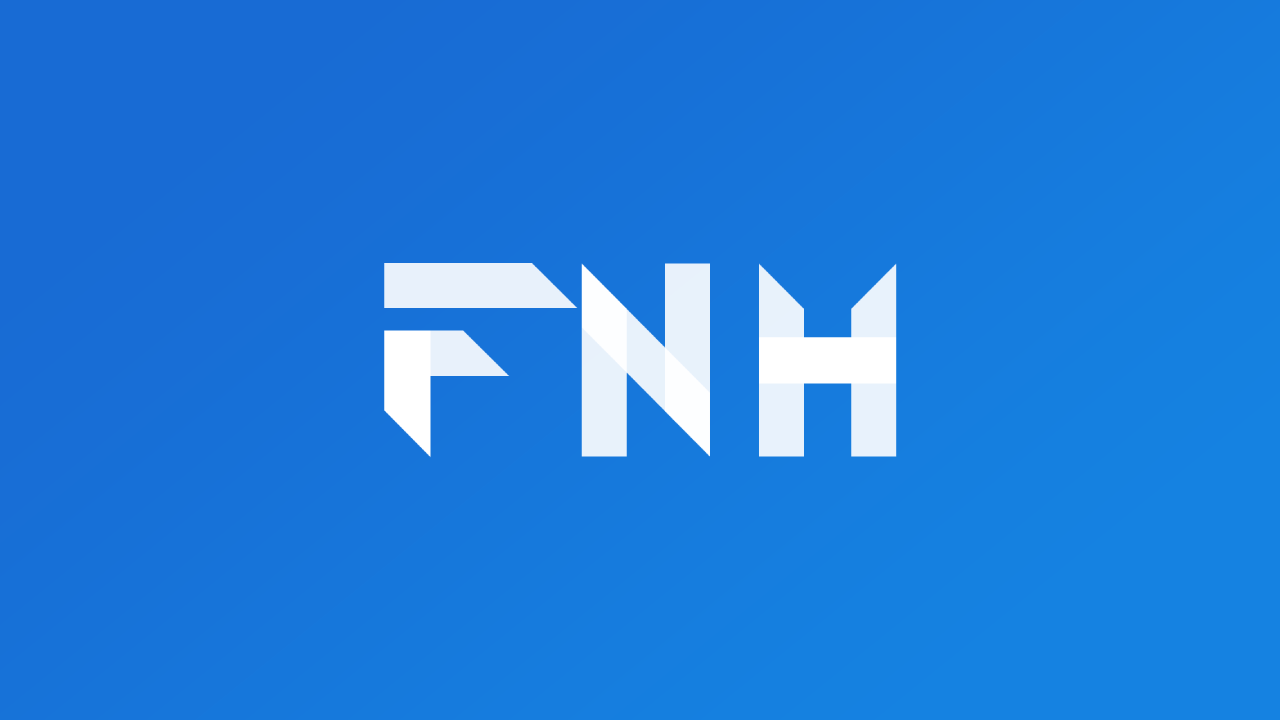
Sizer is a Flutter plugin that simplifies the creation of responsive UIs. It automatically adapts your UI to different screen sizes, making responsiveness a breeze.
Installation
To install Sizer, add the following dependency to your pubspec.yaml file:
dependencies: sizer: ^2.0.15
Usage
Once you've installed Sizer, wrap your MaterialApp widget with the ResponsiveSizer widget:
ResponsiveSizer( builder: (context, orientation, deviceType) { return MaterialApp(); } )
Then, you can start using Sizer's h, w, and sp extensions to specify heights, widths, and font sizes. For example:
Container( width: 20.w, // 20% of screen width height: 30.h, // 30% of screen height )
Text( 'Sizer', style: TextStyle(fontSize: 15.sp), // 15 scaled pixels );
Sizer also provides methods for determining the orientation of the screen (SizerUtil.orientation) and the type of device (SizerUtil.deviceType).
Square Widgets
To create a square widget, simply give the same height and width values:
Container( width: 30.h, height: 30.h, );
Handling Different Orientations
If you want to support both portrait and landscape orientations, you can use the SizerUtil.orientation method:
Device.orientation == Orientation.portrait ? // Widget for Portrait Container( width: 100.w, height: 20.5.h, ) : // Widget for Landscape Container( width: 100.w, height: 12.5.h, )
Handling Different Device Types
If you want to support both mobile and tablet devices, you can use the SizerUtil.deviceType method:
SizerUtil.deviceType == DeviceType.mobile ? // Widget for Mobile Container( width: 100.w, height: 20.5.h, ) : // Widget for Tablet Container( width: 100.w, height: 12.5.h, )
Note
Remember to always import the sizer package when using its extension methods:
import 'package:sizer/sizer.dart';
Auto Import
Auto import in VSCode and Android Studio does not work for Dart extension methods. To fix this, type Device to bring up the auto import suggestion.