Persistent Data Storage with Shared Preferences Plugin for Flutter Apps
Published on by Flutter News Hub
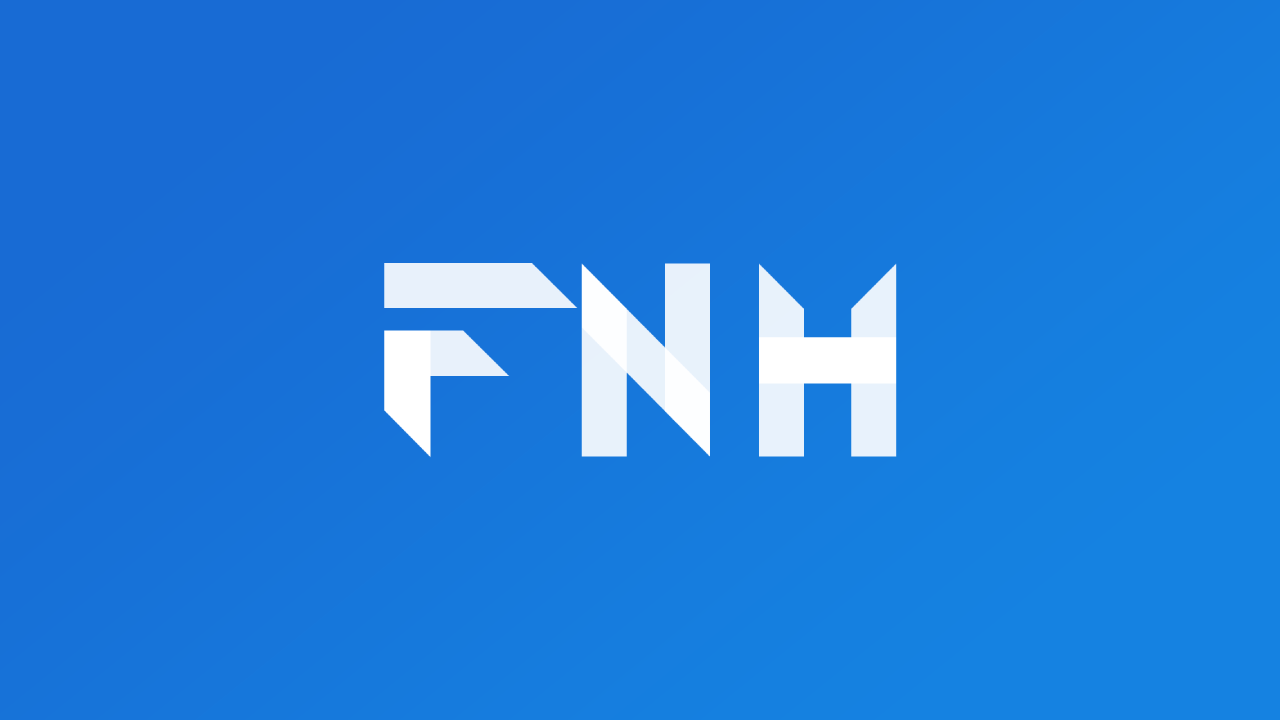
The Shared Preferences plugin allows Flutter developers to easily store and retrieve simple data persistently on the user's device. This data can include values such as user preferences, settings, or any other information that needs to persist across app restarts.
Supported Data Types
The Shared Preferences plugin supports the following data types:
- int
- double
- bool
- String
- List<String>
Usage
To use the Shared Preferences plugin, you first need to add it to your pubspec.yaml file:
dependencies: shared_preferences: ^2.0.13
Once added, you can import the plugin into your Dart code:
import 'package:shared_preferences/shared_preferences.dart';
To obtain an instance of the Shared Preferences object, use SharedPreferences.getInstance():
final SharedPreferences prefs = await SharedPreferences.getInstance();
Writing Data
To write data to Shared Preferences, use the appropriate set* method for the desired data type:
await prefs.setInt('counter', 10); await prefs.setBool('repeat', true); await prefs.setDouble('decimal', 1.5); await prefs.setString('action', 'Start'); await prefs.setStringList('items', ['Earth', 'Moon', 'Sun']);
Reading Data
To read data from Shared Preferences, use the corresponding get* method for the expected data type. If the key does not exist, it returns null.
final int? counter = prefs.getInt('counter'); final bool? repeat = prefs.getBool('repeat'); final double? decimal = prefs.getDouble('decimal'); final String? action = prefs.getString('action'); final List? items = prefs.getStringList('items');
Removing Data
To remove a specific key from Shared Preferences, use the remove method:
await prefs.remove('counter');
Multiple Instances and Caching
Shared Preferences maintains a cache on the Dart side to optimize performance. This can lead to inconsistencies if you're using SharedPreferences from multiple isolates, engine instances, or if you're modifying the preference store externally.
To address this, you can call reload() on the instance before reading from it to update the cache:
prefs.reload();
Migration and Prefixes
By default, SharedPreferences only reads and writes preferences with the prefix flutter.. You can change this prefix by calling setPrefix before creating any instances of SharedPreferences:
SharedPreferences.setPrefix('my_app.');
This allows you to isolate your preferences from other apps or versions of your app.
Testing
For testing purposes, you can use a mock implementation of SharedPreferences:
final Map values = {'counter': 1}; SharedPreferences.setMockInitialValues(values);
Storage Location by Platform
The table below shows the storage location of Shared Preferences on each platform:
Platform Location
| Android | SharedPreferences
| iOS | NSUserDefaults
| Linux | XDG_DATA_HOME directory
| macOS | NSUserDefaults
| Web | LocalStorage
| Windows | Roaming AppData directory
Conclusion
The Shared Preferences plugin provides a simple and efficient way to store and retrieve persistent data in Flutter apps. It supports a range of data types and offers features like caching and prefixing to enhance flexibility and performance.