QR Code Scanning with Flutter: A Comprehensive Guide
Published on by Flutter News Hub
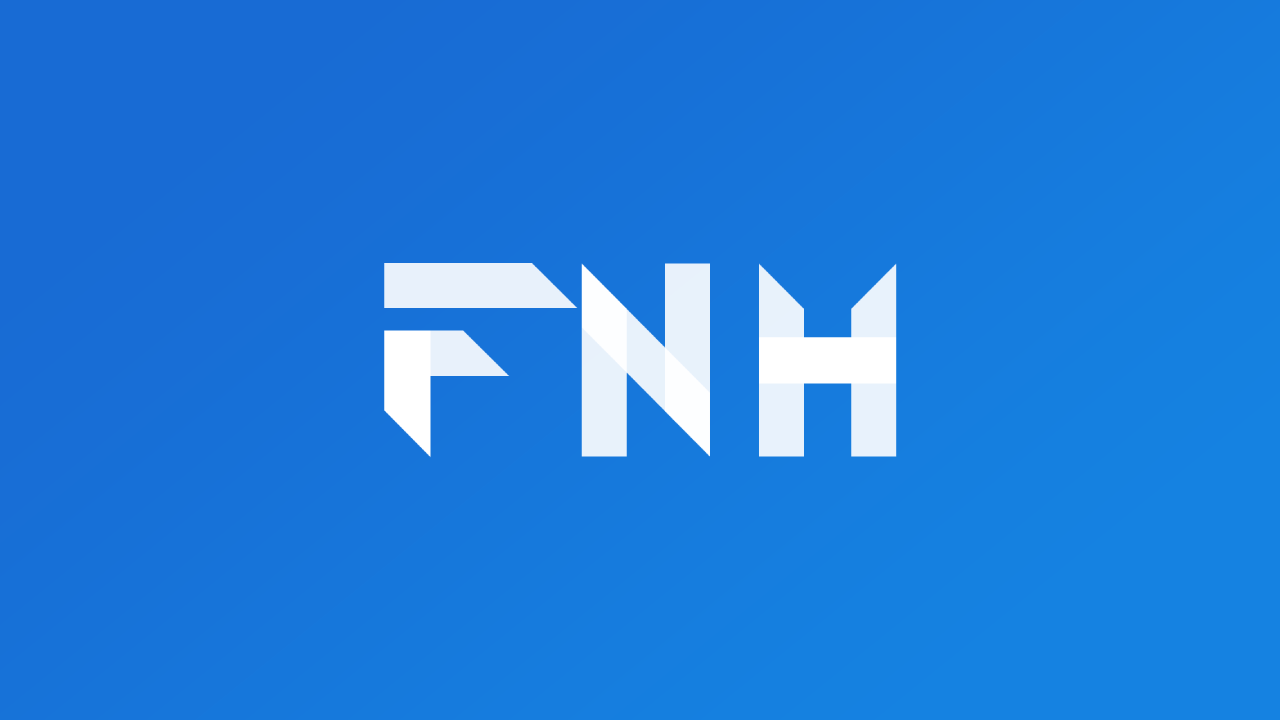
QR code scanning is a ubiquitous need in today's mobile applications. The QR Code Scanner plugin for Flutter provides a seamless and reliable solution for integrating QR code scanning capabilities into your Flutter apps.
Features
- Native integration for Android and iOS
- Real-time QR code scanning
- Customizable settings (flash, camera flip, pause/resume)
- Support for various barcode formats
Android Integration
- Update Gradle, Kotlin, and Kotlin Gradle Plugin versions in your android/build.gradle file.
- Change minSdkVersion to 20 in your android/app/build.gradle file.
iOS Integration
- Add the following to your Info.plist file:
io.flutter.embedded_views_preview NSCameraUsageDescription This app needs camera access to scan QR codes
Usage
- Create a QRView widget:
import 'package:qr_code_scanner/qr_code_scanner.dart'; final GlobalKey qrKey = GlobalKey(debugLabel: 'QR'); QRViewController? controller; class QRViewExampleState extends State { Barcode? result; @override Widget build(BuildContext context) { return Scaffold( body: Column( children: [ Expanded( flex: 5, child: QRView( key: qrKey, onQRViewCreated: _onQRViewCreated, ), ), Expanded( flex: 1, child: Center( child: (result != null) ? Text('Barcode Type: ${result.format}\nData: ${result.code}') : Text('Scan a code'), ), ), ], ), ); } void _onQRViewCreated(QRViewController controller) { this.controller = controller; controller.scannedDataStream.listen((scanData) { setState(() { result = scanData; }); }); } @override void dispose() { controller?.dispose(); super.dispose(); } }
- Listen for scanned QR codes:
controller.scannedDataStream.listen((scanData) { // Do something with the scanned data });
Customization
- Flip between front and back cameras:
await controller.flipCamera();
- Turn flash on or off:
await controller.toggleFlash();
- Pause or resume camera stream and scanner:
await controller.pauseCamera(); await controller.resumeCamera();