Harnessing Connectivity Information with Flutter's connectivity_plus Plugin
Published on by Flutter News Hub
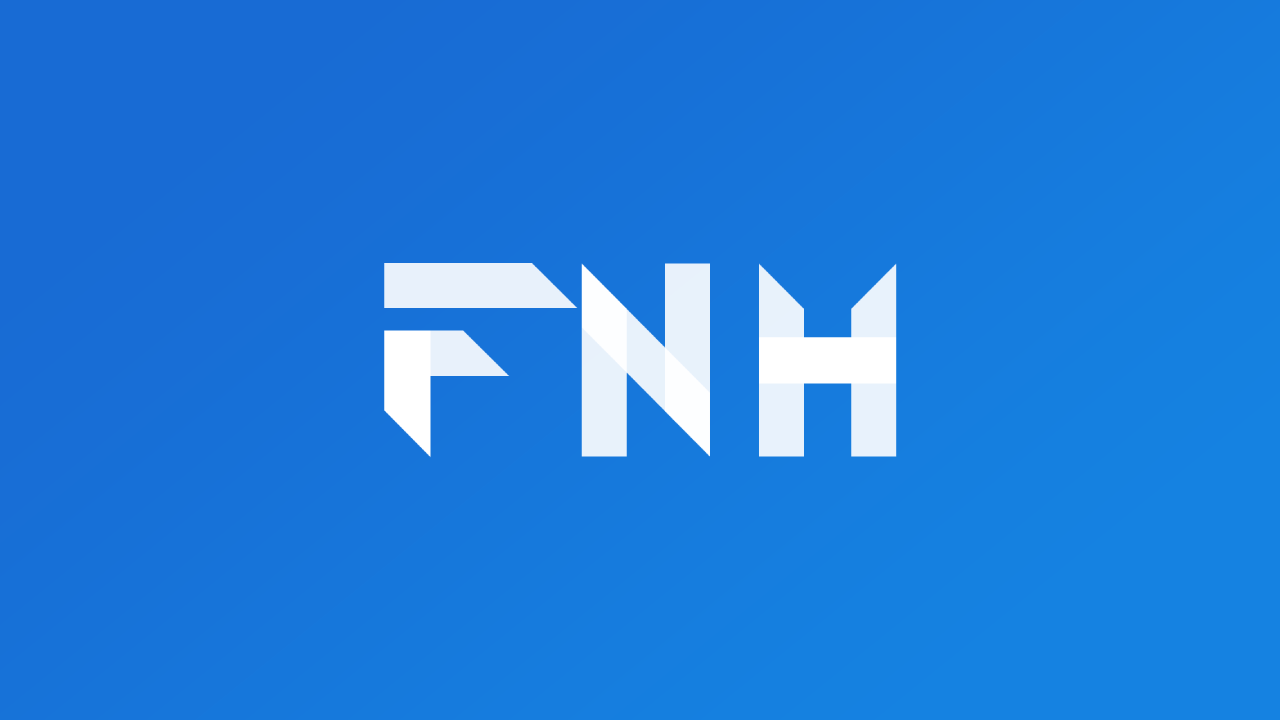
In the realm of Flutter development, understanding network connectivity is crucial for ensuring a seamless user experience. Connectivity types play a significant role in determining the availability and reliability of network services. The connectivity_plus plugin empowers developers with the ability to monitor and respond to connectivity changes, enabling them to build robust and adaptive applications irrespective of the user's network environment.
Understanding Connectivity Types
The connectivity_plus plugin utilizes platform-specific mechanisms to detect and categorize available connectivity types. These types are represented by the ConnectivityResult enum:
- wifi: Indicates a Wi-Fi connection.
- bluetooth: Bluetooth connectivity (Android and Linux only).
- ethernet: Ethernet connection (except for Web).
- mobile: Cellular network connection (except for Web).
- vpn: Virtual Private Network connection (Android, Windows, and Linux only).
- other: A network connection that doesn't fall into any of the above categories.
- none: No network connection is available.
The plugin supports determining the current connectivity status and subscribing to a stream of changes using the Connectivity class.
Checking Current Connectivity Status
import 'package:connectivity_plus/connectivity_plus.dart'; void main() async { var connectivityResult = await (Connectivity().checkConnectivity()); if (connectivityResult == ConnectivityResult.wifi) { // Wi-Fi is available } else if (connectivityResult == ConnectivityResult.mobile) { // Cellular network is available } else { // No connectivity } }
Subscribing to Connectivity Changes
import 'package:connectivity_plus/connectivity_plus.dart'; void main() { Connectivity().onConnectivityChanged.listen((ConnectivityResult result) { // Handle connectivity changes }); }
Platform Support
The connectivity_plus plugin offers widespread platform support, ensuring consistent functionality across various devices and operating systems:
Platform Wi-Fi Bluetooth Ethernet Mobile VPN Other
| Android | ✅ | ✅ | ✅ | ✅ | ✅ | ✅
| iOS | ✅ | ❌ | ✅ | ✅ | ❌ | ✅
| macOS | ✅ | ❌ | ✅ | ✅ | ❌ | ✅
| Web | ✅ | ❌ | ❌ | ❌ | ❌ | ✅
| Linux | ✅ | ✅ | ✅ | ❌ | ✅ | ✅
| Windows | ✅ | ❌ | ✅ | ❌ | ✅ | ✅
iOS and macOS Considerations
On iOS simulators, Wi-Fi connectivity updates may not be reflected accurately due to limitations. The onConnectivityChanged stream doesn't filter events or guarantee distinct values, which is essential to consider when working with these platforms.
Web Implementation
In browsers lacking the NetworkInformation Web API, the plugin falls back to the NavigatorOnLine API, providing a boolean connectivity status (online or offline). Due to privacy concerns, browsers do not disclose specific network information like SSID or MAC addresses.
Additional Resources
Conclusion
The connectivity_plus plugin is an indispensable tool for Flutter developers, empowering them to monitor and respond to network connectivity changes effectively. By leveraging platform-specific mechanisms, the plugin provides a consistent and reliable way to ensure optimal user experiences regardless of the network environment.