Retrofit.dart: A Code Generation Library for Type-Safe and Efficient HTTP Requests in Dart
Published on by Flutter News Hub
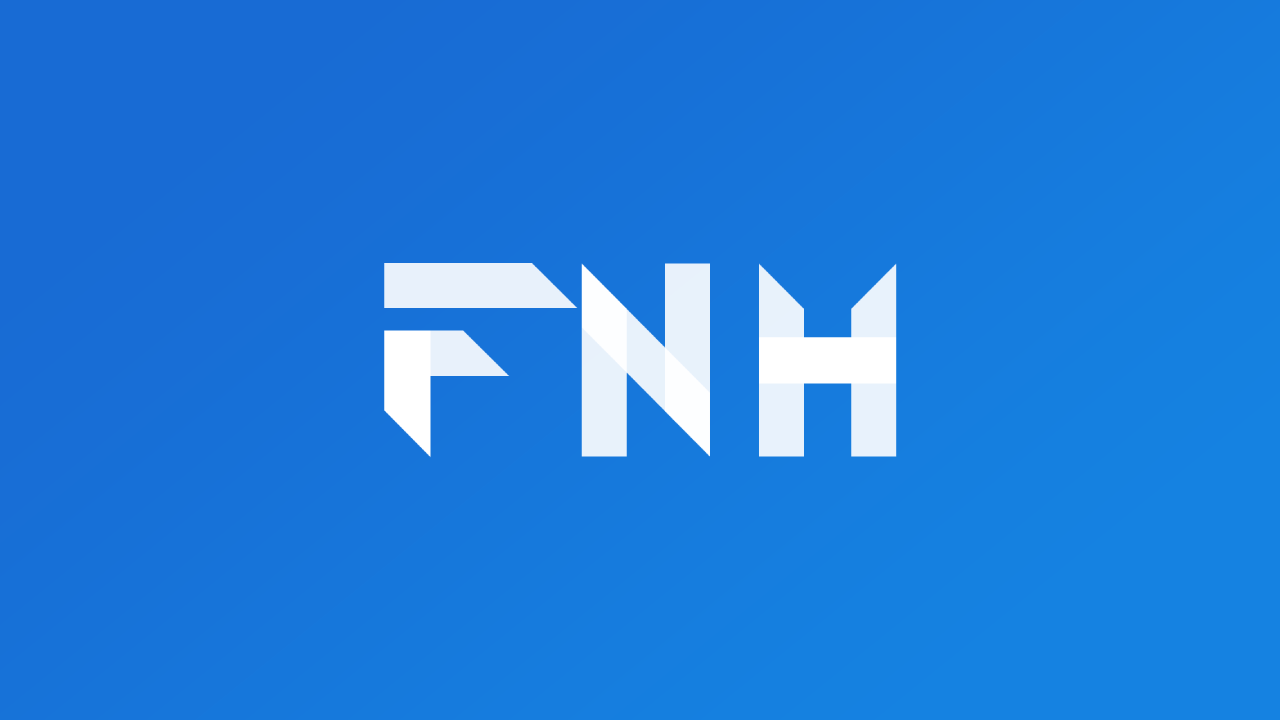
Retrofit.dart is a code generation library inspired by Retrofit and Chopper, specifically designed to simplify and enhance HTTP request handling in Dart projects. It leverages the capabilities of source_gen to generate type-safe and efficient APIs, empowering developers to interact with remote servers with ease.
Getting Started
1. Add Generator Dependency:
dependencies: retrofit: '>=4.0.0 =7.0.0 =2.3.0
2. Define Your API Interface:
@RestApi(baseUrl: 'https://example.com/api/') abstract class MyApiClient { factory MyApiClient(Dio dio) = _MyApiClient; @GET('/users') Future getUsers(); @POST('/users') Future createUser(@Body() User user); }
3. Generate API Implementation:
flutter pub run build_runner build
4. Use the Generated Client:
final dio = Dio(); final client = MyApiClient(dio); client.getUsers().then((users) => print(users));
Key Features
Type Conversion
Retrofit.dart seamlessly handles data conversion between HTTP responses and Dart objects. Simply annotate your model classes with @JsonSerializable and provide a fromJson factory method.
@JsonSerializable() class User { final String id; final String name; final String email; User(this.id, this.name, this.email); factory User.fromJson(Map json) => _$UserFromJson(json); }
HTTP Method Support
Retrofit.dart supports various HTTP methods, including GET, POST, PUT, PATCH, and DELETE. These methods are easily invoked using corresponding annotations.
Query Parameters and Path Variables
You can effortlessly pass query parameters and path variables to your API requests.
@GET('/users/{id}') Future getUser(@Path('id') String id); @GET('/users') Future getUsers(@Query('age') int age, @Query('name') String name);
HTTP Headers
Custom HTTP headers can be added to requests using the @Header annotation.
@GET('/users') Future getUsers(@Header('Authorization') String token);
Error Handling
Retrofit.dart provides a clean and consistent way to handle errors using the catchError method. The caught error object contains detailed information about the failed response.
client.getUsers().catchError((error) { print('Error: ${error.message}'); });
Multiple Endpoints
Retrofit.dart allows you to specify multiple API endpoints within a single RestApi annotation. You can then switch between endpoints based on your needs.
@RestApi(baseUrl: 'https://example.com/api/') abstract class MyApiClient { factory MyApiClient(Dio dio) = _MyApiClient; @GET('/users') Future getUsers(); @RestApi(baseUrl: 'https://example.com/api/v2/') factory MyApiClientV2(Dio dio) = _MyApiClientV2; @GET('/users') Future getUsersV2(); }
Multithreading (Flutter Only)
For Flutter applications, Retrofit.dart offers an optional multithreading feature using the Compute function. This can improve performance by offloading data parsing to a separate thread.
@RestApi(parser: Parser.FlutterCompute) abstract class MyApiClient { ... }
Conclusion
Retrofit.dart empowers Dart developers with a powerful and versatile tool for building efficient and type-safe HTTP clients. Its intuitive syntax and comprehensive features make it an indispensable companion for any project that requires seamless interaction with remote servers.