Hive: A Blazing Fast, Cross-Platform Database for Dart
Published on by Flutter News Hub
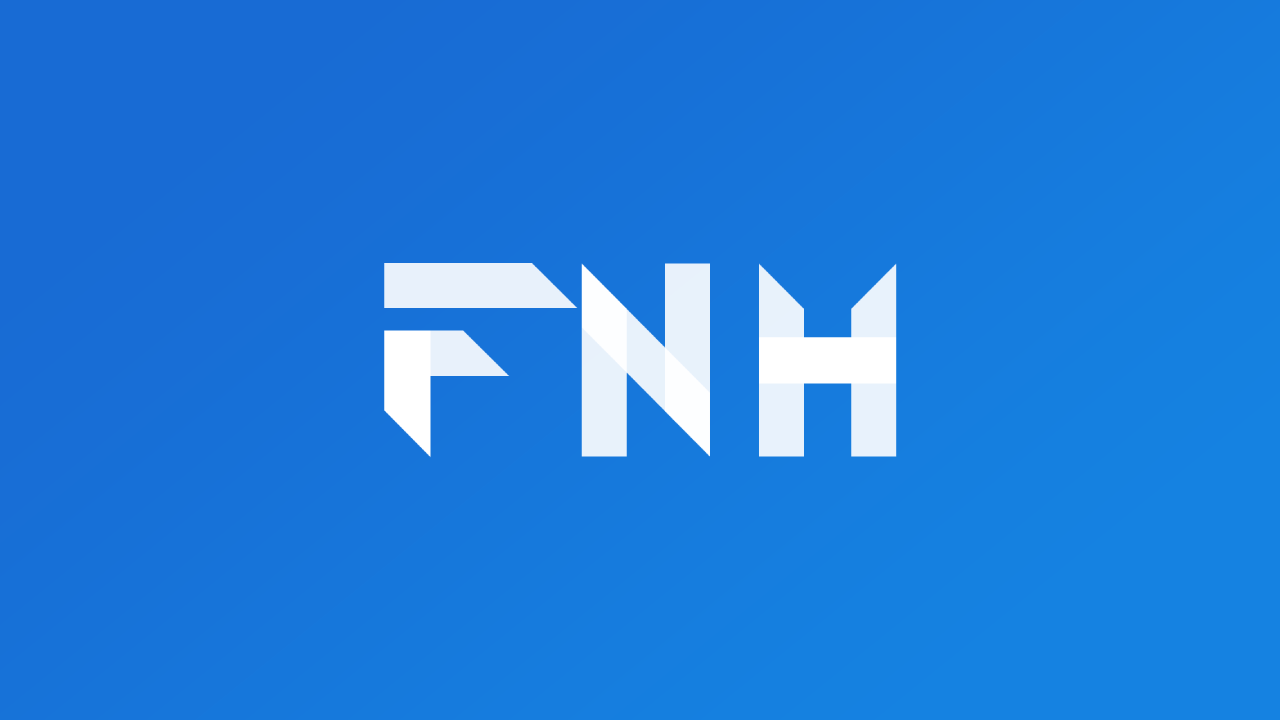
Hive is a lightweight, key-value database written in pure Dart that offers exceptional performance. Inspired by Bitcask, Hive is designed to provide a simple and efficient data storage solution for mobile, desktop, and browser applications. It stands out with its cross-platform compatibility, lightning-fast performance, and robust security features.
Features
- Blazing Speed: Hive's architecture ensures lightning-fast data retrieval and storage operations, making it an ideal choice for performance-sensitive applications.
- Cross-Platform Support: Hive is a truly cross-platform database, supporting all major platforms, including mobile, desktop, and web, without the need for native dependencies.
- Intuitive API: Hive's API is straightforward and easy to use, making it accessible to developers of all skill levels.
- Encryption and Security: Hive takes data security seriously. It includes built-in encryption capabilities to safeguard sensitive information, providing peace of mind to developers.
- Batteries Included: Hive comes with out-of-the-box support for various Dart features, including typesafe collections, optional type adapters, and much more.
Usage
Hive can be used like a conventional dictionary, allowing developers to store and retrieve data with ease.
var box = Hive.box('myBox'); box.put('name', 'David'); var name = box.get('name'); print('Name: $name');
BoxCollections for Enhanced Performance
BoxCollections in Hive group multiple boxes together, providing significant performance benefits, especially on web platforms. They allow for simultaneous opening and closing of all boxes in the collection, optimizing data storage in indexed DB.
final collection = await BoxCollection.open( 'MyFirstFluffyBox', {'cats', 'dogs'}, path: './', key: HiveCipher(), ); final catsBox = collection.openBox('cats');
Store Objects with Ease
Hive not only supports primitive data types but also enables the storage of custom Dart objects. To store objects, a type adapter must be generated.
@HiveType(typeId: 0) class Person extends HiveObject { @HiveField(0) String name; @HiveField(1) int age; }
Objects can then be stored and retrieved, providing a convenient way to manage complex data structures.
var box = await Hive.openBox('myBox'); var person = Person() ..name = 'Dave' ..age = 22; box.add(person); print(box.getAt(0)); // Dave - 22 person.age = 30; person.save(); print(box.getAt(0)); // Dave - 30
Integration with Flutter
Hive is an excellent choice for Flutter applications, providing a fast and reliable data storage solution. Its cached boxes make it suitable for use directly in the build method of Flutter widgets.
class SettingsPage extends StatelessWidget { @override Widget build(BuildContext context) { return ValueListenableBuilder( valueListenable: Hive.box('settings').listenable(), builder: (context, box, widget) { return Switch( value: box.get('darkMode'), onChanged: (val) { box.put('darkMode', val); }, ); }, ); } }
Benchmark
Hive's exceptional performance is evident in independent benchmarks. It consistently outperforms SQLite and SharedPreferences, particularly in write operations.
Conclusion
Hive is an exceptional database solution for Dart developers seeking speed, simplicity, and cross-platform compatibility. Its intuitive API, robust security features, and exceptional performance make it an ideal choice for a wide range of applications, from mobile to desktop and web. Whether you're building a high-performance app or a simple data management system, Hive has got you covered.