Seamless Cross-Platform Development with Universal HTML
Published on by Flutter News Hub
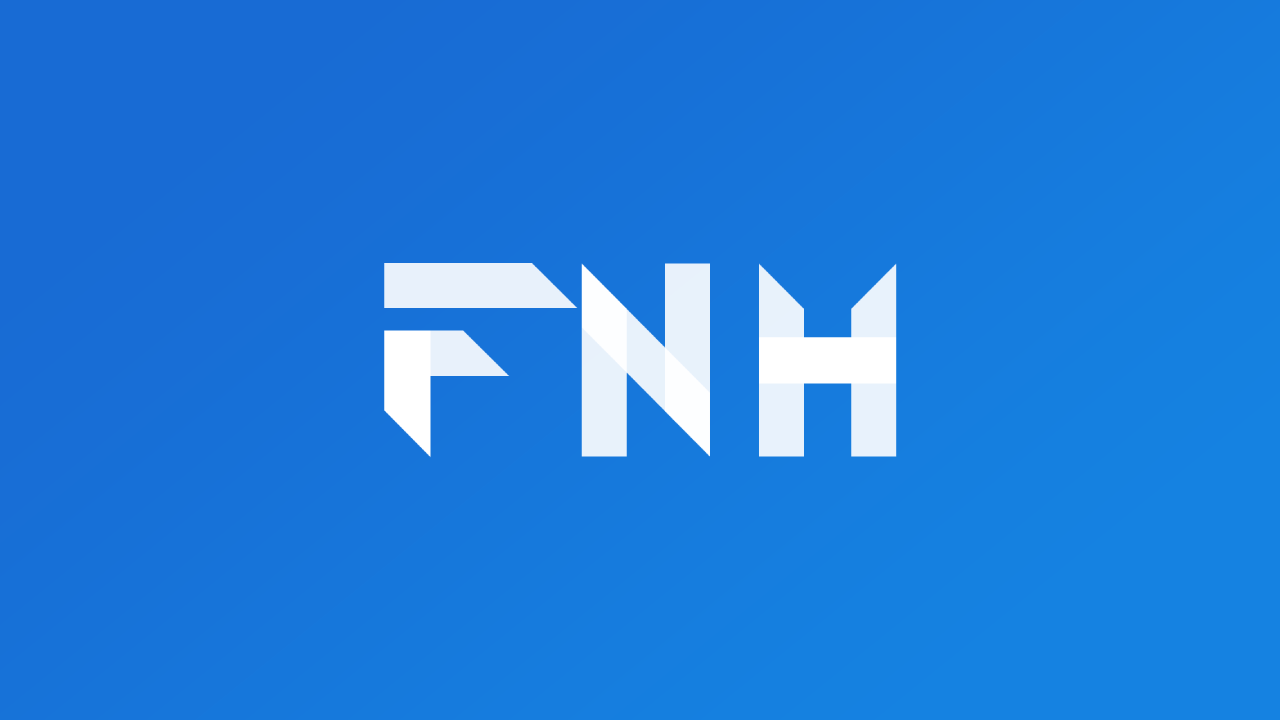
Universal HTML is a revolutionary package that empowers developers with cross-platform compatibility for the HTML DOM API. It's a powerful tool that enables you to write code that seamlessly runs in browsers, mobile devices, desktops, and even Node.JS environments. By simply replacing your 'dart:html' imports with 'package:universal_html/html.dart,' you can eliminate the need for platform-specific code and enjoy the benefits of consistent development across various environments.
Benefits of Universal HTML
- Cross-platform Harmony: Develop code universally for browsers, mobile, desktop, and server-side VM, as well as Node.JS.
- DOM Manipulation Made Easy: Parse, navigate, and modify HTML and XML documents with ease using intuitive methods.
- CSS Query Support: Leverage CSS queries like 'querySelectorAll' to pinpoint DOM nodes for efficient manipulation.
- EventSource Streaming: Access EventSource (SSE) streaming capabilities, a crucial feature for real-time data transfer.
Getting Started
- Add Dependency: Include universal_html in your 'pubspec.yaml' file:
dependencies:
universal_html: ^2.2.4
- Import and Use: Replace 'import 'dart:html;' with 'import "package:universal_html/html.dart";'
import 'package:universal_html/html.dart';
void main() {
// Create DOM elements...
// Manipulate DOM...
}
Examples
Parsing HTML:
import 'package:universal_html/parsing.dart';
void main() {
final htmlDocument = parseHtmlDocument('...');
}
Parsing XML:
import 'package:universal_html/parsing.dart';
void main() {
final xmlDocument = parseXmlDocument('...');
}
Scraping a Website:
import 'package:universal_html/controller.dart';
Future main() async {
final controller = WindowController();
await controller.openHttp(
uri: Uri.parse("https://news.ycombinator.com/"),
onRequest: (request) {
// Set custom headers or cookies if needed...
},
onResponse: (response) {
// Process the response...
},
);
}
EventSource Streaming:
import 'package:universal_html/html.dart';
Future main() async {
final eventSource = EventSource('http://example.com/events');
await for (var message in eventSource.onMessage) {
// Process event...
}
}
Testing:
import 'package:universal_html/controller.dart';
import 'package:test/test.dart';
void main() {
setUp(() {
WindowController.instance = WindowController();
});
test('test #1', () {
// ...
});
}
Conclusion
Universal HTML is an indispensable tool for developers who prioritize cross-platform compatibility and efficient HTML manipulation. Its versatility and ease of use make it a powerful solution for modern web and app development. Embrace Universal HTML and elevate your development experience!