RxDart: Enhancing Dart Streams with Reactive Extensions
Published on by Flutter News Hub
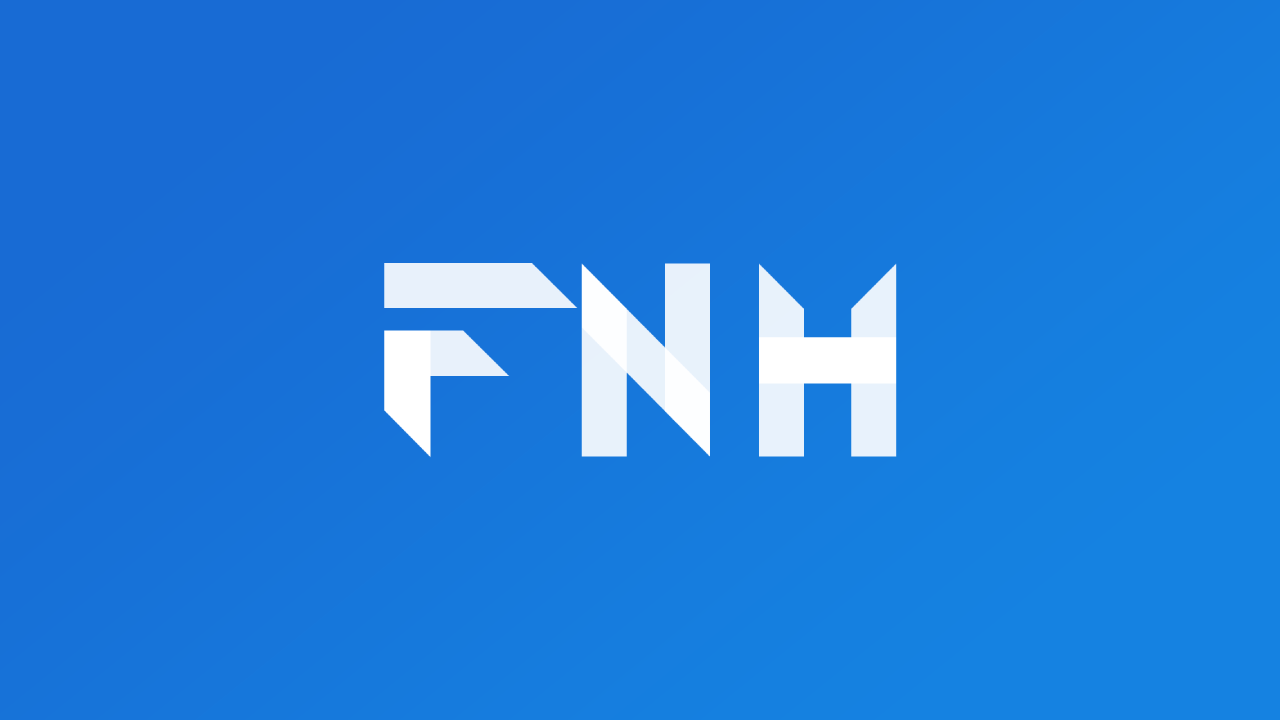
RxDart is a Dart library that extends the capabilities of Dart Streams. It introduces reactive extensions that enhance the functionality and flexibility of streams, making them more suitable for complex data processing tasks.
Key Benefits of RxDart
- Adds additional stream classes for combining and merging multiple streams.
- Provides extension methods that transform streams with operators such as filtering, mapping, and throttling.
- Offers Subjects, which are StreamControllers with additional capabilities like caching and broadcasting.
Stream Classes
RxDart provides several stream classes for specific use cases:
MergeStream: Merges multiple streams into a single stream. ConcatStream: Concatenates streams, emitting events from each stream in sequence. CombineLatestStream: Emits events that combine the latest values from multiple streams. ForkJoinStream: Joins multiple streams and emits a single event containing the combined results.
Extension Methods
Extension methods can be applied to any stream to modify its behavior:
debounce: Delays the emission of events by a specified duration. distinctUnique: Ignores consecutive duplicate events. mapNotNull: Filters out null values, emitting only non-null values. mapTo: Converts each event to a specified constant value. throttleTime: Limits the frequency of event emission to a specified duration.
Subjects
Subjects combine the properties of StreamControllers and Observables:
- BehaviorSubject: Caches the latest value and emits it to new subscribers.
- ReplaySubject: Replays all emitted values to new subscribers.
Example: Reading the Konami Code
import 'package:rxdart/rxdart.dart'; void main() { const konamiKeyCodes = [ KeyCode.UP, KeyCode.UP, KeyCode.DOWN, KeyCode.DOWN, KeyCode.LEFT, KeyCode.RIGHT, KeyCode.LEFT, KeyCode.RIGHT, KeyCode.B, KeyCode.A, ]; final result = querySelector('#result')!; document.onKeyUp .map((event) => event.keyCode) .bufferCount(10, 1) // An extension method provided by rxdart .where((lastTenKeyCodes) => const IterableEquality().equals(lastTenKeyCodes, konamiKeyCodes)) .listen((_) => result.innerHtml = 'KONAMI!'); }
Conclusion
RxDart is a valuable library for enhancing Dart's stream handling capabilities. With its additional stream classes, extension methods, and subjects, it enables developers to create more powerful and flexible data processing solutions.