Infinite Scroll Pagination for Flutter Applications
Published on by Flutter News Hub
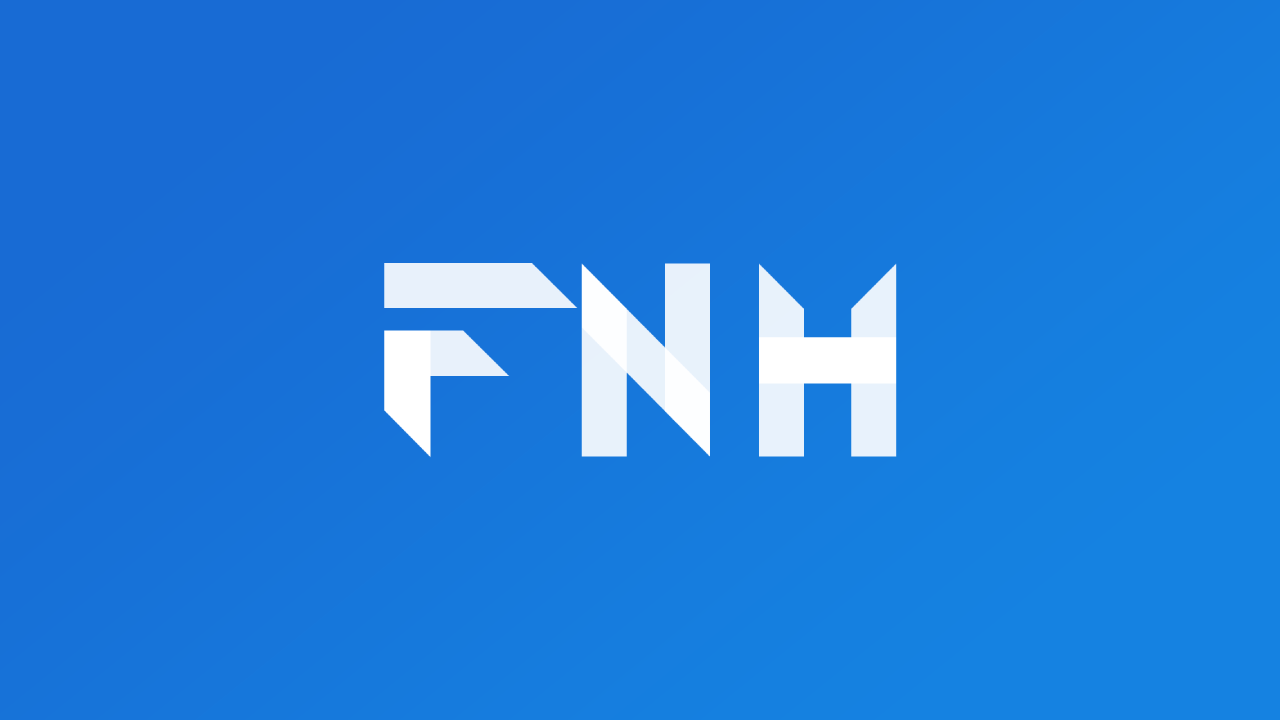
Infinite scroll pagination, also known as endless scrolling, lazy loading, or progressive loading, is a technique used to load data incrementally as the user scrolls down a list. This approach enhances the user experience by eliminating the need for manual pagination or loading indicators.
The 'infinite_scroll_pagination' package provides a comprehensive solution for implementing infinite scrolling in Flutter applications. It offers an unopinionated, extensible, and highly customizable API that seamlessly integrates with various state management approaches and layout widgets.
Getting Started
To start using the 'infinite_scroll_pagination' package, add it as a dependency to your Flutter project's 'pubspec.yaml' file:
dependencies: infinite_scroll_pagination: ^X.Y.Z
Next, import the package into your Dart code:
import 'package:infinite_scroll_pagination/infinite_scroll_pagination.dart';
Core Concepts
The package includes two key components:
- PagingController: Manages the pagination logic, including fetching data from your API, managing the page state, and triggering page requests.
