Leverage the Speech-to-Text Plugin for Effortless Speech Recognition in Flutter Apps
Published on by Flutter News Hub
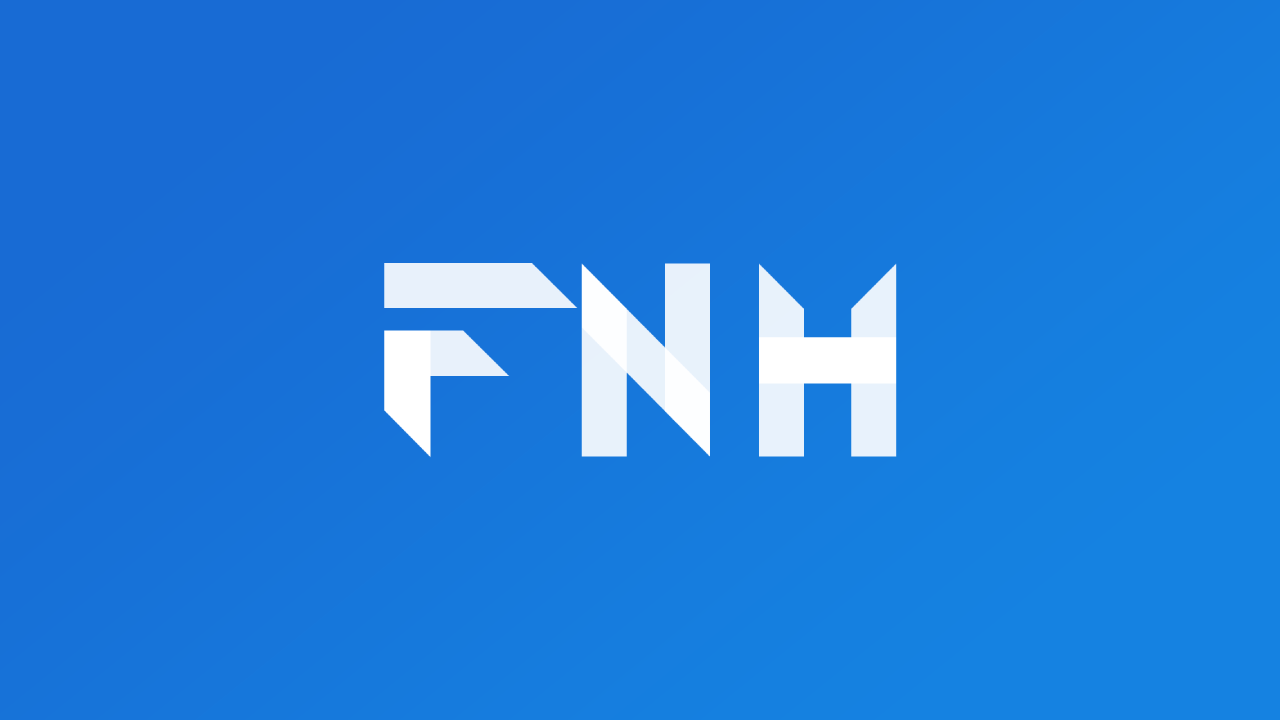
Speech recognition has become an integral part of modern applications, enabling users to interact with devices and services using their voice. The speech_to_text plugin for Flutter provides a comprehensive solution for developers to integrate speech recognition capabilities into their apps, seamlessly supporting Android, iOS, and web platforms.
Installation and Setup
To incorporate speech recognition into your Flutter project, begin by adding the speech_to_text package to your pubspec.yaml file:
dependencies: speech_to_text: ^6.6.1
Next, initialize the plugin in your Dart code:
import 'package:speech_to_text/speech_to_text.dart'; void main() { SpeechToText speech = SpeechToText(); // ... Initialize and use the plugin }
Permissions:
For the plugin to function correctly, ensure you have the necessary permissions in place:
- iOS: Add the following keys to your Info.plist file:
- NSSpeechRecognitionUsageDescription
- NSMicrophoneUsageDescription
- Android: Add the following permission to your AndroidManifest.xml file:
- android.permission.RECORD_AUDIO
Usage
The plugin provides a straightforward interface to manage speech recognition sessions:
Initialization:
Before using the plugin, initialize it:
bool available = await speech.initialize( onStatus: statusListener, onError: errorListener);
Starting and Stopping Speech Recognition:
To begin listening for speech, call the listen method:
speech.listen(onResult: resultListener);
To stop the active speech recognition session, call stop:
speech.stop();
Handling Results:
The plugin provides callbacks to handle the results of speech recognition:
void resultListener(SpeechRecognitionResult result) { // Process the recognized words }
Advanced Features
The plugin offers several advanced features to enhance the user experience:
Customizing Options:
Use the optional parameters in the listen method to customize recognition settings:
speech.listen( localeId: 'en-US', // Specify a specific language partialResults: true, // Enable partial results pauseDuration: Duration(seconds: 10), // Set a pause duration sound: 'start', // Play a sound when starting recognition listenMode: ListenMode.confirmation, // Set the recognition mode );
Continuous Speech Recognition:
While the plugin is designed for short speech commands, continuous recognition can be achieved using a custom implementation, as discussed in issue #63.
Browser Support:
Speech recognition is supported on certain browsers. Check the browser user agent to determine compatibility.
Troubleshooting
Encountering issues? Here are some common troubleshooting tips:
iOS:
- Ensure the device has the necessary languages installed for speech recognition.
- Check for conflicting sound plugins that may interfere with recognition.
Android:
- Set the compileSdkVersion to 31 or higher in your build.gradle file.
- Grant the RECORD_AUDIO permission in the AndroidManifest.xml file.
- Note that Android speech recognition has a brief pause timeout.
Conclusion
The speech_to_text plugin empowers Flutter developers to harness the power of speech recognition in their applications. With its user-friendly API and advanced features, this plugin enables seamless integration of speech capabilities, enhancing the user experience and driving innovation in voice-based interactions.