Simplify Equality Comparisons with Equatable in Dart
Published on by Flutter News Hub
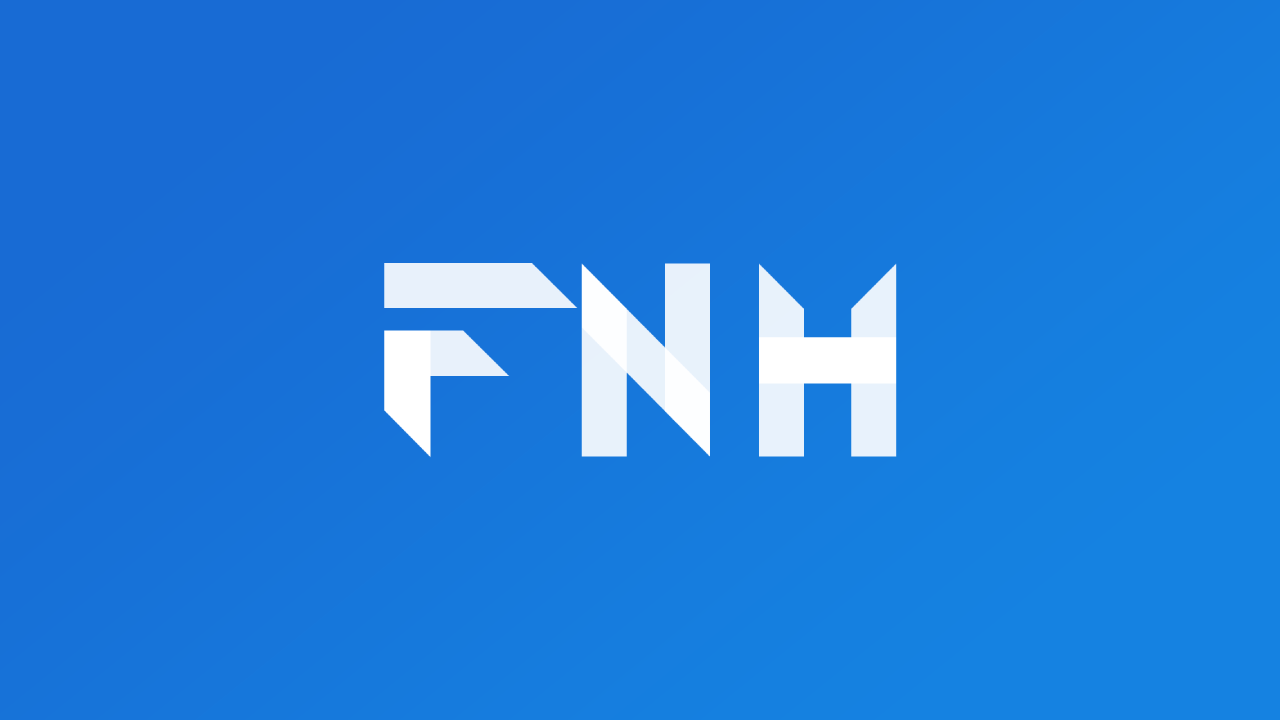
In Dart, comparing objects for equality by default relies on instance identity. To customize equality behavior, developers must manually override the == and hashCode operators, which can be tedious and error-prone.
Equatable to the Rescue
Equatable is a Dart package that simplifies equality comparisons by automatically overriding == and hashCode for immutable classes. It provides a concise and efficient way to compare objects based on their properties.
Usage
To use Equatable, import the package and extend your class from it:
import 'package:equatable/equatable.dart'; class Person extends Equatable { final String name; const Person(this.name); @override List get props => [name]; }
The props getter defines the properties used for comparison. In this example, the name property is used to determine equality.
Example
final person1 = Person("Bob"); final person2 = Person("Bob"); print(person1 == person2); // true
toString
Equatable can also implement the toString method to include the values of the properties used for comparison:
@override bool get stringify => true;
EquatableMixin
For classes that cannot extend Equatable directly, the EquatableMixin can be used to provide similar functionality:
class EquatableDateTime extends DateTime with EquatableMixin { @override List get props => [year, month, day, hour, minute, second, millisecond, microsecond]; }
Performance
Equatable is designed to be efficient. Benchmarks show minimal overhead compared to manual equality implementations:
Conclusion
Equatable simplifies equality comparisons in Dart by automating the generation of custom equality logic. It improves code quality, reduces boilerplate, and minimizes the risk of errors. Embracing Equatable can significantly streamline the process of writing equality-based code in your Dart applications.