Printing Documents with Flutter: A Comprehensive Guide
Published on by Flutter News Hub
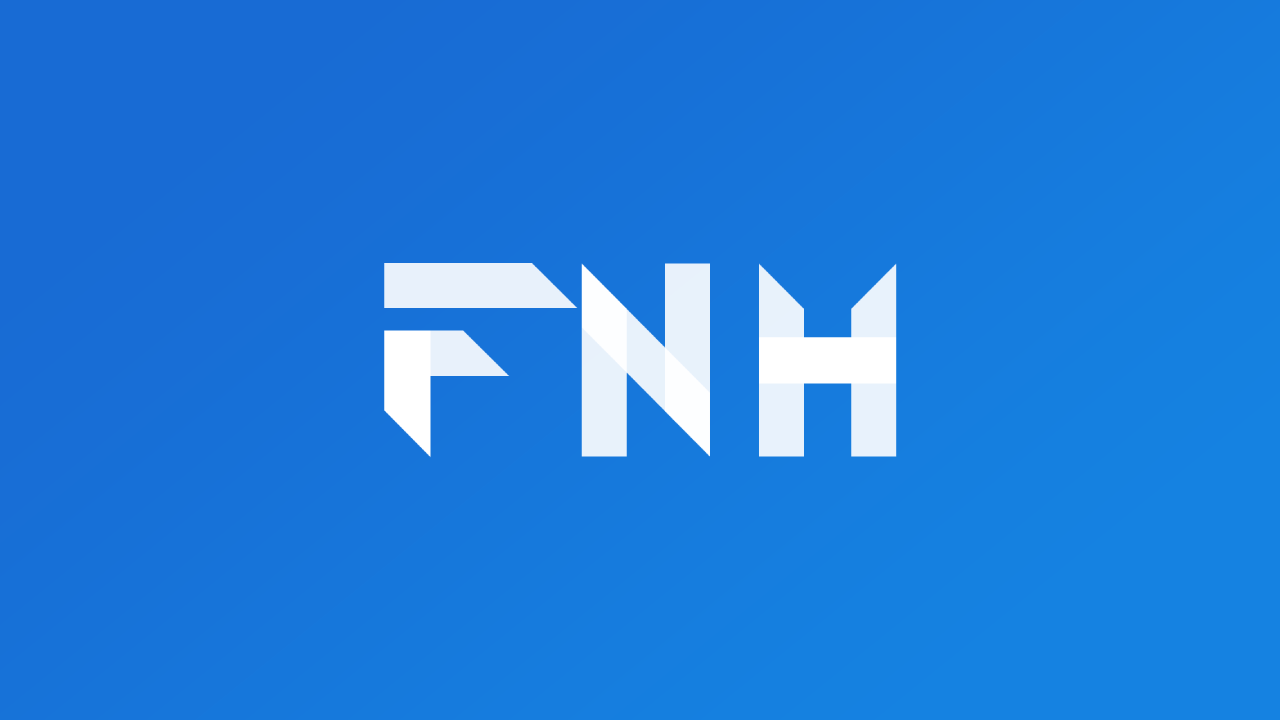
In the realm of mobile development, printing documents remains a crucial task. With the advent of Flutter, developers now have access to a powerful tool that simplifies the process of generating and printing documents on both Android and iOS devices.
This article delves into the world of printing with Flutter, providing a detailed overview of the printing plugin and its capabilities. We will explore everything from installation to document design and explore advanced features such as encryption and digital signatures.
Getting Started with the Printing Plugin
To embark on your printing journey with Flutter, the first step is to install the printing plugin. This can be achieved by adding the following line to your project's pubspec.yaml file:
dependencies: printing: ^[version]
Once the plugin is installed, you can begin incorporating its functionality into your Flutter app. Let's dive into some code examples to demonstrate its usage:
Generating and Saving a PDF Document
Creating a PDF document in Flutter is straightforward with the combination of the pdf and pdf/widgets libraries. Consider the following example:
import 'package:pdf/pdf.dart'; import 'package:pdf/widgets.dart'; void main() { final doc = Document(); doc.addPage(Page( build: (Context context) { return Center( child: Text('Hello World'), ); }, )); doc.save(); }
In this example, we create a Document object, add a Page, and populate it with a simple Text widget. Finally, we instruct the document to save itself as a PDF file.
Printing a PDF Document
With your document ready, you can leverage the Printing plugin to print it. The following code snippet showcases how:
await Printing.layoutPdf( onLayout: (PdfPageFormat format) async => doc.save(), );
Alternatively, you can share the document with other applications using the sharePdf method:
await Printing.sharePdf( bytes: await doc.save(), filename: 'my-document.pdf', );
Beyond Printing: Advanced Features
The Printing plugin empowers you with a range of advanced features, including:
Encryption: Protect your sensitive documents using industry-standard encryption algorithms such as RC4-40, RC4-128, AES-128, and AES-256.
Digital Signature: Digitally sign your PDF documents to ensure authenticity and prevent tampering. The plugin supports SHA1 and SHA-256 signatures.
PDF Parsing and Manipulation: Load existing PDF documents, manipulate their pages, and add signatures using the built-in PDF parser.
Designing Your PDF Documents
Designing visually appealing and informative PDF documents is essential. PdfPreview, a Flutter widget bundled with the Printing plugin, provides an interactive canvas for designing your documents. It offers hot-reload functionality, allowing you to preview your designs in real-time.
Conclusion
The Printing plugin for Flutter empowers developers with a comprehensive set of tools for printing and managing documents on mobile devices. From generating PDF documents to printing and sharing them, this plugin simplifies the entire process, making it a must-have for any mobile application that requires document handling capabilities.