Mocktail: A Comprehensive Guide for Mocking in Dart
Published on by Flutter News Hub
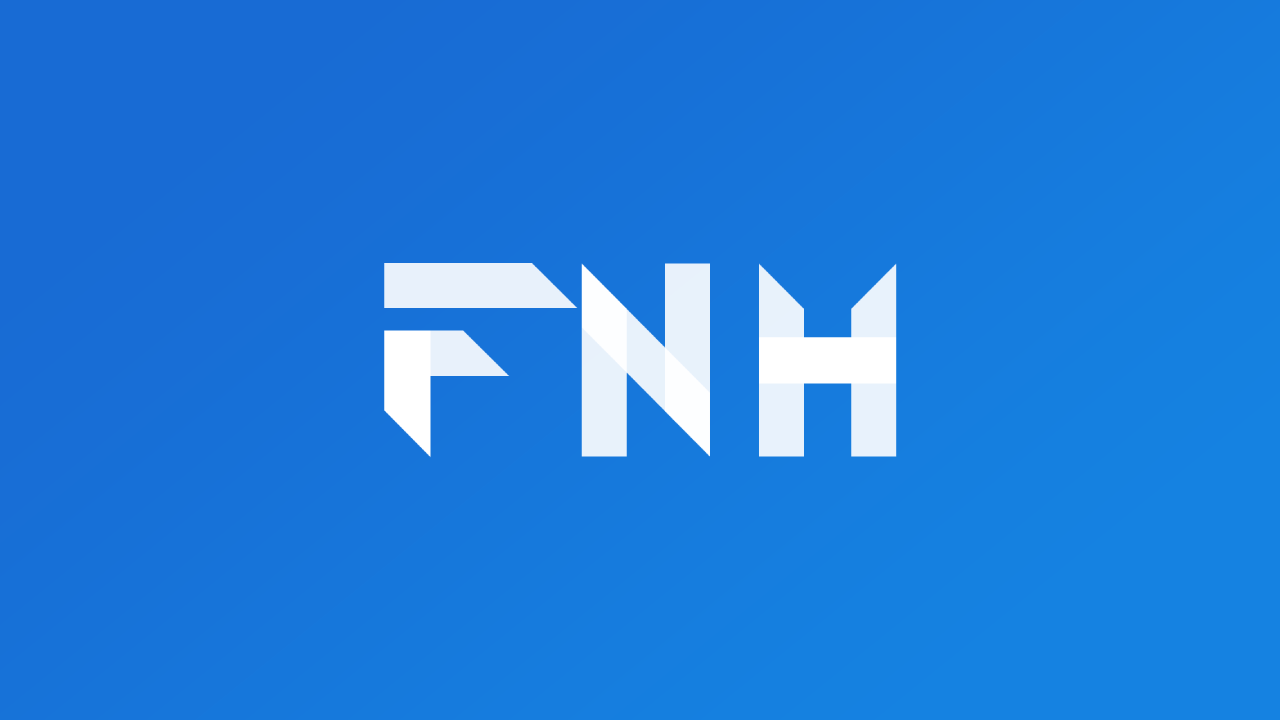
Mocktail is a powerful mocking library for Dart, designed to make testing a breeze. Its intuitive API and extensive features provide a seamless and efficient way to create and interact with mocks.
Creating Mocks
To create a mock, simply extend the Mock class and implement the interface you want to mock. For instance, consider a Cat class:
class Cat { String sound() => 'meow!'; bool likes(String food, {bool isHungry = false}) => false; final int lives = 9; }
To create a mock for Cat, we can use:
class MockCat extends Mock implements Cat {}
Stubbing and Verifying Behavior
Mocking involves stubbing and verifying method behavior. To stub a method, use when() to specify the behavior. For example, to stub sound() to return "meow":
when(() => cat.sound()).thenReturn('meow');
To verify a method has been called, use verify(). To verify sound() was called once:
verify(() => cat.sound()).called(1);
Additional Usage
Mocktail offers a wide range of additional features:
- Stubbing before interaction: Stubbing can be done before interacting with the mock.
- Multiple interactions: You can interact with the mock multiple times.
- Dynamic stubs: Stubs can be calculated dynamically.
- Argument matchers: Use any() to match any argument, or create custom matchers with any(that: matcher).
- Capture arguments: Use captureAny() to capture any argument, or captureAny(that: matcher) to capture specific arguments.
- Resetting mocks: Use reset(mock) to reset stubs and interactions.
Example:
// Stub `likes` for specific arguments when(() => cat.likes('fish', isHungry: false)).thenReturn(true); // Verify `likes` was called with specific arguments verify(() => cat.likes(any(that: startsWith('d')))).called(1);
FAQ
Q: Why am I getting an invalid_implementation_override error when mocking certain classes?
A: For classes like ThemeData, use a mixin to override toString.
Q: Why can't I stub/verify extension methods?
A: Extension methods should be stubbed/verified on the instance they interact with.
Q: Why do I get a TypeError when stubbing using any()?
A: Ensure the type of the method being stubbed is explicitly specified.
Conclusion
Mocktail is a powerful and easy-to-use mocking library for Dart, providing a comprehensive set of features to simplify testing. Its intuitive API and extensive documentation make it an essential tool for any Dart developer.