Introducing Flutter Earth Globe: A Comprehensive Interactive Globe Widget for Flutter
Published on by Flutter News Hub
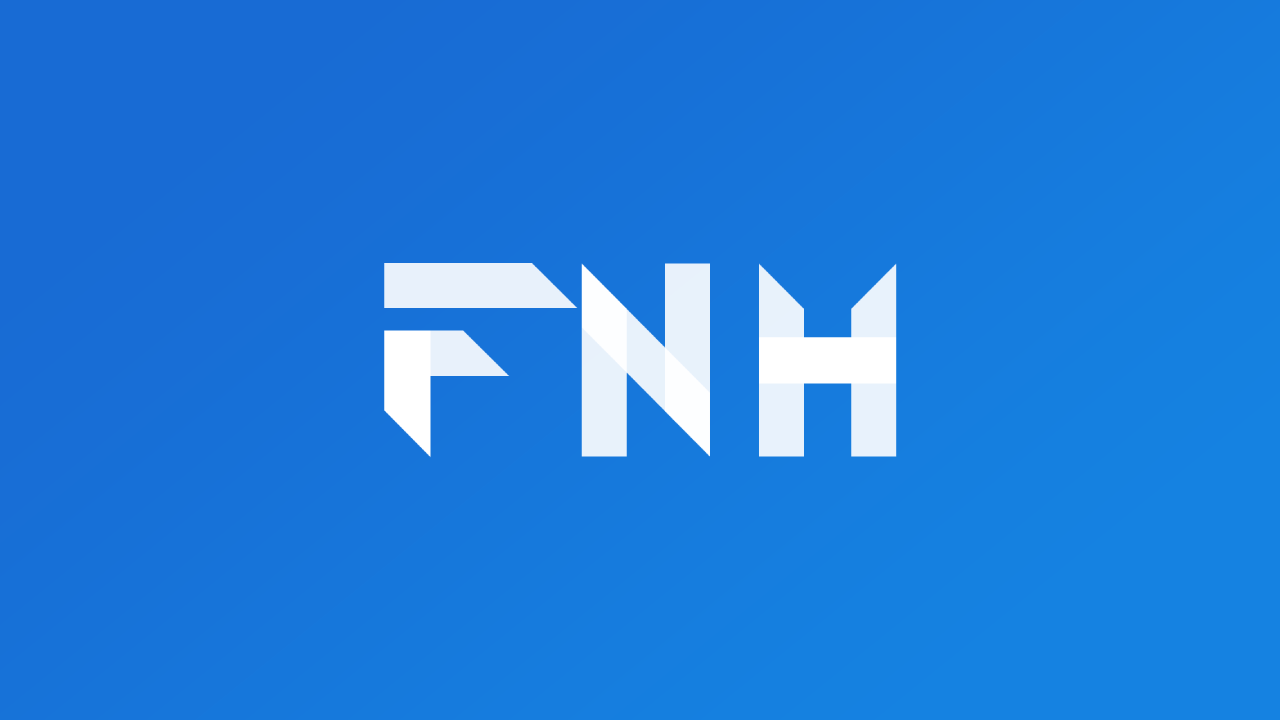
Overview
Flutter Earth Globe is an indispensable asset for Flutter developers seeking to incorporate interactive 3D globes into their applications. This feature-rich widget exudes elegance and adaptability, making it a compelling choice for visualizing planets or any other spherical data.
Live Demo
Witness the Earth Globe's capabilities in action at https://pana-g.github.io/flutter_earth_globe/.
Features
- 3D Interactive Globe: Experience a realistic and engaging 3D globe model of Earth.
- Customizable Appearance: Personalize the globe's appearance with custom colors, textures, and more.
- Zoom and Rotation: Explore the globe seamlessly with intuitive zoom and rotation gestures.
- Point Support: Pinpoint locations with customizable points.
- Connections Support: Establish connections between different coordinates.
- Custom Labels Support: Add custom labels to points or connections for enhanced visualization.
- Responsive Design: Adapt to various devices and screen sizes effortlessly.
Installation
To incorporate Flutter Earth Globe into your project:
- Add it to your
pubspec.yaml
file:
dependencies:
flutter_earth_globe: ^[latest_version]
- Import the package into your Dart code:
import 'package:flutter_earth_globe/flutter_earth_globe.dart';
Usage
Here's a basic example of how to use Flutter Earth Globe:
import 'package:flutter/material.dart';
import 'package:flutter_earth_globe/flutter_earth_globe.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
late FlutterEarthGlobeController _controller;
@override
initState() {
_controller = FlutterEarthGlobeController(
rotationSpeed: 0.05,
isBackgroundFollowingSphereRotation: true,
background: Image.asset('assets/2k_stars.jpg').image,
surface: Image.asset('assets/2k_earth-day.jpg').image);
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Flutter Earth Globe Example')),
body: SafeArea(
child: FlutterEarthGlobe(
controller: _controller,
radius: 120,
),
),
),
);
}
}
Customization
Tailor the Earth Globe to your needs with these customization options:
- Points: Create and add points to the globe:
final FlutterEarthGlobeController _controller = FlutterEarthGlobeController();
List points = [
Point(
id: '1',
coordinates: const GlobeCoordinates(51.5072, 0.1276),
label: 'London',
isLabelVisible: true,
style: const PointStyle(color: Colors.red, size: 6)),
Point(
id: '2',
isLabelVisible: true,
coordinates: const GlobeCoordinates(40.7128, -74.0060),
style: const PointStyle(color: Colors.green),
onHover: () {},
label: 'New York'),
// ... more points
];
for (var point in points) {
_controller.addPoint(point);
}
- Background and Surface: Display custom images as the background and surface:
final FlutterEarthGlobeController _controller = FlutterEarthGlobeController();
@override
initState(){
_controller.onLoaded = () {
_controller.loadBackground(Image.asset('assets/2k_stars.jpg').image, followsRotation: true);
_controller.loadSurface(Image.asset('assets/2k_earth-day.jpg',).image, );
};
super.initState();
}
- Sphere Style: Modify the appearance of the sphere:
final FlutterEarthGlobeController _controller = FlutterEarthGlobeController();
_controller.changeSphereStyle(SphereStyle(shadowColor: Colors.orange.withOpacity(0.8), shadowBlurSigma: 20));
Support the Project
Support Flutter Earth Globe by:
- Thumbs up on pub
- Star on GitHub
- Reporting issues
- Making pull requests
With your help, we can enhance this library further!