Image Picker Plugin for Flutter: A Comprehensive Guide
Published on by Flutter News Hub
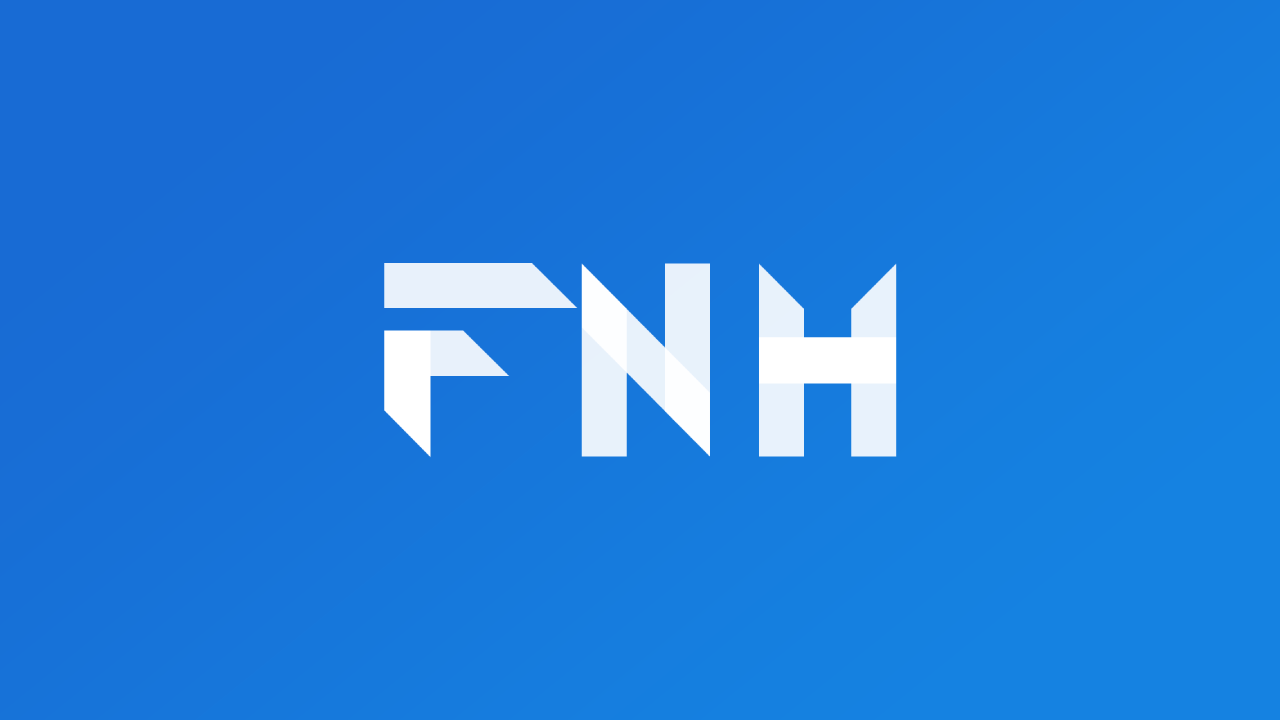
The Image Picker plugin for Flutter provides a straightforward way to pick images and videos from the user's camera or device gallery. This plugin supports multiple file selection, lost data retrieval, and offers flexibility for handling MainActivity destruction on Android.
Installation
iOS:
- Add image_picker as a dependency in your pubspec.yaml file.
- Include the following keys in your Info.plist file:
- NSPhotoLibraryUsageDescription - Explain why your app needs photo library permission.
- NSCameraUsageDescription - Provide a reason for camera access.
- NSMicrophoneUsageDescription - Optional for video recording.
Android:
- Add image_picker to your pubspec.yaml file.
- No further configuration is required.
Windows, macOS, and Linux:
- Install image_picker and file_selector packages.
- Add filesystem access entitlement (com.apple.security.files.user-selected.read-only) for macOS.
Handling MainActivity Destruction on Android
When facing memory issues, Android may terminate the MainActivity using the image picker. To handle this scenario, use the ImagePicker.retrieveLostData() method to retrieve the lost data.
Future getLostData() async { final ImagePicker picker = ImagePicker(); final LostDataResponse response = await picker.retrieveLostData(); if (response.isEmpty) { return; } final List? files = response.files; if (files != null) { _handleLostFiles(files); } else { _handleError(response.exception); } }
Usage
Picking Images and Videos:
final ImagePicker picker = ImagePicker(); final XFile? image = await picker.pickImage(source: ImageSource.gallery); final XFile? photo = await picker.pickImage(source: ImageSource.camera); final XFile? galleryVideo = await picker.pickVideo(source: ImageSource.gallery); final XFile? cameraVideo = await picker.pickVideo(source: ImageSource.camera);
Multiple Selection:
final List images = await picker.pickMultiImage(); final List medias = await picker.pickMultipleMedia();