- PagedChildBuilderDelegate: Defines how to build and display the paginated items.
Basic Usage
The following code snippet demonstrates a basic implementation of infinite scrolling pagination in a Flutter application:
class BeerListView extends StatefulWidget { // Define the page size for each request static const _pageSize = 20; @override _BeerListViewState createState() => _BeerListViewState(); } class _BeerListViewState extends State { // Create the PagingController final PagingController _pagingController = PagingController(firstPageKey: 0); @override void initState() { // Add a listener to the PagingController to fetch new data as the user scrolls _pagingController.addPageRequestListener((pageKey) { _fetchPage(pageKey); }); super.initState(); } // Fetch a page of data from the API Future _fetchPage(int pageKey) async { try { // Simulate API call by fetching a list of items final newItems = await RemoteApi.getBeerList(pageKey, BeerListView._pageSize); // Check if it's the last page final isLastPage = newItems.length < BeerListView._pageSize; // Append the new items to the PagingController if (isLastPage) { _pagingController.appendLastPage(newItems); } else { final nextPageKey = pageKey + newItems.length; _pagingController.appendPage(newItems, nextPageKey); } } catch (error) { // Handle error by setting it on the PagingController _pagingController.error = error; } } // Build the PagedListView widget @override Widget build(BuildContext context) => PagedListView( pagingController: _pagingController, builderDelegate: PagedChildBuilderDelegate( itemBuilder: (context, item, index) => BeerListItem(beer: item), ), ); @override void dispose() { // Dispose the PagingController when the widget is disposed _pagingController.dispose(); super.dispose(); } }
Key Features
- State Management Agnostic: Works seamlessly with any state management approach, including setState, BLoC, and more.
- Layout Agnostic: Provides out-of-the-box widgets for GridView, SliverGrid, ListView, and SliverList layouts, and allows for custom layouts.
- API Agnostic: Supports any pagination strategy, giving you complete control over API calls.
- Highly Customizable: Customize the appearance of progress indicators, error messages, and empty lists.
- Extensible: Easily integrate with pull-to-refresh, filtering, sorting, and more.
- Status Change Listeners: Not only displays status indicators, but also provides listeners for handling events like errors and empty lists.
Advanced Usage
Customizing Indicators
You can customize the progress, error, and empty list indicators by providing your own widgets to the PagedChildBuilderDelegate:
PagedChildBuilderDelegate( itemBuilder: (context, item, index) => BeerListItem(beer: item), progressBuilder: (context) => const Center(child: CircularProgressIndicator()), firstPageErrorIndicatorBuilder: (context) => const Center(child: Text('Error')), firstPageProgressIndicatorBuilder: (context) => const Center(child: CircularProgressIndicator()), newPageErrorIndicatorBuilder: (context) => const Center(child: Text('Error')), newPageProgressIndicatorBuilder: (context) => const Center(child: CircularProgressIndicator()), noItemsFoundIndicatorBuilder: (context) => const Center(child: Text('No Items')), )
Pull-to-Refresh Integration
To integrate pull-to-refresh, use a RefreshIndicator widget:
RefreshIndicator( onRefresh: () async { // Refresh the PagingController by calling its refresh() method _pagingController.refresh(); }, child: PagedListView(...), )
Filtering and Sorting
You can filter or sort the paginated data by using a StreamController and updating the PagingController with the filtered/sorted results:
// StreamController to listen for filter changes final filterStreamController = StreamController(); // Update the PagingController with filtered results filterStreamController.stream.listen((filter) { // Filter the data based on the provided filter final filteredData = RemoteApi.getBeerList(pageKey, BeerListView._pageSize).where((beer) => beer.name.contains(filter)); // Update the PagingController with the filtered data _pagingController.replace(filteredData); });
Conclusion
The 'infinite_scroll_pagination' package provides a robust and flexible solution for implementing infinite scroll pagination in Flutter applications. Its unopinionated design, extensibility, and customization options make it an ideal choice for handling pagination scenarios of any complexity.