Riverpod: A Reactive Caching and Data-Binding Framework
Published on by Flutter News Hub
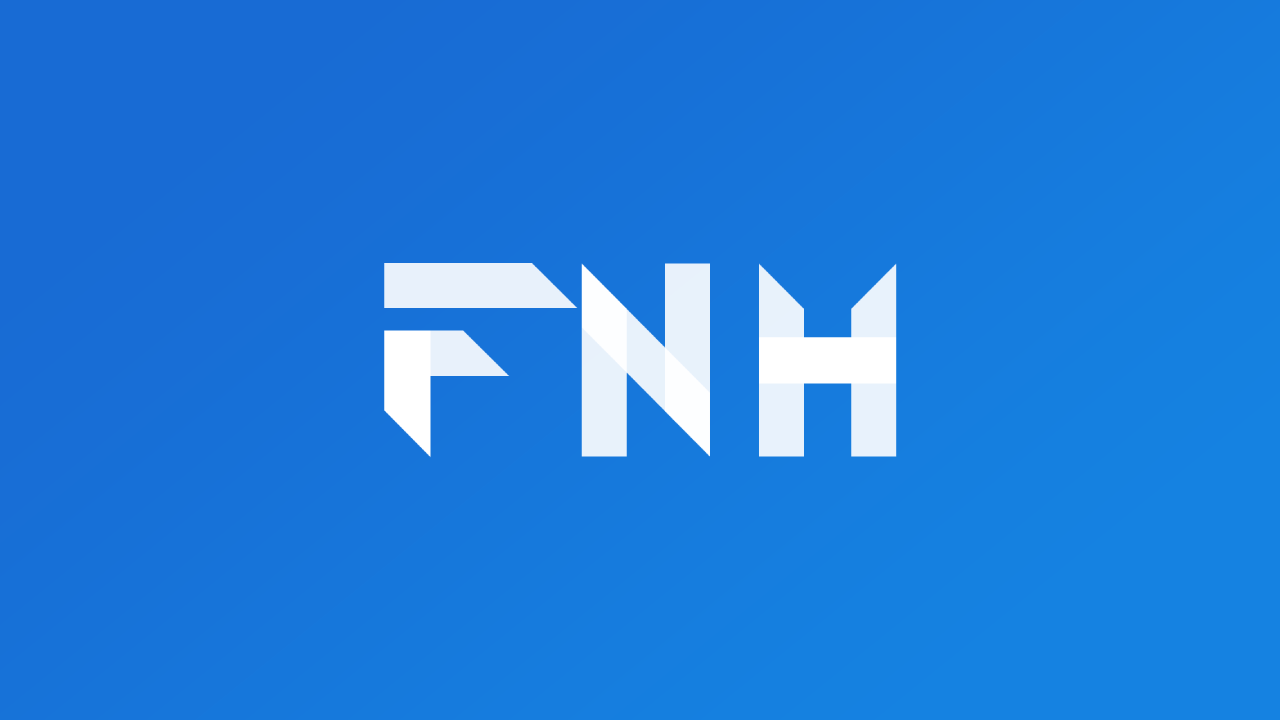
Riverpod is a state management framework for Dart and Flutter that simplifies working with asynchronous code. It was inspired by the Provider package, but it offers several key advantages, including:
- Automatic handling of errors and loading states
- Native support for advanced scenarios, such as pull-to-refresh
- Separation of logic from the UI, ensuring testability and reusability
Key Features
- Automatic error handling: Riverpod automatically catches errors in asynchronous operations and displays error messages to the user. No need to manually handle errors or write try-catch blocks.
- Loading state management: Riverpod tracks the loading state of asynchronous operations. The UI can display a loading indicator while data is being fetched, and then automatically update when the data is available.
- Separation of logic from UI: Riverpod encourages the separation of business logic from the UI. This makes code more testable, maintainable, and reusable.
- Advanced scenario support: Riverpod natively supports advanced scenarios such as pull-to-refresh. This simplifies the implementation of complex UI interactions.
Getting Started
To use Riverpod, add the following dependency to your pubspec.yaml file:
dependencies: riverpod: ^1.0.0
Next, define a provider function to fetch data from a network request:
@riverpod Future fetchUser() async { final response = await http.get(Uri.parse('https://example.com/api/users/1')); return jsonDecode(response.body)['name']; }
In your UI, you can listen to the provider and display the data:
final user = useProvider(fetchUserProvider); return Text(user.when( data: (data) => data, loading: () => 'Loading...', error: (error, stackTrace) => 'Error: $error', ));
Example: Pull-to-Refresh
Riverpod makes it easy to implement pull-to-refresh functionality. Here's how:
- Create a provider that fetches data from the network:
@riverpod Future fetchPosts() async { // ... }
- In your UI, use the RefreshController widget to wrap the content that you want to refresh:
final refreshController = RefreshController(); return RefreshIndicator( onRefresh: () { refreshController.refresh(fetchPosts()); }, child: ListView.builder( // ... ), );
Conclusion
Riverpod is a powerful and easy-to-use state management framework for Dart and Flutter. It simplifies working with asynchronous code and provides several key advantages over traditional approaches. If you're looking for a state management solution, Riverpod is definitely worth checking out.