Revolutionize Flutter Development with Multi App Viewer: A Comprehensive Exploration
Published on by Flutter News Hub
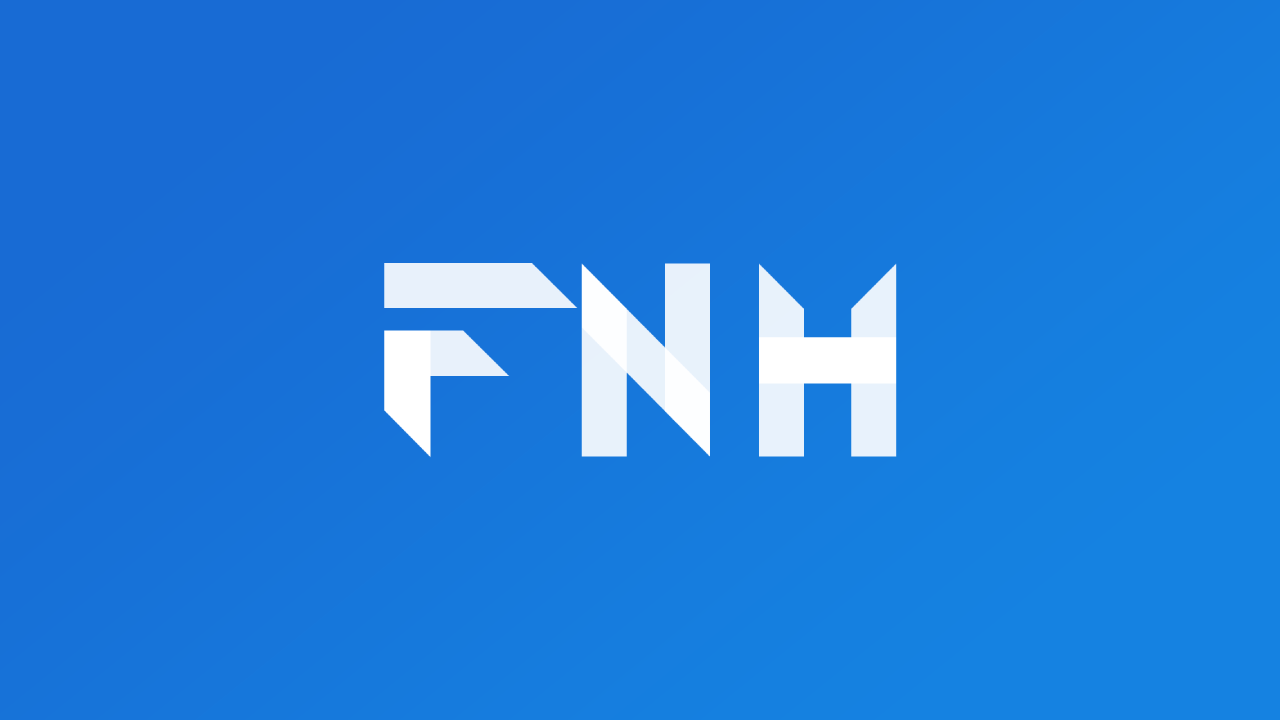
Multi App Viewer (MAV) is an indispensable developer tool that empowers you to simultaneously view and navigate multiple instances of the same Flutter app with diverse visual settings. This cutting-edge tool transforms the app development process, enabling you to:
- Effortlessly compare designs and select the most suitable one.
- Rapidly create professional screenshots and animations.
- Showcase your app's adaptability and responsiveness.
- Save time and streamline navigation by synchronizing multiple app instances.
Getting Started with MAV
To integrate MAV into your Flutter project, follow these steps:
- Add the MAV dependency to your
pubspec.yaml
file. - Copy the
example/lib/main.dart
file into your project with a new name. - Replace
YourApp()
with your app's top-level widget. - Create a new run configuration targeting the modified file and run or debug it.
Code Example:
void main() {
runApp(const MavCounterDemo());
}
class MavCounterDemo extends StatelessWidget {
const MavCounterDemo();
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'MAV Demo',
debugShowCheckedModeBanner: false,
home: MavFrameWidget(
itemBuilder: (MavItem mavItem) {
// Replace MyApp() with your app widget.
return YourApp();
},
),
);
}
}
Customizing Child Instances
To customize individual app instances, use the mavItem
parameter in your root widget:
class MyApp extends StatelessWidget {
final MavItem? mavItem;
MyApp();
}
Configure your instances using mavItem
and its configuration
fields, or add your own logic based on item index.
Code Example for Custom Navigation:
MaterialApp.router(
builder: (context, rootWidget) {
// Use mavItem and configuration to customize navigation.
return Directionality(
textDirection: item?.configuration.textDirection ?? TextDirection.ltr,
child: Theme(
data: Theme.of(context).copyWith(
platform: mavItem?.configuration.targetPlatform,
),
child: rootWidget!,
),
);
},
// ...
);
Synchronized Navigation and Event Handling
MAV enables synchronized navigation by adding a NavigationObserver
and an onNavigationCallback
:
late final GoRouter _router = GoRouter(
// MAV customization.
navigatorKey: mavItem?.navigatorKey,
observers: mavItem?.navigatorObserver == null ? null : [mavItem!.navigatorObserver],
// ...
);
To listen for navigation events and forward them to other instances, add an onNavigationCallback
to the app router.
MaterialApp.router(
builder: (context, rootWidget) {
item?.onNavigationCallback = (String routeName, int index, ) {
if (index != mavItem?.index) {
// Code to forward navigation.
}
};
// ...
},
);
Scripting and Automation
MAV supports scripting for automated actions. Create a list of commands that include navigation, configuration changes, and synchronization commands.
List script = [
// Navigation and configuration changes.
];
// Run the script on toolbar invocation.
MavFrame.buttonScript = script;
// Run the script automatically on app load.
MavFrame.autoRunScript = script;
// Repeat the script indefinitely.
MavFrame.autoRepeat = true;
Additional Considerations
- MAV is a development tool; do not include it in release versions.
- Non-reentrant apps may experience conflicts when running multiple instances.
- Performance may decrease with increasing number of instances.
- Ensure the screen size is adequate for multiple app instances.
Conclusion
Multi App Viewer empowers Flutter developers with unparalleled efficiency and flexibility. Its intuitive interface, customizable settings, and advanced features make it an indispensable tool for rapid iteration, design comparison, and app showcasing. Embrace the power of MAV to transform your Flutter development workflow and unlock the full potential of your apps.