Server Universe: A Comprehensive Library for Expediting Server Development
Published on by Flutter News Hub
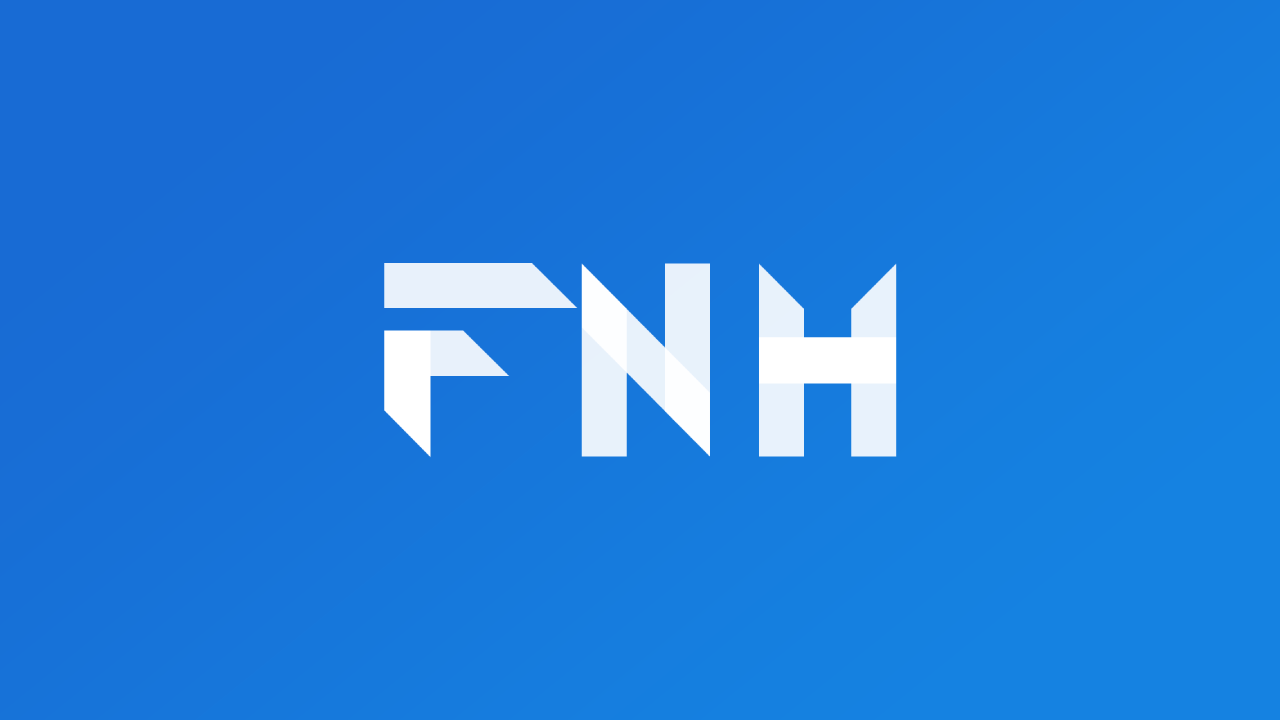
Server Universe is a feature-rich library designed to simplify and accelerate the development of server-side applications. It offers a standardized code style, CLI (command-line interface), API, customizable extensions, user-friendly information, and cross-platform support.
Features
- Cross-platform compatibility (devices, Edge Severless functions)
- Standarization Style Code
- Command-line interface (CLI)
- API (suitable for bot or userbot development)
- Customizable Extension (add extensions for enhanced development speed)
- Pretty Information (user-friendly for beginners)
Installation
Dart
dart pub add server_universe
Flutter
flutter pub add server_universe_flutter
CLI
dart pub global activate server_universe
Quick Start
API
import 'dart:io';
import 'package:server_universe/api/server_universe_api.dart';
void main(List args) async {
ServerUniverseApi serverUniverseApi = ServerUniverseApi();
serverUniverseApi.create(newName: "hi", directoryBase: Directory("path_to_dir/slebew")).listen((event) {
print(event.value);
});
serverUniverseApi.build(directoryBase: Directory("path_to_dir/slebew"), directoryOutputBuildServerUniverse: Directory("path_to_dir/slebew/build"), inputFileName: "path_to_dir/slebew/bin/server.dart", server_universeDartBuildType: ServerUniverseBuildType.release, server_universeDartPlatformType: ServerUniversePlatformType.supabase).listen((event) {
print(event.value);
});
}
CLI
dart run server_universe
Edge
import 'package:server_universe/edge/edge.dart';
void main() async {
print("start");
ServerUniverseEdge app = ServerUniverseEdge(
onNotFound: (request, res) async {
return res.status(404).json({
"@type": "error",
"message": "path_not_found",
"description": "PATH: $ Not Found"
});
},
onError: (req, res, object, stackTrace) {
return res.status(500).json({
"@type": "error",
"message": "server_crash"
});
},
);
app.ensureInitialized();
app.all("/", (req, res) {
return res.send("oke");
});
app.all("/version", (req, res) {
return res.json({
"@type": "version",
"version": "new update",
});
});
}
Native
import 'dart:io';
import 'package:general_lib/extension/dynamic.dart';
import 'package:server_universe/native.dart';
void main() async {
print("start");
int port = int.tryParse(Platform.environment["PORT"] ?? "3000") ?? 3000;
String host = Platform.environment["HOST"] ?? "0.0.0.0";
ServerUniverseNative app = ServerUniverseNative(
logLevel: LogType.debug,
onNotFound: (request, res) async {
return res.status(400).send(({
"@type": "error",
"message": "path_not_found",
"description": "PATH: Not Found",
}.toStringifyPretty()));
},
);
app.all("/", (req, res) {
return res.status(200).send("oke");
});
int count = 0;
app.all("/version", (req, res) async {
count++;
return await res.status(200).send("\nCOUNT: $");
});
await app.listen(port: port, bindIp: host);
print("Server on");
}
Conclusion
Server Universe is a powerful and versatile library that streamlines server development. Its cross-platform compatibility, customizable features, and user-friendly design make it an ideal choice for projects ranging from simple server-side applications to complex Edge functions.