Screen Go: A Comprehensive Guide to Responsive UI Design in Flutter
Published on by Flutter News Hub
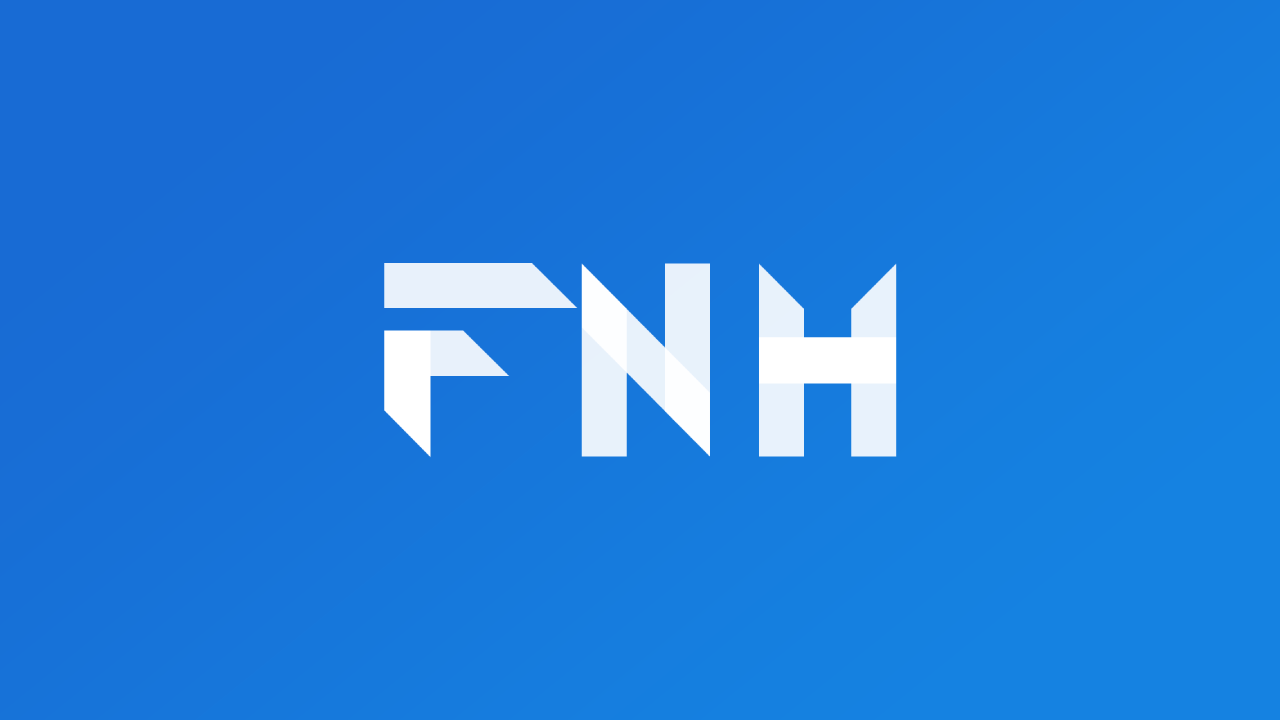
Screen Go is a powerful Flutter package that simplifies the development of responsive user interfaces. With a range of helper widgets and extensions, you can effortlessly create layouts that adapt seamlessly to different screen sizes and orientations.
Installation:
Add screen_go
to your pubspec.yaml
file:
dependencies:
screen_go:
Usage:
Import the Package:
import 'package:screen_go/screen_go.dart';
Wrap MaterialApp:
Wrap your MaterialApp
with the ResponsiveSizer
widget to enable responsive behavior:
ScreenGo(
materialApp: true,
builder: (context, deviceInfo) {
return MaterialApp(
home: HomePage(),
);
},
);
Widget Size:
Use the h
and w
extensions to set widget sizes based on device height and width:
Container(
width: 20.w, // 20% of device's width
height: 30.5.h, // 30.5% of device's height
)
Font Size:
Set font sizes using the sp
extension, which calculates based on pixel density:
Text(
'Screen Go',
style: TextStyle(fontSize: 15.sp),
)
Padding:
Use h
and w
extensions to set padding based on screen dimensions:
Padding(
padding: EdgeInsets.symmetric(vertical: 5.h, horizontal: 3.w),
child: Container(),
);
Icon Size:
Scale icon sizes with the sp
extension:
Icon(
Icons.home,
size: 12.sp,
);
Orientation Handling:
otv() Method:
Set different values for portrait and landscape orientations:
otv(
context: context,
portrait: Container(
width: 100.w,
height: 20.5.h,
),
landscape: Container(
width: 100.w,
height: 12.5.h,
),
)
stv() Method:
Set different values for mobile, tablet, and desktop screen types:
stv(
context: context,
mobile: Container(
width: 100.w,
height: 20.5.h,
),
tablet: Container(
width: 100.w,
height: 12.5.h,
),
desktop: Container(
width: 100.w,
height: 12.5.h,
),
)
ResponsiveScreen Widget:
Create widgets for specific screen types:
ResponsiveScreen(
mobile: Container(
child: Text('For Mobile Screen'),
),
tablet: Container(
child: Text('For Tablet Screen'),
),
desktop: Container(
child: Text('For Desktop Screen'),
),
)
Advanced Responsive Features:
The ScreenGo
widget provides access to detailed device information:
Scaffold(
body: ScreenGo(
builder: (context, deviceInfo) {
return Container(
height: deviceInfo.screenHeight,
width: deviceInfo.screenWidth,
child: SizedBox(
height: deviceInfo.parentHeight / 2,
width: deviceInfo.parentWidth / 2,
),
);
},
),
)
Extensions for Unit Conversion:
Screen Go provides extensions for converting values between different units:
10.cm // 10 centimeters
10.mm // 10 millimeters
10.Q // 10 quarter-millimeters
10.inches // 10 inches
10.pc // 10 picas
10.pt // 10 points
10.px // 10 pixels