Retry: A Dart Package for Effortless Asynchronous Function Retries
Published on by Flutter News Hub
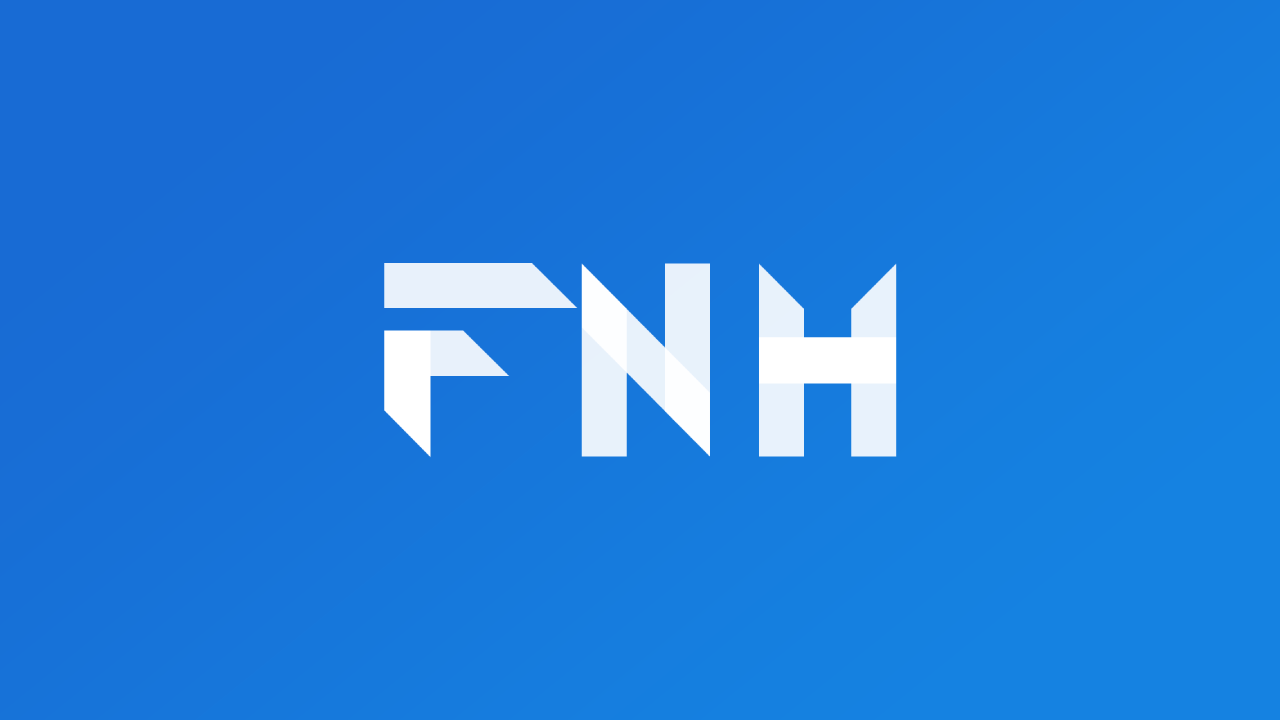
Introducing Retry, a Dart package that simplifies retrying asynchronous functions. This package empowers you to avoid crashing due to intermittent errors, such as unstable network connections or overloaded servers.
Getting Started with retry
Begin by integrating the retry function into your code for straightforward retry logic with exponential back-off:
import 'package:retry/retry.dart'; final response = await retry( () => http.get('https://google.com').timeout(Duration(seconds: 5)), retryIf: (e) => e is SocketException || e is TimeoutException, ); print(response.body);
By default, the retry function attempts the operation eight times, applying exponential sleep intervals after each attempt to prevent overwhelming the server.
Customizing with RetryOptions
For more control over retry behavior, utilize RetryOptions:
final r = RetryOptions(maxAttempts: 8); final response = await r.retry( () => http.get('https://google.com').timeout(Duration(seconds: 5)), retryIf: (e) => e is SocketException || e is TimeoutException, ); print(response.body);
Use Cases for Retry
- Retrying API calls to mitigate intermittent network issues.
- Recovering from database connection drops.
- Resolving issues with message queues or event streams.
- Enhancing the robustness of web service clients.
Conclusion
By leveraging Retry, you can enhance the resilience of your Dart applications, ensuring they gracefully handle temporary setbacks and continue delivering seamless functionality.