Home Widget Plugin for Flutter
Published on by Flutter News Hub
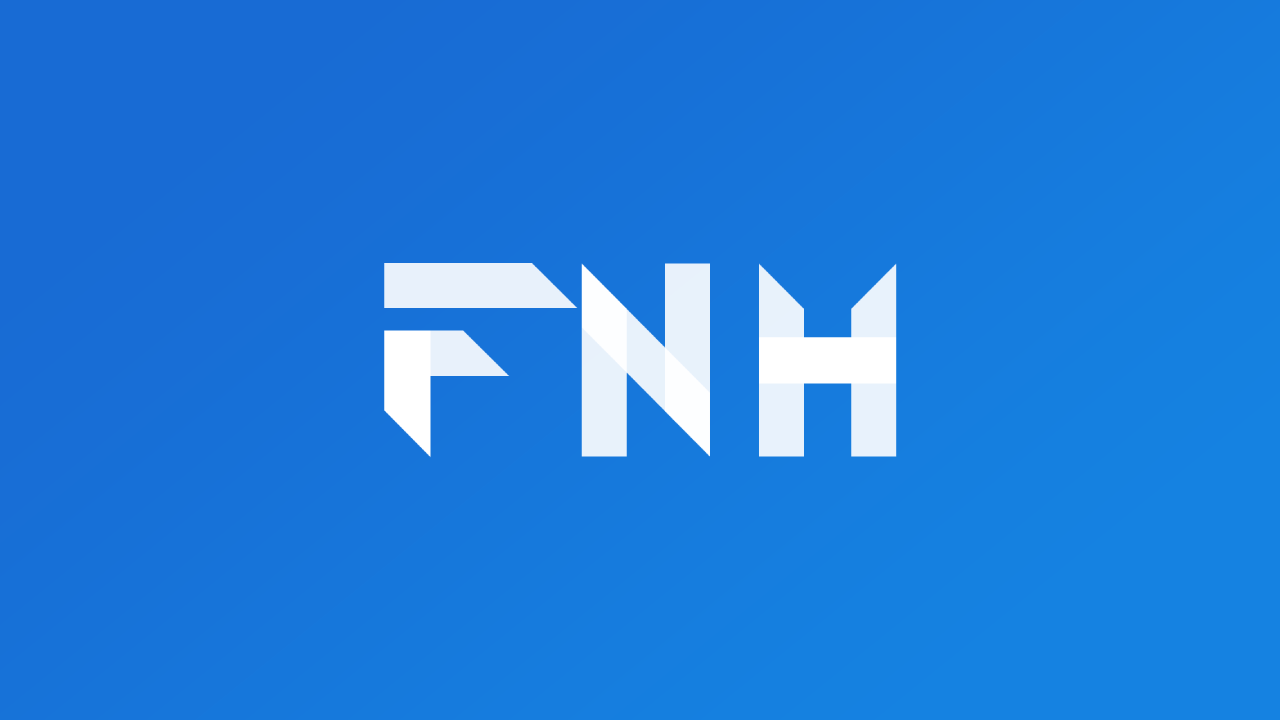
Overview
HomeWidget is an open-source Flutter plugin that facilitates the creation of Home Screen Widgets on both Android and iOS. It provides a unified interface for sending and retrieving data, aiding in the seamless updating of widgets.
Key Features:
- Easily create interactive Home Screen Widgets
- Efficient data exchange between Flutter and native widgets
- Automatic widget updates
- Interactive widgets with customizable actions
Usage
The following sections provide a comprehensive guide to using the HomeWidget plugin:
Setup:
- Add dependencies: Include home_widget as a dependency in your pubspec.yaml file.
- Register interactivity callback: Call HomeWidget.registerInteractivityCallback to register a function for handling widget interactions.
- Set app group ID (iOS only): Specify the app group ID for data sharing between your app and widget using HomeWidget.setAppGroupId.
Saving Data:
- Use HomeWidget.saveWidgetData to store data associated with the widget.
Retrieving Data:
- Use HomeWidget.getWidgetData to fetch the data stored in the widget.
Updating Widgets:
- Call HomeWidget.updateWidget to force a reload of the Home Screen Widget.
Interactive Widgets
Android (Jetpack Glance):
- Register an AppIntent in your app and pass it to the widget's intent in the onClick modifier.
Android (XML):
- Add a HomeWidgetBackgroundIntent.getBroadcast PendingIntent to the view you want to add a click listener to.
iOS:
- Use a UIAppIntent in the widget's view and specify the app group ID.
Background Update
- Utilize HomeWidget.updateWidget in the background to keep widgets up-to-date even when the app is not active.
Request Pin Widget
Android only (API 26+):
- Request users to pin the widget to their home screens using HomeWidget.requestPinWidget.
Resources
- Google Codelab
- Interactive HomeScreen Widgets with Flutter using home_widget
- iOS Lockscreen Widgets with Flutter and home_widget
Code Examples
Saving Data (Dart):
HomeWidget.saveWidgetData('id', 'Hello from Flutter!');
Retrieving Data (Dart):
String? data = await HomeWidget.getWidgetData('id', defaultValue: 'default value'); print(data); // Output: Hello from Flutter!
Interactive Widget (Android Jetpack Glance):
Text( "Title", modifier = GlanceModifier.clickable( onClick = actionStartActivity(context, Intent.parseUri("homeWidgetExample://titleClicked")) ) )
Interactive Widget (Android XML):
Add the necessary Receiver and Service to your AndroidManifest.xml file
<receiver android:name="es.antonborri.home_widget.HomeWidgetBackgroundReceiver" android:exported="true"> <intent-filter> <action android:name="es.antonborri.home_widget.action.BACKGROUND" /> </intent-filter> </receiver> <service android:name="es.antonborri.home_widget.HomeWidgetBackgroundService" android:permission="android.permission.BIND_JOB_SERVICE" android:exported="true"/>
Add a HomeWidgetBackgroundIntent.getBroadcast PendingIntent to the View you want to add a click listener to
val backgroundIntent = HomeWidgetBackgroundIntent.getBroadcast( context, Uri.parse("homeWidgetExample://titleClicked") ) setOnClickPendingIntent(R.id.widget_title, backgroundIntent)
Interactive Widget (iOS):
Button(intent: AppIntent(url: URL(string: "homeWidgetExample://titleClicked"))) { Text(entry.title).bold().font(.title) }