Initialize and Use Time Zone Database in Dart
Published on by Flutter News Hub
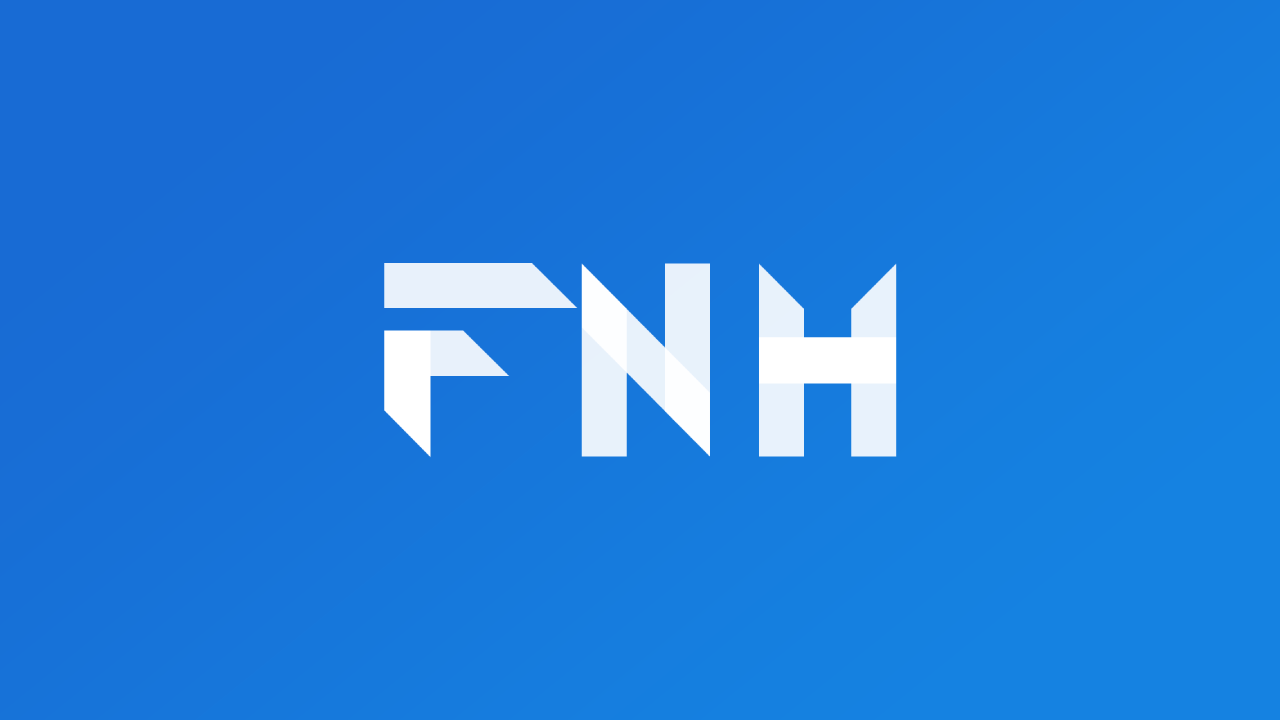
Time zones are essential for dealing with dates and times in different geographical regions. The timezone
package for Dart provides access to the IANA time zone database, allowing developers to work with time zones effectively.
Initialization
Before using the timezone
package, you must initialize the selected time zone database. There are three methods for initialization, depending on the environment:
Dart Library Initialization:
import 'package:timezone/data/latest.dart' as tz;
void main() {
tz.initializeTimeZones();
// Initialize other database variants:
// tz.initializeTimeZones('latest_all.dart') for the full database
// tz.initializeTimeZones('latest_10y.dart') for a smaller database
}
Browser Environment Initialization:
import 'package:timezone/browser.dart' as tz;
Future setup() async {
await tz.initializeTimeZone();
}
Standalone Environment Initialization:
import 'package:timezone/standalone.dart' as tz;
Future setup() async {
await tz.initializeTimeZone();
}
Getting Time Zone Locations
After initialization, you can use the getLocation
function to obtain a Location
object representing a specific time zone.
var detroit = tz.getLocation('America/Detroit');
TimeZone Object
The TimeZone
object represents a specific time zone at a particular point in time. You can obtain a TimeZone
object using the timeZone
function, passing in a location and a timestamp in milliseconds since the epoch.
var timeInUtc = DateTime.utc(1995, 1, 1);
var timeZone = detroit.timeZone(timeInUtc.millisecondsSinceEpoch);
TimeZone Aware DateTime
The TZDateTime
class extends the DateTime
class from dart:core
, adding time zone awareness. You can create a TZDateTime
object using the TZDateTime
constructor.
var date = tz.TZDateTime(detroit, 2014, 11, 17);
Converting DateTimes Between Time Zones
To convert a DateTime
or TZDateTime
object between time zones, create a new TZDateTime
object using the from
constructor.
var localTime = tz.DateTime(2010, 1, 1);
var detroitTime = tz.TZDateTime.from(localTime, detroit);
Listing Known Time Zones
The timeZoneDatabase
top-level member contains all known time zones after initialization.
var locations = tz.timeZoneDatabase.locations;
Time Zone Database Updates
The timezone
package provides a script (tool/refresh.sh
) to update the time zone database.
$ chmod +x tool/refresh.sh
$ tool/refresh.sh
By following these steps, developers can easily initialize and use the IANA time zone database in Dart applications, ensuring accurate and efficient handling of dates and times across different time zones.