Integrate Sign in with Apple into Your Flutter App
Published on by Flutter News Hub
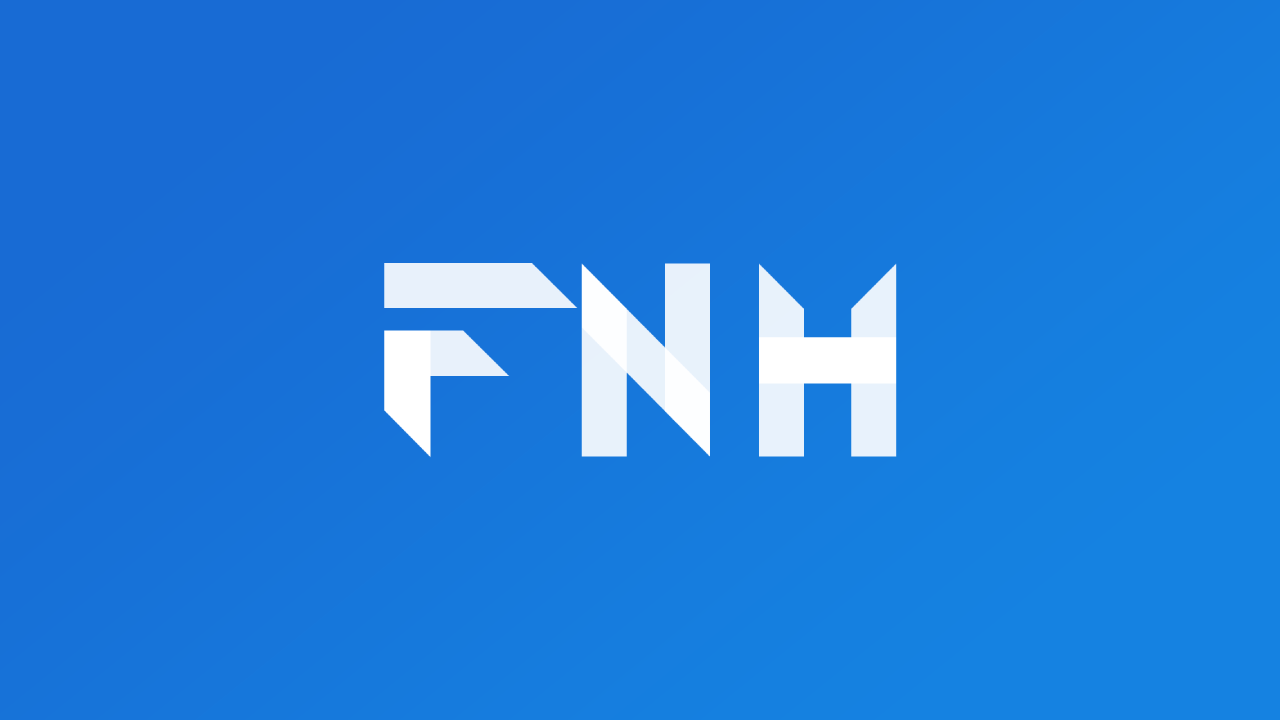
Sign in with Apple is a convenient and secure way for users to authenticate with your Flutter app using their existing Apple ID credentials. This plugin provides a bridge to integrate this functionality seamlessly into your project.
Supported Platforms
- iOS
- macOS
- Android
- Web
Example Usage
import 'package:sign_in_with_apple/sign_in_with_apple.dart'; class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Sign in with Apple', home: Scaffold( appBar: AppBar( title: Text('Sign in with Apple example'), ), body: Container( child: Center( child: SignInWithAppleButton( onPressed: () async { final credential = await SignInWithApple.getAppleIDCredential( scopes: [ AppleIDAuthorizationScopes.email, AppleIDAuthorizationScopes.fullName, ], ); print(credential); // Send the credential (especially `credential.authorizationCode`) to your server to create a session after they have been validated with Apple (see Integration section for more information on how to do this) }, ), ), ), ), ); } }
Flow
Integration
Prerequisites:
- A paid membership to the Apple Developer Program
Setup:
1. Register an App ID:
- Create a new App ID at https://developer.apple.com/account/resources/identifiers/list/bundleId.
2. Create a Service ID (for Web and Android integration):
- Go to https://developer.apple.com/account/resources/identifiers/list/serviceId.
- Register a new Service ID.
- Enable Sign in with Apple for the service.
- Add the domains and subdomains where you want to use Sign in with Apple.
Server:
- Example server setup on Glitch
- Validate incoming authorization codes from your app clients with Apple's servers.
- Derive a new session from the validated credentials in your system.
- Daily verify the session with Apple and revoke the session if the authorization has been withdrawn on Apple's side.
Android:
- Use the Android V2 Embedding.
- Ensure launchMode is singleTask or singleTop.
iOS:
- Add the Sign in with Apple capability to your app's capabilities.
- Create and download new Provisioning Profiles with the Sign in with Apple capability.
macOS:
- Ensure the "Sign in with Apple" capability is present in your app's capabilities.
- Register your Mac for local development.