Server Universe: A Comprehensive Guide to Server-Side Development
Published on by Flutter News Hub
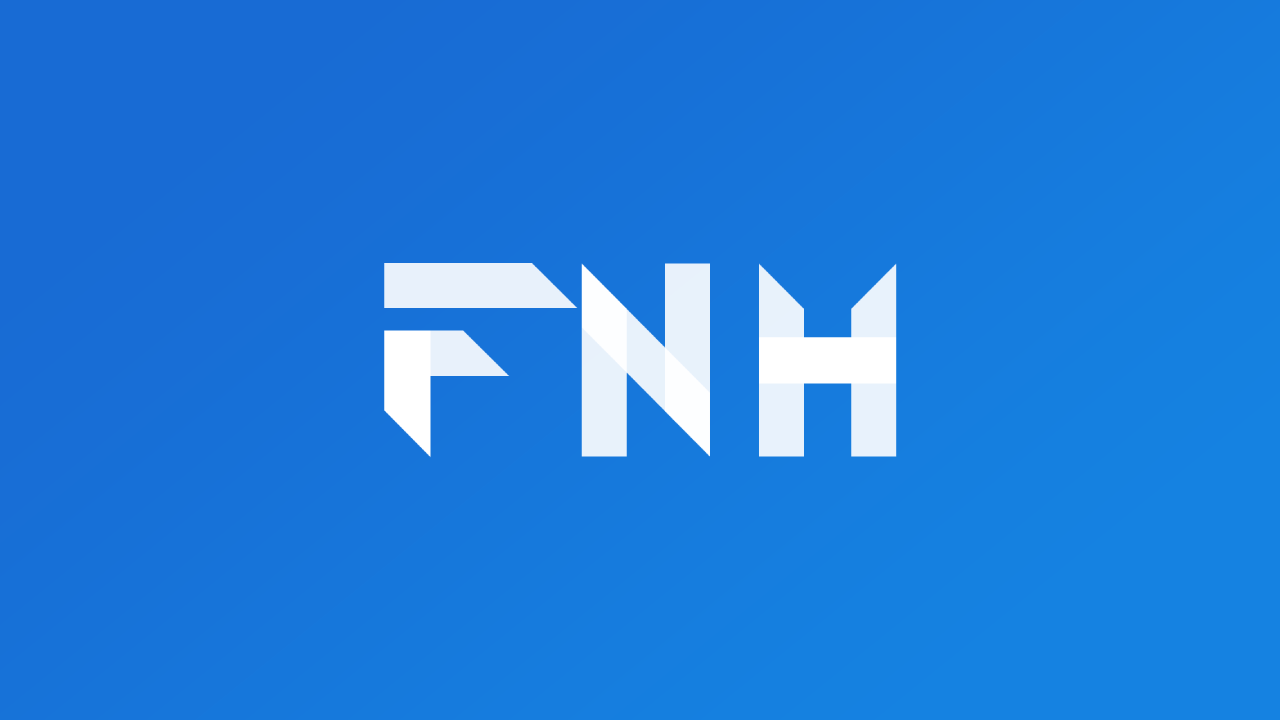
Server Universe is a versatile library designed to streamline server-side development across multiple platforms, including devices, edge serverless functions, and more. Its cross-platform nature and customizable features make it an ideal choice for developers of any skill level.
Features
- Cross-Platform Support: Deploy your server code on various platforms, including devices, edge serverless functions, and native environments.
- Standardization: Enforces consistent code style for enhanced readability and maintainability.
- Command-Line Interface (CLI): Provides a command-line tool for easy project creation, management, and documentation.
- API: Integrates with your codebase for greater flexibility and control.
- Customizable Extensions: Allows you to add custom extensions for advanced functionality and tailored solutions.
- User-Friendly Interface: Supports beginner-level developers with its intuitive interface and straightforward documentation.
Quick Start
API
import 'package:server_universe_dart/native/native.dart';
void main() async {
print("start");
int port = int.tryParse(Platform.environment["PORT"] ?? "3000") ?? 3000;
String host = Platform.environment["HOST"] ?? "0.0.0.0";
ServerUniverseNative app = ServerUniverseNative(
onNotFound: (request, res) async {
return res.json({
"@type": "error",
"message": "path_not_found",
"description": "PATH: Not Found"
});
},
onInternalError: (req, res) {
return res.json({
"@type": "error",
"message": "server_crash"
});
},
);
app.all("/", (req, res) {
return res.send("oke");
});
app.all("/version", (req, res) {
return res.json({
"@type": "version",
"version": "0.0.0",
});
});
await app.listen(port, host);
print("Server on");
}
CLI
dart pub add server_universe_dart
Edge
import 'package:server_universe_dart/edge/edge.dart';
void main() async {
print("start");
ServerUniverseEdge app = ServerUniverseEdge(
onNotFound: (request, res) async {
return res.status(404).json({
"@type": "error",
"message": "path_not_found",
"description": "PATH: $ Not Found"
});
},
onError: (req, res, object, stackTrace) {
return res.status(500).json({
"@type": "error",
"message": "server_crash"
});
},
);
app.ensureInitialized();
app.all("/", (req, res) {
return res.send("oke");
});
app.all("/version", (req, res) {
return res.json({
"@type": "version",
"version": "0.0.0",
});
});
}
Native
import 'dart:io';
import 'package:server_universe_dart/native/native.dart';
void main() async {
print("start");
int port = int.tryParse(Platform.environment["PORT"] ?? "3000") ?? 3000;
String host = Platform.environment["HOST"] ?? "0.0.0.0";
ServerUniverseNative app = ServerUniverseNative(
onNotFound: (request, res) async {
return res.json({
"@type": "error",
"message": "path_not_found",
"description": "PATH: Not Found"
});
},
onInternalError: (req, res) {
return res.json({
"@type": "error",
"message": "server_crash"
});
},
);
app.all("/", (req, res) {
return res.send("oke");
});
app.all("/version", (req, res) {
return res.json({
"@type": "version",
"version": "0.0.0",
});
});
await app.listen(port, host);
print("Server on");
}
Conclusion
Server Universe is a powerful and versatile toolkit that simplifies server-side development and enhances efficiency. Its cross-platform capabilities, user-friendly interface, and customizable features make it a go-to choice for developers seeking speed and reliability in their server-side projects.