Sembast.dart: A Simple, Embedded Database Solution for Dart
Published on by Flutter News Hub
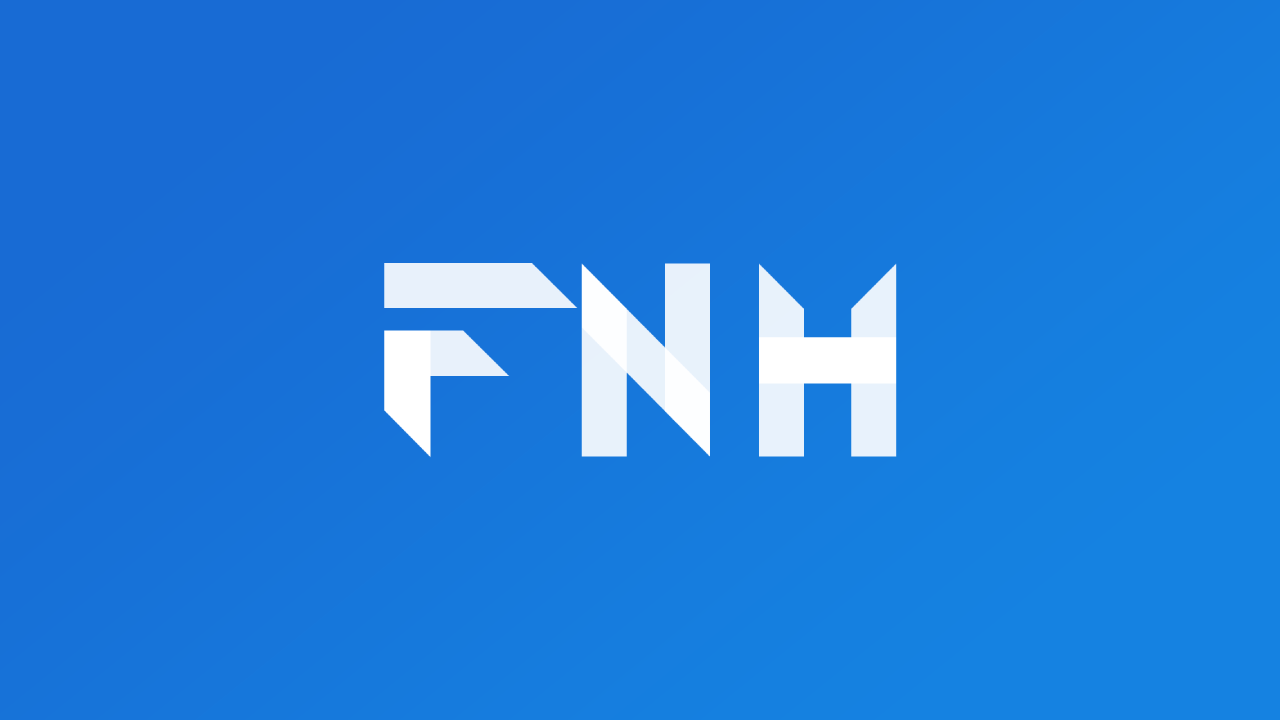
Sembast.dart is a powerful and easy-to-use NoSQL database solution for single-process Dart applications. This database resides in a single file, which is loaded into memory when opened. Changes are appended immediately to the file and the file is automatically compacted when needed.
Opening a Sembast.dart Database
To open a Sembast.dart database, you will need a file path and a database factory. For example:
String dbPath = 'sample.db'; DatabaseFactory dbFactory = databaseFactoryIo; Database db = await dbFactory.openDatabase(dbPath);
Working with Sembast.dart Stores and Records
A store is a collection of records that share a common data structure. The main store is a general-purpose store that can be used for storing any type of data. To create a new store, you can use the following code:
var store = StoreRef.main();
To add a record to a store, you can use the put() method. For example:
await store.record('title').put(db, 'Simple application'); await store.record('version').put(db, 10); await store.record('settings').put(db, {'offline': true});
To read a record from a store, you can use the get() method. For example:
var title = await store.record('title').get(db) as String; var version = await store.record('version').get(db) as int; var settings = await store.record('settings').get(db) as Map;
To delete a record from a store, you can use the delete() method. For example:
await store.record('version').delete(db);
Performing Transactions in Sembast.dart
Transactions are used to group multiple database operations together to ensure that they are either all committed or all rolled back. To create a transaction, you can use the following code:
await db.transaction((txn) async { await store.add(txn, {'name': 'fish'}); await store.add(txn, {'name': 'cat'}); });
Filtering and Sorting Data in Sembast.dart
Sembast.dart supports filtering and sorting data using the Finder class. For example, the following code finds all animals with a name greater than "cat":
var finder = Finder(filter: Filter.greaterThan('name', 'cat'), sortOrders: [SortOrder('name')]); var records = await store.find(db, finder: finder);
Listening to Changes in Sembast.dart
You can listen to changes in a store or query using the snapshots() method. For example, the following code listens to changes in the main store:
var subscription = store.snapshots().listen((snapshot) { // Handle changes to the store });
Conclusion
Sembast.dart is a powerful and versatile database solution for Dart applications. It is easy to use, supports a variety of data types, and offers a range of features for working with data. Whether you are developing a mobile app, a web app, or a desktop app, Sembast.dart can meet your database needs.