Persistent Bottom Navigation Bar for Flutter
Published on by Flutter News Hub
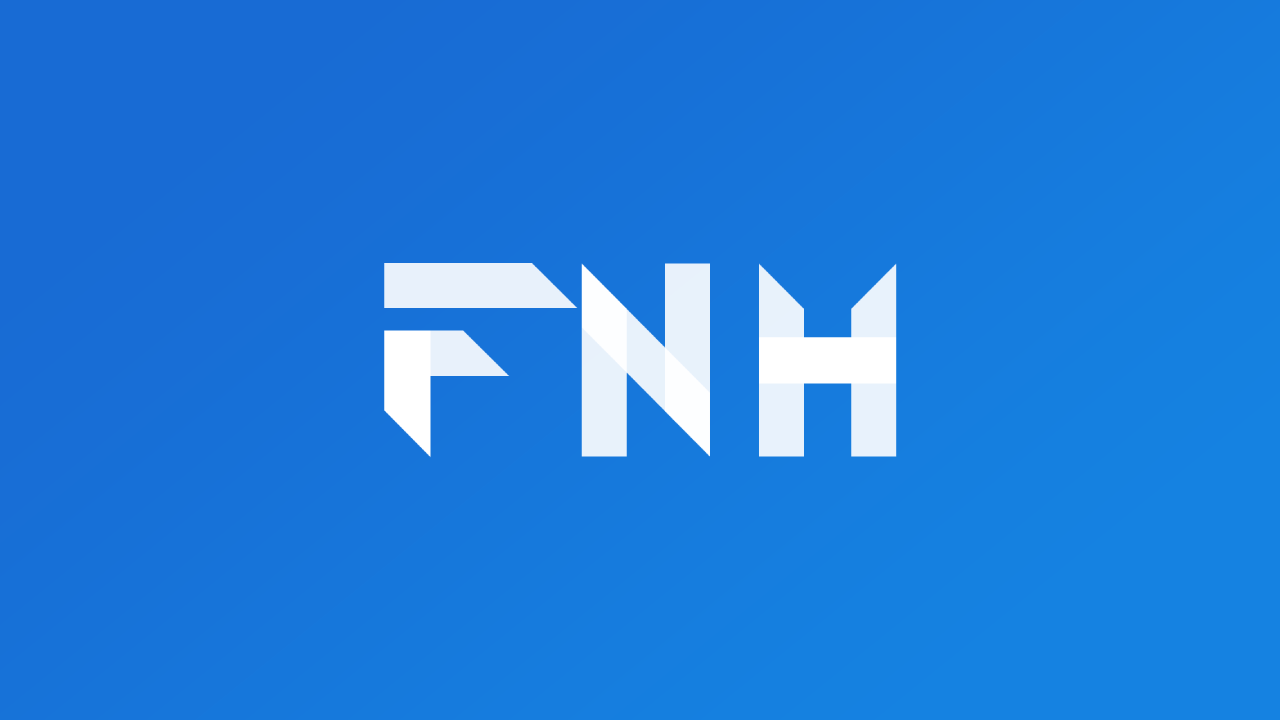
Enhance your Flutter app's navigation experience with the Persistent Bottom Navigation Bar package. This customizable package provides a static bottom navigation bar, allowing users to easily switch between screens.
Features
- Highly customizable, with 20+ predefined styles and options to tailor to your app's design.
- Seamless screen transitions, with the ability to push new screens with or without the navigation bar visible.
- Handles hardware and software back button presses, ensuring smooth navigation on both Android and iOS.
Installation
Add the dependency to your Flutter project's pubspec.yaml file:
dependencies: persistent_bottom_nav_bar: any
Import the package into your Dart code:
import 'package:persistent_bottom_nav_bar/persistent_tab_view.dart';
Usage
Create a PersistentTabController to manage the navigation state:
PersistentTabController _controller = PersistentTabController(initialIndex: 0);
Declare the PersistentTabView as your main widget:
PersistentTabView( context, controller: _controller, screens: _buildScreens(), items: _navBarsItems(), confineInSafeArea: true, backgroundColor: Colors.white, )
Custom Navigation Bar Styling
For a unique navigation bar design, create a custom widget:
class CustomNavBarWidget extends StatelessWidget { final int selectedIndex; final List items; final ValueChanged onItemSelected; CustomNavBarWidget({ this.selectedIndex, this.items, this.onItemSelected, }); @override Widget build(BuildContext context) { return Container( width: double.infinity, height: 60.0, child: Row( children: items.map((item) => _buildItem(item, selectedIndex == items.indexOf(item))).toList(), ), ); } Widget _buildItem(PersistentBottomNavBarItem item, bool isSelected) { return GestureDetector( onTap: () => onItemSelected(items.indexOf(item)), child: Column( children: [ IconTheme( data: IconThemeData( size: 26.0, color: isSelected ? item.activeColorPrimary : item.inactiveColorPrimary, ), child: item.icon, ), Text( item.title, style: TextStyle( color: isSelected ? item.activeColorPrimary : item.inactiveColorPrimary, fontWeight: FontWeight.w400, fontSize: 12.0, ), ), ], ), ); } }
Integrate the custom widget into PersistentTabView:
PersistentTabView.custom( context, controller: _controller, itemCount: items.length, customWidget: CustomNavBarWidget( items: _navBarsItems(), selectedIndex: _controller.index, onItemSelected: (index) => setState(() => _controller.index = index), ), )
Screen Navigation Functions
Push screens with the following functions, controlling navigation bar visibility:
PersistentNavBarNavigator.pushNewScreen( context, screen: MainScreen(), withNavBar: true, pageTransitionAnimation: PageTransitionAnimation.cupertino, );
PersistentNavBarNavigator.pushDynamicScreen( context, screen: HomeModalScreen(), withNavBar: true, );
Conclusion
The Persistent Bottom Navigation Bar is a versatile solution for creating intuitive and visually appealing navigation in Flutter apps. Its customizable options and navigation functions make it a powerful tool for enhancing the user experience.