Just Audio: A Comprehensive Guide for Feature-Rich Audio Playback in Flutter
Published on by Flutter News Hub
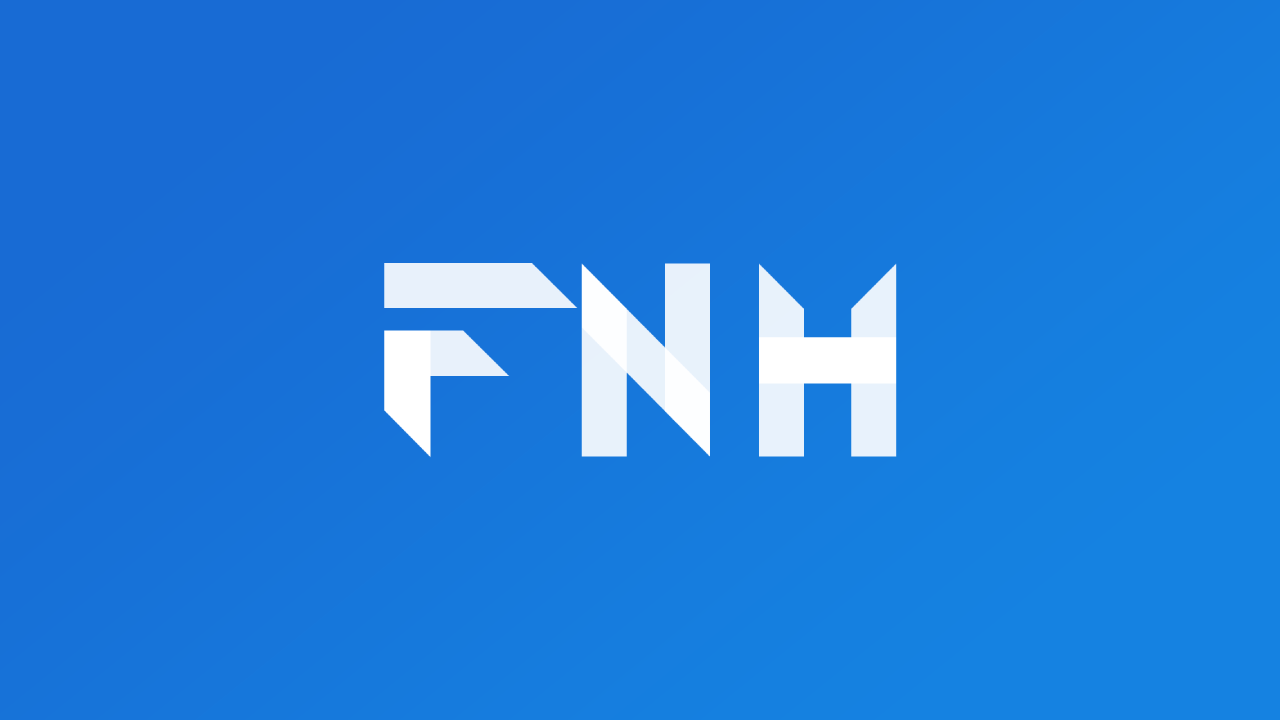
Just Audio is a powerful audio player for Flutter that offers an extensive range of features for developing feature-rich audio applications. It supports various audio sources, including URLs, files, and byte streams, and provides fine-grained control over playback, buffering, and more.
Basic Usage
To use Just Audio, add it to your Flutter project's pubspec.yaml file:
dependencies: just_audio: ^latest_version
Import the package into your Dart code:
import 'package:just_audio/just_audio.dart';
To play audio from a URL, use the setUrl method:
final player = AudioPlayer(); await player.setUrl('https://example.com/my-audio.mp3'); await player.play();
To play audio from a file, use the setAsset method:
final player = AudioPlayer(); await player.setAsset('assets/my-audio.mp3'); await player.play();
To play audio from a byte stream, use the setAudioSource method:
final player = AudioPlayer(); final audioSource = AudioSource.uri(Uri.parse('https://example.com/my-audio.mp3')); await player.setAudioSource(audioSource); await player.play();
Advanced Features
Just Audio provides a wide range of advanced features, including:
- Buffering and caching: Just Audio automatically buffers and caches audio data to minimize interruptions during playback.
- Playback control: You can play, pause, seek, and control the playback speed and volume.
- Looping and shuffling: Just Audio supports looping and shuffling of audio tracks.
- Metadata retrieval: Just Audio provides access to metadata such as the title, artist, and album of the currently playing track.
- Error handling: Just Audio provides error events and a way to catch exceptions related to audio playback.
State Streams
Just Audio offers various state streams that provide real-time updates on the player's state, including:
- playerStateStream: Provides the current playing and processingState of the player.
- processingStateStream: Provides updates on the player's processing state, such as idle, loading, buffering, and completed.
- sequenceStateStream: Provides updates on the current sequence (playlist) and its state.
- playbackEventStream: Provides events related to playback, such as pause, resume, seek, and position.
Platform Support
Just Audio supports a wide range of platforms, including:
- Android
- iOS
- macOS
- Web
- Windows
- Linux
Platform-Specific Configuration
For some platforms, additional configuration may be required. For example, on iOS, you may need to add the following to your Info.plist file:
<key>NSAppTransportSecurity</key> <dict> <key>NSAllowsArbitraryLoads</key> <true/> </dict>
Consult the Just Audio documentation for more details on platform-specific configuration.
Troubleshooting
Common troubleshooting tips include:
- Ensure that the audio file is in a supported format.
- Check the server headers to ensure that they are correct and include appropriate Content-Type and Content-Length headers.
- Verify that the file name extension matches the actual audio format.
Conclusion
Just Audio is a robust and feature-rich audio player for Flutter that enables developers to create engaging and immersive audio experiences. Its comprehensive API, state streams, and cross-platform support make it an excellent choice for a wide range of audio applications.