Introducing BLoC: A Powerful Design Pattern for Flutter and Dart
Published on by Flutter News Hub
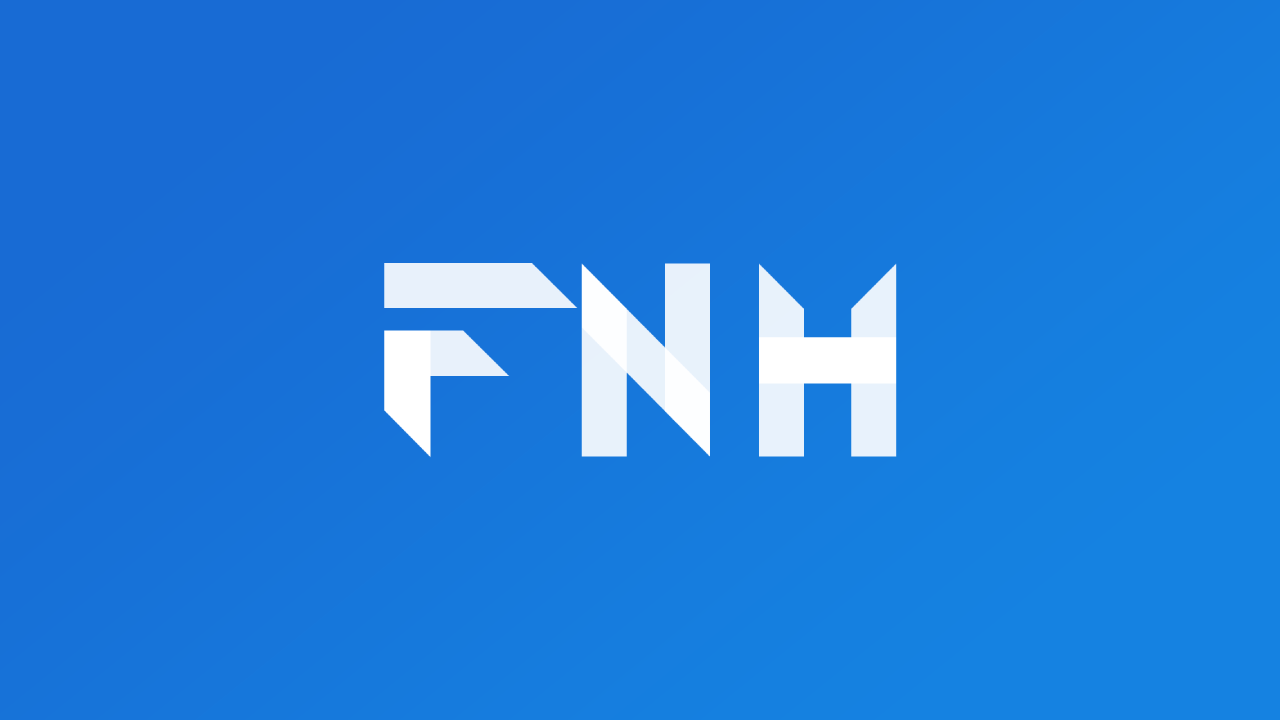
The BLoC (Business Logic Component) Design Pattern is a popular approach to managing complex state and business logic in Dart applications, particularly in Flutter development. This pattern helps separate presentation logic from business logic, leading to improved testability, reusability, and code maintainability.
Understanding Cubits and Blocs: Core Concepts in BLoC
Cubits:
- A Cubit is a simple state management tool that allows you to manage a single state value.
- It provides a direct way to update the state by calling the emit method, making it straightforward to implement basic state management.
Blocs:
- Blocs are more advanced than Cubits and are used for complex state management scenarios.
- They follow a request-response pattern, where events are used to trigger state changes.
- Blocs offer advanced features such as event transformations, error handling, and transition tracking.
Creating a Cubit
class CounterCubit extends Cubit { CounterCubit() : super(0); void increment() => emit(state + 1); }
Using a Cubit
void main() { final cubit = CounterCubit(); print(cubit.state); // 0 cubit.increment(); print(cubit.state); // 1 cubit.close(); }
Creating a Bloc
class CounterBloc extends Bloc { CounterBloc() : super(0) { on((event, emit) => emit(state + 1)); } } sealed class CounterEvent {} final class CounterIncrementPressed extends CounterEvent {}
Using a Bloc
Future main() async { final bloc = CounterBloc(); print(bloc.state); // 0 bloc.add(CounterIncrementPressed()); await Future.delayed(Duration.zero); print(bloc.state); // 1 await bloc.close(); }
Observing State Changes
- Both Cubits and Blocs provide hooks to observe state changes.
- You can override methods like onChange and onTransition to track state changes and perform custom actions.
Benefits of Using BLoC
- Separation of Concerns: BLoC helps separate presentation logic from business logic, enhancing code organization and maintainability.
- Testability: Cubits and Blocs provide clear interfaces for testing, making it easier to test state management scenarios.
- Reusable Logic: BLoC components can be reused across different parts of your application, reducing code duplication.
- Optimized Performance: BLoC optimizes state management by minimizing unnecessary rebuilds and improving performance.
Conclusion
The BLoC Design Pattern is a powerful tool for managing state and business logic in Dart applications. By leveraging Cubits and Blocs, developers can achieve effective state management, improved code quality, and a more robust and maintainable codebase.