Navigating with AutoRoute in Flutter
Published on by Flutter News Hub
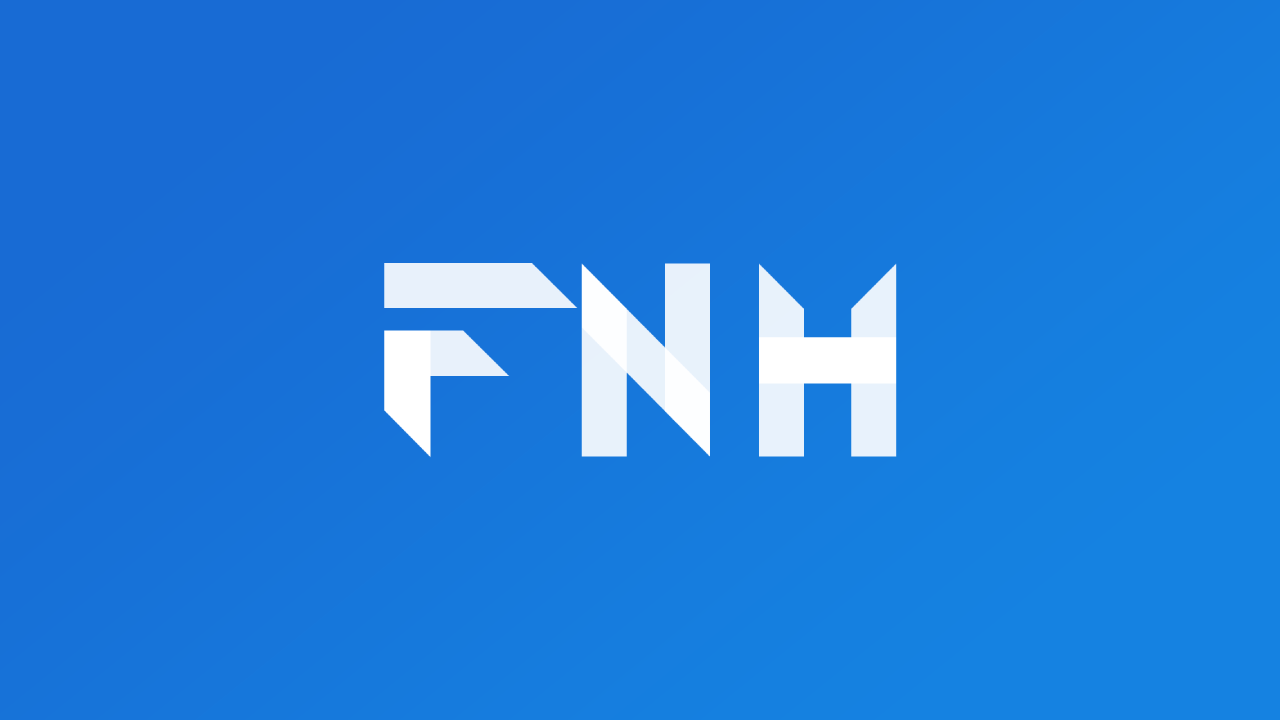
AutoRoute is a powerful navigation library for Flutter that simplifies route setup and provides strongly-typed arguments passing. In this guide, we'll explore how to use AutoRoute for navigating between screens.
Installation
To install AutoRoute, add the following dependencies to your pubspec.yaml file:
dependencies: auto_route: ^latest-version dev_dependencies: auto_route_generator: ^latest-version build_runner:
Setup
To set up AutoRoute, create a router class annotated with @AutoRouterConfig. Override the routes getter and populate it with your routes.
@AutoRouterConfig() class AppRouter extends $AppRouter { @override List get routes => [ AutoRoute(page: HomeScreen.page), AutoRoute(page: ProductDetails.page), ]; }
Navigating Between Screens
To navigate between screens, use the push, pop, and replace methods of the Router object. These methods accept PageRouteInfo objects or path strings.
Example:
// Push a new screen context.router.push(const ProductDetails(id: 1)); // Pop the current screen context.router.pop(); // Replace the current screen context.router.replace(const NewScreen());
Passing Arguments
AutoRoute automatically detects and handles page arguments. Simply define them in the constructor of your page widget.
class ProductDetails extends StatelessWidget { const ProductDetails({required this.id}); final int id; }
Returning Results
To return results from a page, use either the pop completer or pass a callback function as an argument.
Example:
// Using the pop completer final result = await context.router.push(const LoginScreen()); // Using a callback function context.router.push(const EditProfileScreen(onProfileUpdated: (updatedProfile) { // Handle updated profile }));
Nested Navigation
To implement nested navigation, create a nested router inside of a page widget and populate its children field with the child routes.
Example:
class DashboardScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Row( children: [ // ... Expanded( child: AutoRouter(children: [ AutoRoute(path: 'users', page: UsersScreen.page), AutoRoute(path: 'posts', page: PostsScreen.page), ]), ), ], ); } }
Tab Navigation
AutoRoute provides support for tab navigation using the AutoTabsRouter.
class DashboardScreen extends StatelessWidget { @override Widget build(BuildContext context) { return AutoTabsRouter( routes: const [ UsersScreen(), PostsScreen(), ], initialIndex: 0, builder: (context, child) { // Customize the tab bar here return TabLayout(child: child); }, ); } }
Deep Linking
AutoRoute can handle deep links by matching platform links to your routes.
// ... MaterialApp.router( routerConfig: _appRouter.config( deepLinkTransformer: (uri) { // Handle deep links here return SynchronousFuture(uri); }, ), );
Custom Route Transitions
Use CustomRoute to define custom route transitions.
CustomRoute( page: ProfileScreen.page, transitionsBuilder: (context, animation, secondaryAnimation, child) { // Define your custom transition here return FadeTransition(opacity: animation, child: child); }, );
Conclusion
AutoRoute simplifies navigation in Flutter and provides features like strong-typing, automatic argument handling, and support for nested and tab navigation. By following these steps, you can easily implement robust navigation in your Flutter applications.