ObjectBox: A High-Performance Flutter Database for Dart Objects
Published on by Flutter News Hub
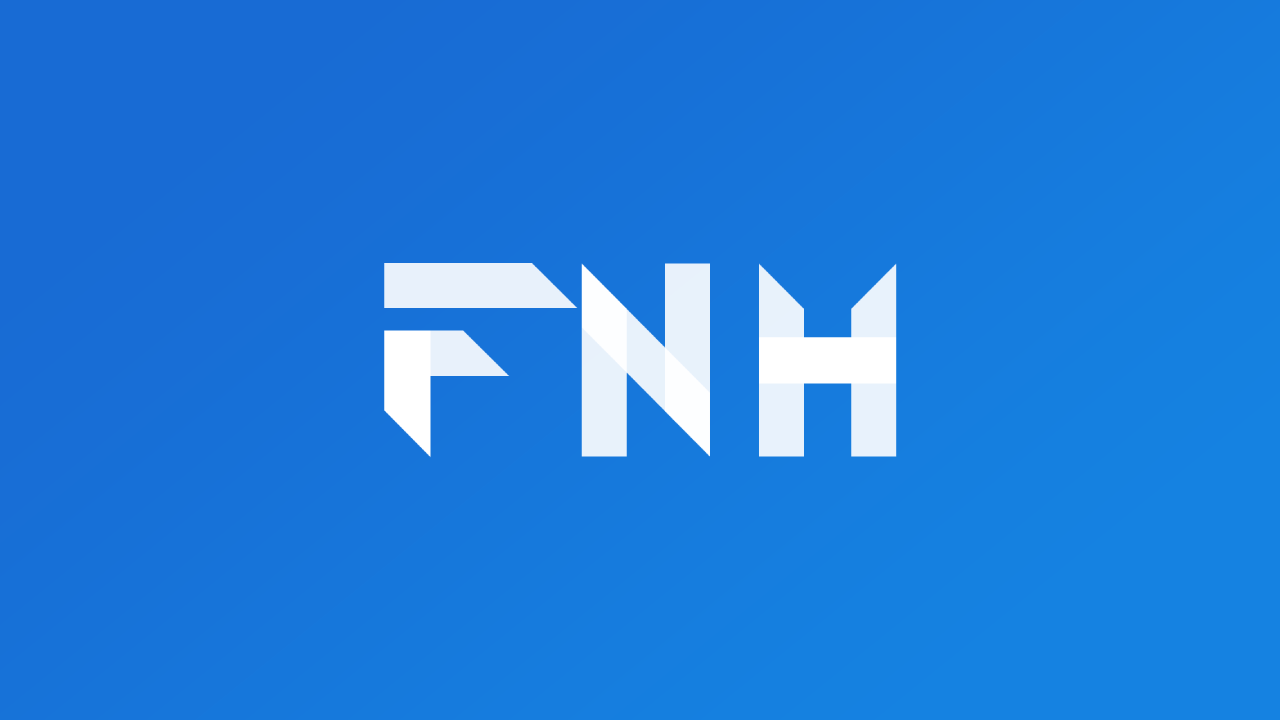
ObjectBox is a powerful database solution that provides unmatched performance and efficiency for storing Dart objects in your Flutter applications. With its ACID compliance and cross-platform capabilities, ObjectBox empowers developers to build robust and scalable mobile and IoT apps.
Key Features
- Blazingly Fast: 10x faster than SQLite, delivering unparalleled performance for demanding applications.
// Simple example showing the speed of ObjectBox final store = await openStore(); final box = store.box(); final person = Person(name: "John Doe"); final id = box.put(person); final retrievedPerson = box.get(id)!; // Retrieve the object with lightning speed
- ACID Compliant: Ensures data integrity and reliability with support for Atomicity, Consistency, Isolation, and Durability.
- Cross-Platform: Build once, deploy anywhere on Android, iOS, macOS, Linux, and Windows.
// Open the store across platforms using the same syntax final store = await openStore();
- Scalable: Handles millions of objects with ease, making it ideal for complex data-intensive apps.
- NoSQL Database: Eliminates the need for rows and columns, allowing you to store pure Dart objects directly.
// Define your object model with the @Entity annotation @Entity() class Person { @Id() int id; String name; }
- Built-in Relations: Supports object links and relationships, simplifying data modeling and reducing boilerplate code.
// Define a relationship between Person and Address @Entity() class Address { @Id() int id; String street; int personId; // Foreign key to the Person table }
- Powerful Queries: Filter data efficiently using a comprehensive query API, including support for across-relation queries.
// Query for people with a specific name final query = box.query(Person_.name.equals("John Doe")).build();
- Schema Migration: Seamlessly handle schema changes without data loss, ensuring a smooth transition as your app evolves.
// Add a new field to the Person object @Entity() class Person { @Id() int id; String name; String email; // New field added }
Performance Benchmarks
ObjectBox outperforms popular Flutter database solutions in key operations:
// CRUD (Create, Read, Update, Delete) benchmark results Operation | ObjectBox | SQLite | Hive ---------|----------|--------|------ Create | 0.15 ms | 1.7 ms | 0.8 ms Read | 0.04 ms | 0.4 ms | 0.1 ms Update | 0.14 ms | 1.3 ms | 0.4 ms Delete | 0.14 ms | 1.2 ms | 0.3 ms
Getting Started
To start using ObjectBox in your Flutter app, follow these simple steps:
- Add ObjectBox to your pubspec.yaml:
dependencies: objectbox: ^1.0.0
- Open the ObjectBox store and create a box for your objects:
final store = await openStore(); final box = store.box();
- Store and retrieve objects using the box:
// Create a new person final person = Person(name: "John Doe"); // Save the person to the database final id = box.put(person); // Retrieve the person from the database final retrievedPerson = box.get(id)!;
FAQ
- Why should I use ObjectBox over other database solutions?
ObjectBox offers exceptional performance, cross-platform support, and a user-friendly API designed for Dart objects, making it an ideal choice for demanding Flutter applications.
- How can I improve the performance of my ObjectBox database?
Use indices to speed up queries, batch operations to reduce database interactions, and optimize your object model for efficient data storage.
- Can I use ObjectBox with other ORMs or data frameworks?
ObjectBox can be used as a standalone database solution or integrated with other ORMs or data frameworks to complement their functionality.
Conclusion
ObjectBox provides an exceptional Flutter database experience, empowering developers with the tools to create high-performance, scalable, and reliable apps. With its intuitive API, cross-platform capabilities, and blazing-fast performance, ObjectBox is the perfect choice for storing and managing Dart objects in your mobile and IoT applications.