Manage and Interact with Home Screen Quick Actions in Flutter
Published on by Flutter News Hub
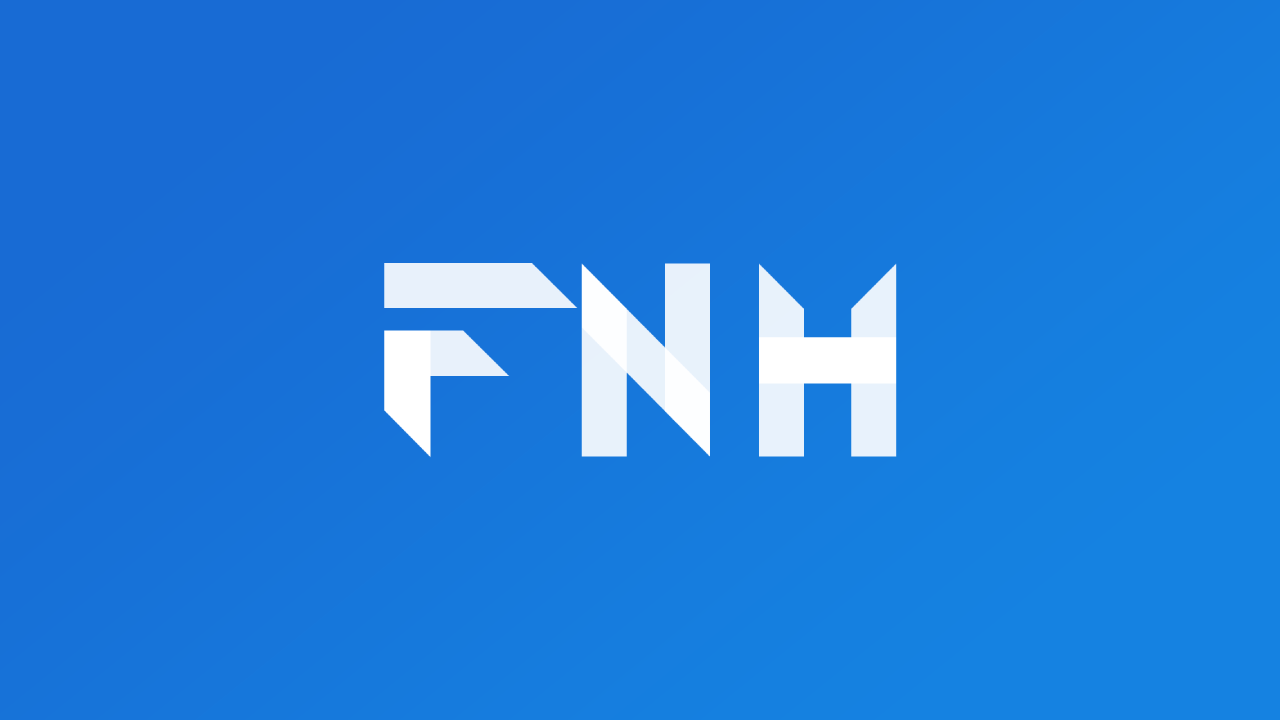
Elevate your Flutter applications with easy management and interaction for home screen quick actions, known as Quick Actions on iOS and App Shortcuts on Android.
Initialization and Configuration
To initiate the library, incorporate the following code snippet early in your app's lifecycle:
final QuickActions quickActions = const QuickActions(); quickActions.initialize((shortcutType) { if (shortcutType == 'action_main') { print('The user tapped on the "Main view" action.'); } // Implement additional handling code... });
This will invoke a callback function when a user launches your app through a quick action.
Managing Quick Actions
To manage quick actions effectively, utilize the setShortcutItems method:
quickActions.setShortcutItems([ const ShortcutItem( type: 'action_main', localizedTitle: 'Main view', icon: 'icon_main', ), const ShortcutItem( type: 'action_help', localizedTitle: 'Help', icon: 'icon_help', ), ]);
Ensure the type value is unique within your application and corresponds to the icon resource name (e.g., icon_main).
Android Considerations
- The plugin supports SDK 16+, but remains inactive below SDK 25 (Android 7.1).
- Referenced drawables in your Dart code may require explicit marking for retention in release builds.
Code Examples
// Initialize and manage quick actions final QuickActions quickActions = const QuickActions(); quickActions.initialize((shortcutType) { // Handle different quick action types }); quickActions.setShortcutItems([ const ShortcutItem( type: 'action_main', localizedTitle: 'Main View', icon: 'ic_main_view', ), const ShortcutItem( type: 'action_settings', localizedTitle: 'Settings', icon: 'ic_settings', ), ]);
// Android-specific drawable marking for retention android.enableAapt2 = true aaptOptions { noCompress '.*\.(png|gif|jpg|jpeg)' }
Conclusion
This Flutter plugin empowers you to seamlessly integrate and manage home screen quick actions, enhancing the user experience and application discoverability.