Launch URLs Seamlessly with Flutter's url_launcher Plugin
Published on by Flutter News Hub
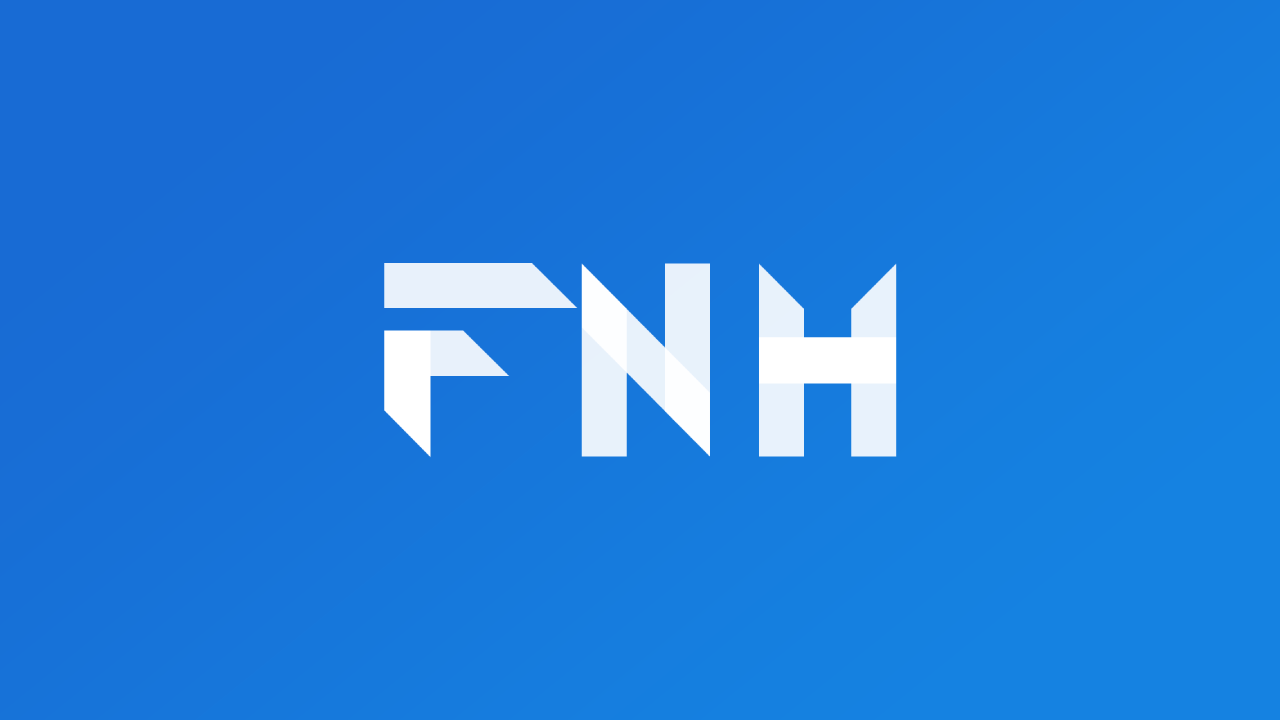
The url_launcher plugin for Flutter empowers developers to effortlessly launch URLs from their applications. With comprehensive support across various platforms, this plugin makes it a breeze to open web pages, send emails, initiate phone calls, or perform other URL-based actions.
Installation and Usage
To incorporate the url_launcher plugin into your Flutter project, add the following dependency to your pubspec.yaml file:
dependencies: url_launcher: ^6.1.0
Once the plugin is installed, you can start launching URLs with just a few lines of code:
import 'package:url_launcher/url_launcher.dart'; Future launchUrl(String url) async { if (await canLaunchUrl(Uri.parse(url))) { await launchUrl(Uri.parse(url)); } else { throw 'Could not launch $url'; } }
Supported URL Schemes
The supported URL schemes vary depending on the platform and installed applications. Some commonly used schemes include:
- https://: Opens a web page in the default browser
- mailto:: Creates an email in the default email app
- tel:: Initiates a phone call using the default phone app
- sms:: Sends an SMS message using the default messaging app
- file:: Opens a file or folder using the default app association (supported on desktop platforms)
Handling Unsupported URLs
If you need to determine whether a specific URL scheme is supported before launching it, you can use the canLaunchUrl function:
if (await canLaunchUrl(Uri.parse(url))) { // URL scheme is supported } else { // URL scheme is not supported }
Customizing Launch Behavior
On some platforms, web URLs can be launched either in an in-app web view or in the default browser. The launchUrl function provides a LaunchMode parameter to specify the desired behavior:
await launchUrl(Uri.parse(url), mode: LaunchMode.inAppWebView);
Additional Notes
- For non-http or https schemes, use encodeQueryParameters to properly encode query parameters, as shown below:
String encodeQueryParameters(Map params) { return params.entries.map((MapEntry e) => '${e.key}=${e.value}').join('&'); } // ... final Uri emailLaunchUri = Uri( scheme: 'mailto', path: '[email protected]', query: encodeQueryParameters({ 'subject': 'Example Subject & Symbols are allowed!', }), ); launchUrl(emailLaunchUri);
- For SMS encoding, use the following approach:
final Uri smsLaunchUri = Uri( scheme: 'sms', path: '0118 999 881 999 119 7253', queryParameters: { 'body': Uri.encodeComponent('Example Subject & Symbols are allowed!'), }, );
- If you need to launch URLs that cannot be expressed by Uri, use the alternate APIs provided by importing url_launcher_string.dart, but only as a last resort.
- On macOS, ensure you have the necessary entitlements to access files outside your application's sandbox.
By leveraging the url_launcher plugin, you can seamlessly launch URLs from your Flutter applications, enhancing user interactions and broadening your app's functionality.