Mastering HTTP Requests with Dart's http Package
Published on by Flutter News Hub
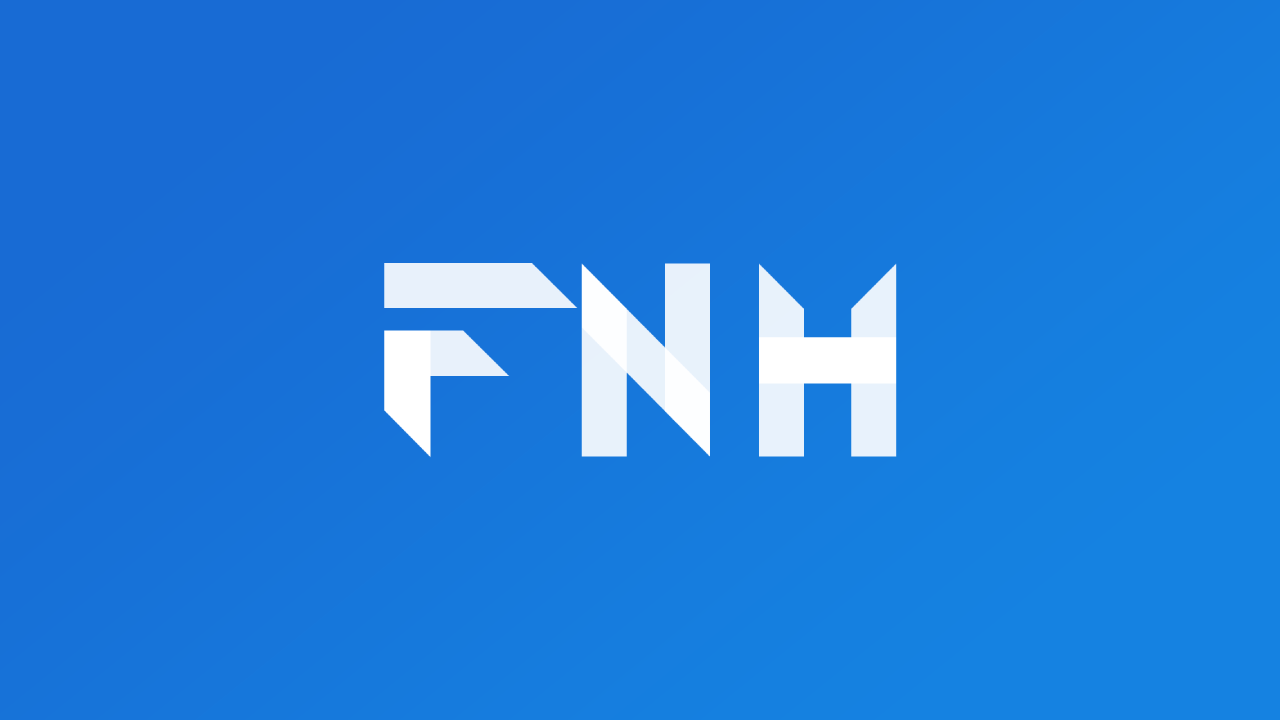
The http package in Dart provides a comprehensive library for making HTTP requests efficiently and effectively. This guide will delve into its features, usage, and configuration, empowering you to effortlessly interact with web resources.
Using Top-Level Functions
For straightforward HTTP requests, top-level functions offer an easy way to get started.
import 'package:http/http.dart' as http; var url = Uri.https('example.com', 'whatsit/create'); var response = await http.post(url, body: {'name': 'doodle', 'color': 'blue'}); print('Response status: ${response.statusCode}'); print('Response body: ${response.body}'); print(await http.read(Uri.https('example.com', 'foobar.txt')));
Using a Client
If you need to make multiple requests to the same server, consider using a Client instance:
var client = http.Client(); try { var response = await client.post( Uri.https('example.com', 'whatsit/create'), body: {'name': 'doodle', 'color': 'blue'}); var decodedResponse = jsonDecode(utf8.decode(response.bodyBytes)) as Map; var uri = Uri.parse(decodedResponse['uri'] as String); print(await client.get(uri)); } finally { client.close(); }
Configuring the HTTP Client
To use a specific HTTP client implementation, you can configure it through the httpClient function:
Client httpClient() { if (Platform.isAndroid) { return CronetClient.defaultCronetEngine(); } if (Platform.isIOS || Platform.isMacOS) { return CupertinoClient.defaultSessionConfiguration(); } return IOClient(); }
Retrying Requests
The retry subpackage provides RetryClient to automatically retry failed requests:
import 'package:http/http.dart' as http; import 'package:http/retry.dart'; Future main() async { final client = RetryClient(http.Client()); try { print(await client.read(Uri.http('example.org', ''))); } finally { client.close(); } }
Summary
The http package empowers Dart developers to efficiently make HTTP requests. Whether using top-level functions or a Client instance, the package simplifies the process of interacting with web resources. Furthermore, its configurability allows for tailoring to specific needs, such as retrying requests or choosing a desired HTTP client implementation.