Mastering Path Manipulation in Dart with the Comprehensive Path Library
Published on by Flutter News Hub
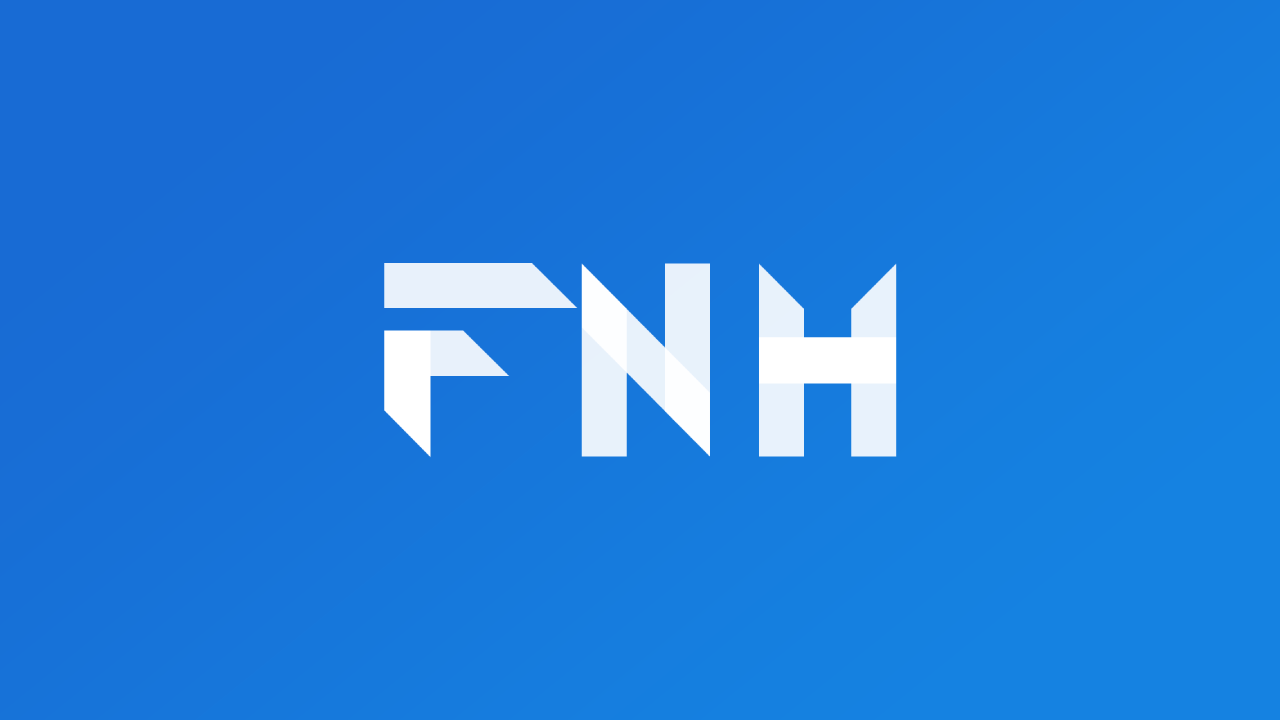
The path library for Dart provides a comprehensive suite of functions and classes for effortlessly manipulating paths, a fundamental task in programming. Whether you're on Linux, Windows, or MacOS, this library ensures seamless cross-platform path handling.
Top-Level Functions: Simplicity and Efficiency
The path library offers an array of top-level functions for common path operations:
import 'package:path/path.dart' as p; // Join path components p.join('directory', 'file.txt'); // Split a path into components p.split('directory/folder/file.txt'); // Get the file extension p.extension('file.txt');
These functions automatically detect and handle platform-specific path separators, making cross-platform development a breeze.
Contextual Path Manipulation: Explicit Path Control
For fine-grained control over path manipulation, you can create a Context object with an explicit path style:
var context = p.Context(style: Style.windows); context.join('directory', 'file.txt');
This guarantees that path operations use the specified style, regardless of the underlying platform.
Stability and Compatibility: A Foundation for Reliability
The path library is committed to stability, ensuring that valid input and output operations remain consistent across versions. However, invalid input or incorrect output handling may be subject to changes as bug fixes and specification updates occur.
FAQ: Addressing Common Questions
Where can I use the path library?
The path library operates on the Dart VM and in browsers, both with dart2js and Dartium. It uses window.location.href as the current path in browsers.
Why not create first-class path objects?
The library treats paths as strings to avoid the complexities and limitations of wrapping them in objects.
How cross-platform is the library?
The path library handles Windows path corner cases, including drive letters, UNC prefixes, and forward/backward slash compatibility.
What constitutes a path in the browser?
In the browser, paths are represented as URL strings, adhering to the WHATWG URL specification.
Code Examples for Clarity
// Normalize path separators on Windows print(p.normalize('dir\\sub\\file.txt')); // Output: 'dir/sub/file.txt' // Resolve relative paths print(p.resolve('dir', 'a.txt', 'b.txt')); // Output: 'dir/b.txt' // Create a URL context var context = p.Context(style: Style.url); print(context.normalize('http://example.com/foo/bar')); // Output: 'http://example.com/foo/bar/' // Check if a path is absolute print(p.isAbsolute('C:\\Users\\example')); // Output: true
Conclusion
The path library serves as an indispensable tool for developers working with Dart. Its comprehensive functionality, cross-platform support, and commitment to stability make it an essential library for path manipulation tasks.