Server Universe: A Comprehensive Guide for Fast and Customizable Server Rest API Development
Published on by Flutter News Hub
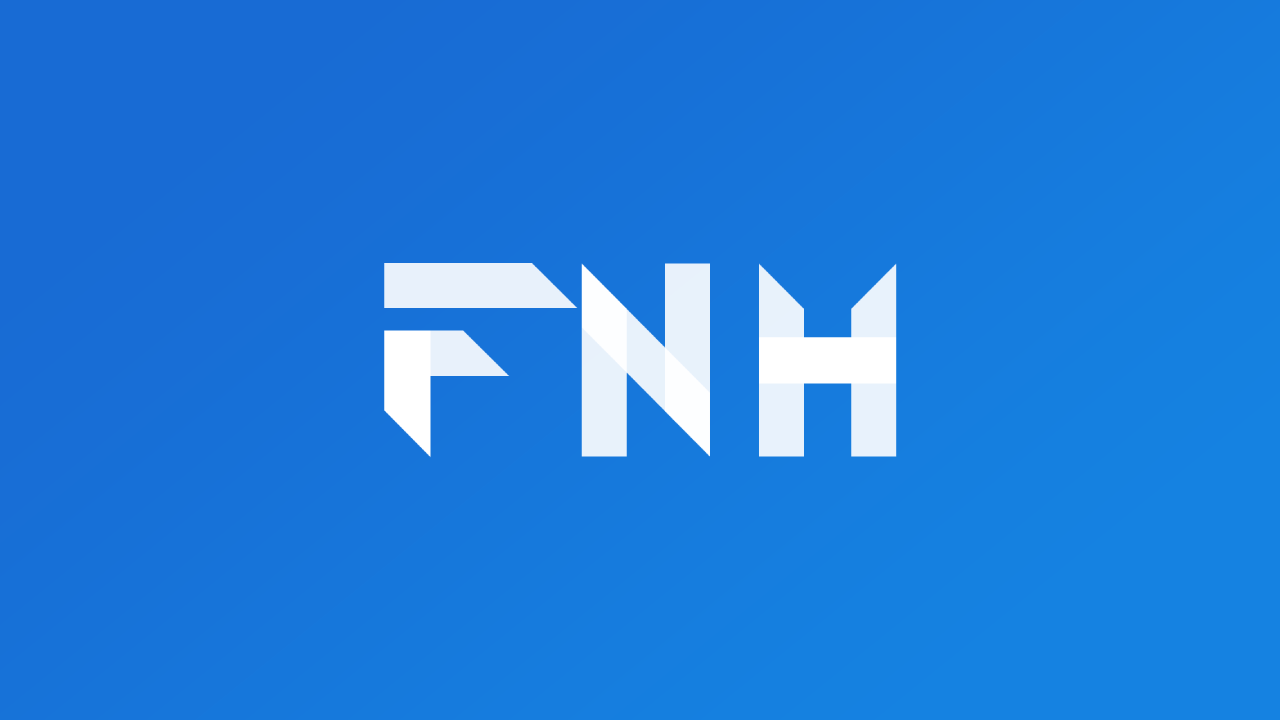
Server Universe is a library that empowers developers to create high-performance and highly customizable server rest APIs with ease. It supports multiple platforms, including mobile, edge servers, and serverless functions, enabling seamless deployment in various environments.
Key Features of Server Universe
- Cross-platform support for device, edge serverless functions, and server side
- Code standardization for enhanced readability and maintainability
- Built-in CLI for command-line assistance and project creation
- Extensible architecture allows for custom extensions to accelerate development
- User-friendly documentation for beginners and experienced developers alike
Benefits of Using Server Universe
- Speed: Boasts an optimized codebase for faster server performance.
- Customization: Offers granular customization options to tailor APIs to specific requirements.
- Cross-platform: Supports deployment on diverse platforms, increasing flexibility and accessibility.
- Simplicity: Simplifies server development with intuitive API design and minimal code configuration.
- Documentation: Provides extensive documentation and tutorials for seamless onboarding and learning.
Installation
Dart and Flutter
-
Dart:
dart pub add server_universe
-
Flutter:
flutter pub add server_universe_flutter
CLI Installation
-
dart pub global activate server_universe
Getting Started with Server Universe
API Usage
import 'package:server_universe/api/server_universe_api.dart';
void main() async {
ServerUniverseApi serverUniverseApi = ServerUniverseApi();
serverUniverseApi.create(newName: "hi", directoryBase: Directory("path_to_dir/slebew")).listen((event) { print(event.value); });
serverUniverseApi.build(directoryBase: Directory("path_to_dir/slebew"), directoryOutputBuildServerUniverse: Directory("path_to_dir/slebew/build"), inputFileName: "path_to_dir/slebew/bin/server.dart", server_universeDartBuildType: ServerUniverseBuildType.release, server_universeDartPlatformType: ServerUniversePlatformType.supabase).listen((event) { print(event.value); });
}
CLI Usage
dart run server_universe
Edge Usage
import 'package:server_universe/edge/edge.dart';
void main() async {
print("start");
ServerUniverseEdge app = ServerUniverseEdge( onNotFound: (request, res) async { return res.status(404).json({"@type": "error", "message": "path_not_found", "description": "PATH: $ Not Found"}); }, onError: (req, res, object, stackTrace) { return res.status(500).json({"@type": "error", "message": "server_crash"}); }, );
app.ensureInitialized();
app.all("/", (req, res) { return res.send("oke"); });
app.all("/version", (req, res) { return res.json({ "@type": "version", "version": "new update", }); });
}
Native Usage
import 'dart:io';
import 'package:general_lib/extension/dynamic.dart';
import 'package:server_universe/native.dart';
void main() async {
print("start");
int port = int.tryParse(Platform.environment["PORT"] ?? "3000") ?? 3000;
String host = Platform.environment["HOST"] ?? "0.0.0.0";
ServerUniverseNative app = ServerUniverseNative( logLevel: LogType.debug, onNotFound: (request, res) async { return res.status(400).send(({ "@type": "error", "message": "path_not_found", "description": "PATH: Not Found", }.toStringifyPretty())); }, );
app.all("/", (req, res) { return res.status(200).send("oke"); });
int count = 0;
app.all("/version", (req, res) async { count++; return await res.status(200).send("\nCOUNT: $"); });
await app.listen(port:port, bindIp: host);
print("Server on");
}
Further Exploration
Extension Development
Server Universe supports the development of custom extensions to enhance its functionality. Refer to the documentation for detailed instructions on creating and integrating your extensions.
Client-Side Integration
For client-side applications, consider using the server_universe_flutter package to seamlessly interact with Server Universe servers.
Troubleshooting and Support
Extensive documentation and support resources are available for Server Universe. Visit the official documentation, join the support group, or reach out to the developer directly for assistance.
Conclusion
Server Universe is an invaluable tool for developers seeking to create fast, customizable, and cross-platform server rest APIs. With its comprehensive features and extensive support, it empowers you to build robust and tailored server solutions with ease.