Image Cropping with Flutter's Image Cropper Plugin
Published on by Flutter News Hub
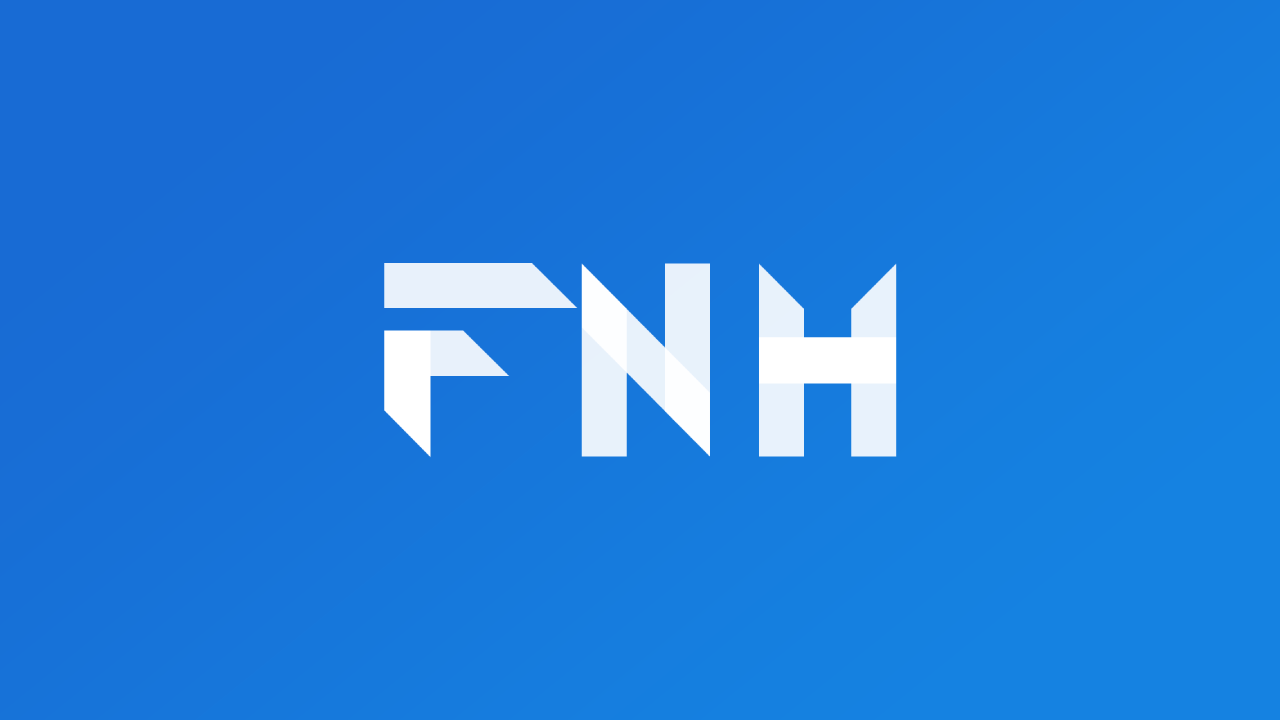
The Image Cropper plugin for Flutter allows you to easily crop images from your mobile applications. It provides a consistent UI across different platforms (Android, iOS, and Web) and supports various cropping options.
Implementation
1. Installation
Add the Image Cropper plugin to your Flutter project's pubspec.yaml file:
dependencies: image_cropper: ^1.5.0
Run flutter pub get to install the plugin.
2. Usage
Import the Image Cropper into your Dart code:
import 'package:image_cropper/image_cropper.dart';
3. Crop an Image
To crop an image, use the cropImage method of the ImageCropper class. The following code crops an image from a file:
final croppedFile = await ImageCropper.cropImage( sourcePath: 'path/to/original_image.jpg', aspectRatioPresets: [ CropAspectRatioPreset.square, CropAspectRatioPreset.ratio3x2, CropAspectRatioPreset.original, CropAspectRatioPreset.ratio4x3, CropAspectRatioPreset.ratio16x9, ], );
4. Customization
The Image Cropper plugin allows you to customize the UI and cropping behavior based on the platform. You can specify different settings for Android, iOS, and Web:
Android:
uiSettings: [ AndroidUiSettings( toolbarTitle: 'My Cropper', toolbarColor: Colors.deepOrange, toolbarWidgetColor: Colors.white, initAspectRatio: CropAspectRatioPreset.original, lockAspectRatio: false, ), ],
iOS:
uiSettings: [ IOSUiSettings( title: 'My Cropper', ), ],
Web:
uiSettings: [ WebUiSettings( context: context, // BuildContext of the current screen ), ],
Sample Code
Here's a complete example of cropping an image using the Image Cropper plugin:
import 'package:flutter/material.dart'; import 'package:image_picker/image_picker.dart'; import 'package:image_cropper/image_cropper.dart'; class ImageCropExample extends StatefulWidget { @override _ImageCropExampleState createState() => _ImageCropExampleState(); } class _ImageCropExampleState extends State { CroppedFile? _croppedFile; Future _cropImage() async { final imageFile = await ImagePicker().pickImage(source: ImageSource.gallery); if (imageFile != null) { final croppedFile = await ImageCropper.cropImage( sourcePath: imageFile.path, aspectRatioPresets: [ CropAspectRatioPreset.square, CropAspectRatioPreset.ratio3x2, CropAspectRatioPreset.original, CropAspectRatioPreset.ratio4x3, CropAspectRatioPreset.ratio16x9, ], uiSettings: [ AndroidUiSettings( toolbarTitle: 'My Cropper', toolbarColor: Colors.deepOrange, toolbarWidgetColor: Colors.white, initAspectRatio: CropAspectRatioPreset.original, lockAspectRatio: false, ), IOSUiSettings( title: 'My Cropper', ), WebUiSettings( context: context, // BuildContext of the current screen ), ], ); if (croppedFile != null) { setState(() { _croppedFile = croppedFile; }); } } } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Image Cropper Example')), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ if (_croppedFile != null) Image.file(_croppedFile!.path), ElevatedButton( onPressed: _cropImage, child: Text('Crop Image'), ), ], ), ), ); } }
Conclusion
The Image Cropper plugin provides a powerful and customizable way to crop images in your Flutter applications. It enables you to offer a consistent and user-friendly cropping experience across different platforms.