QR Code Generation with QR.Flutter for Flutter
Published on by Flutter News Hub
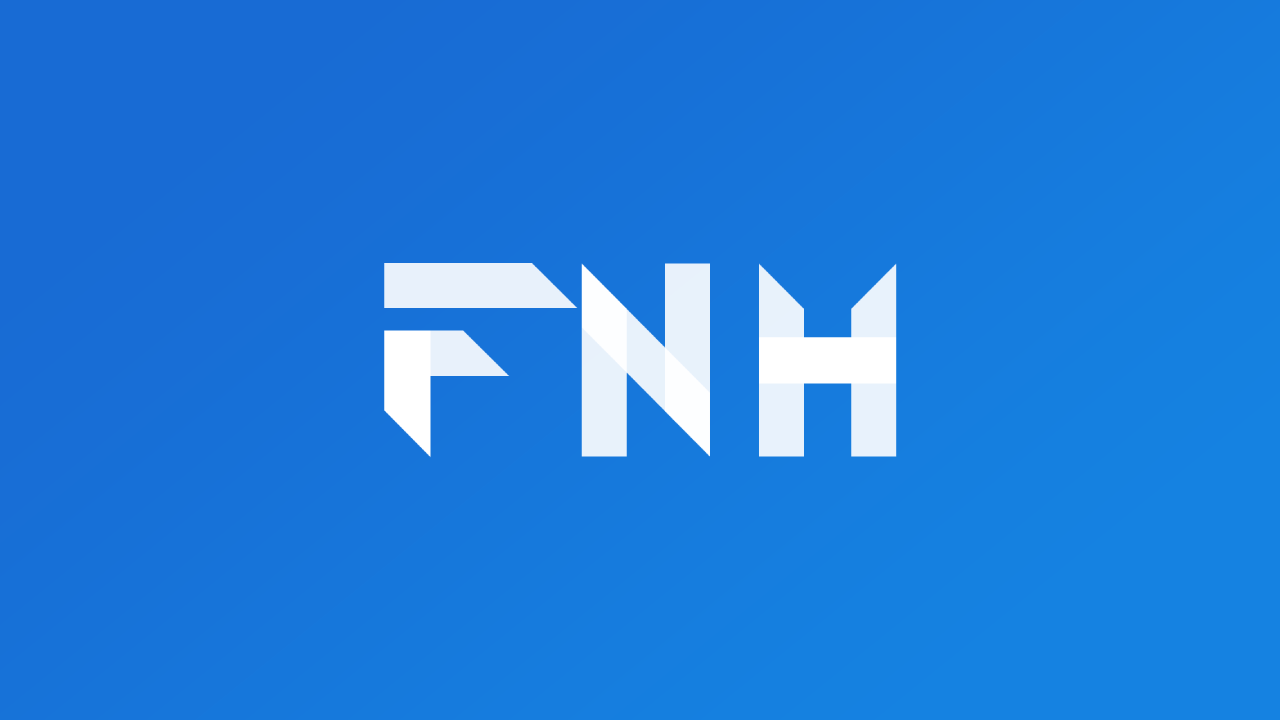
QR Flutter is a Flutter library that enables effortless and swift QR code rendering as a widget or through a custom painter.
Installation
Add the following dependency to your pubspec.yaml file:
dependencies: qr_flutter: ^4.1.0
Getting Started
Import the dependency into your code:
import 'package:qr_flutter/qr_flutter.dart';
To render a basic QR code:
QrImageView( data: '1234567890', version: QrVersions.auto, size: 200.0, );
Configuration Options
QR Flutter provides various configuration options to customize the QR code appearance and behavior:
Property Type Description
| version | int | QR code version (1-40) or QrVersions.auto for automatic detection.
| errorCorrectionLevel | int | Error correction level.
| size | double | QR code size in pixels.
| padding | EdgeInsets | Padding around the QR code.
| backgroundColor | Color | Background color.
| eyeStyle | QrEyeStyle | QR code eyes' shape and color.
| dataModuleStyle | QrDataModuleStyle | Data modules' shape and color.
| gapless | bool | Prevent gaps between modules (default: true).
| errorStateBuilder | QrErrorBuilder | Widget to display in case of an error.
| constrainErrorBounds | bool | Constrain error widget to QR code bounds.
| embeddedImage | ImageProvider | Image to overlay in the QR code center.
| embeddedImageStyle | QrEmbeddedImageStyle | Embedded image styling options.
| embeddedImageEmitsError | bool | Display error if embedded image fails to load.
| semanticsLabel | String | Accessibility label for the QR code.
Examples
Basic QR Code:
QrImageView( data: 'This is a simple QR code', version: QrVersions.auto, size: 320, gapless: false, );
QR Code with Embedded Image:
QrImageView( data: 'This QR code has an embedded image as well', version: QrVersions.auto, size: 320, gapless: false, embeddedImage: AssetImage('assets/images/my_embedded_image.png'), embeddedImageStyle: QrEmbeddedImageStyle( size: Size(80, 80), ), );
QR Code with Error State:
QrImageView( data: 'This QR code will show the error state instead', version: 1, size: 320, gapless: false, errorStateBuilder: (cxt, err) { return Container( child: Center( child: Text( 'Uh oh! Something went wrong...', textAlign: TextAlign.center, ), ), ); }, );
Conclusion
QR.Flutter provides a robust and user-friendly way to generate QR codes in Flutter applications, empowering developers to encode vital information through visually scannable images.